To allow Next.js to display images from all domains, modify your next.config.js
file to add an object with protocol
and hostname
properties to the remotePatterns
array property.
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
// other properties...
remotePatterns: [
{
protocol: 'https',
hostname: '**',
},
],
};
module.exports = nextConfig;
Why would I need to allow all domains for Next.js images?
Because you want to avoid this error:
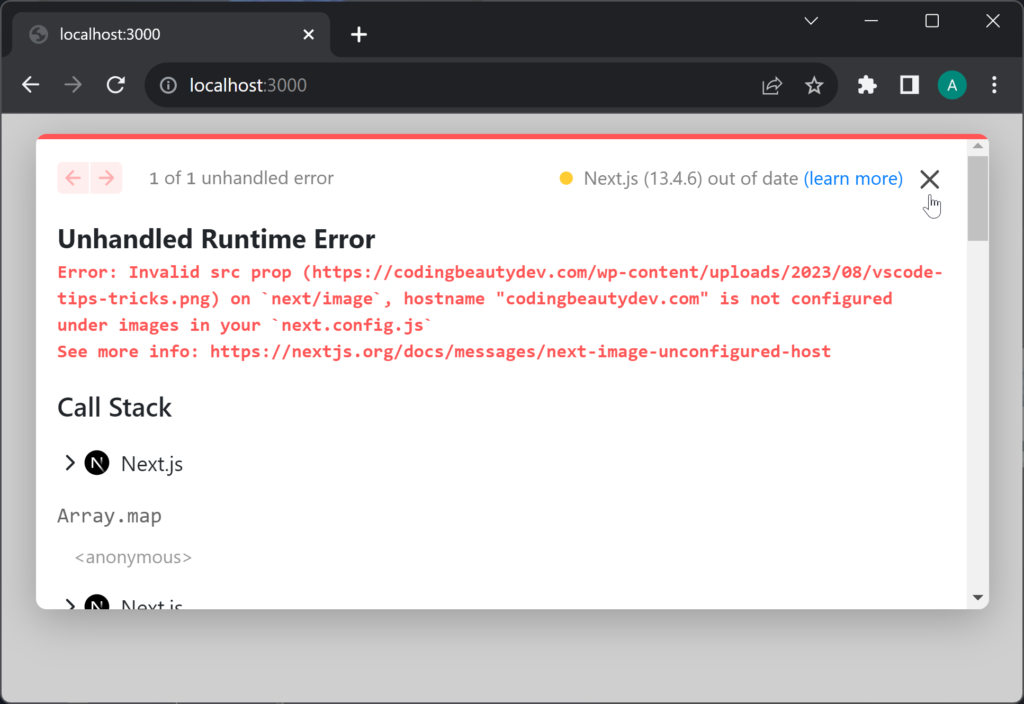
Which happens when you have next.config.js
with a limited number of specified domains, for example:
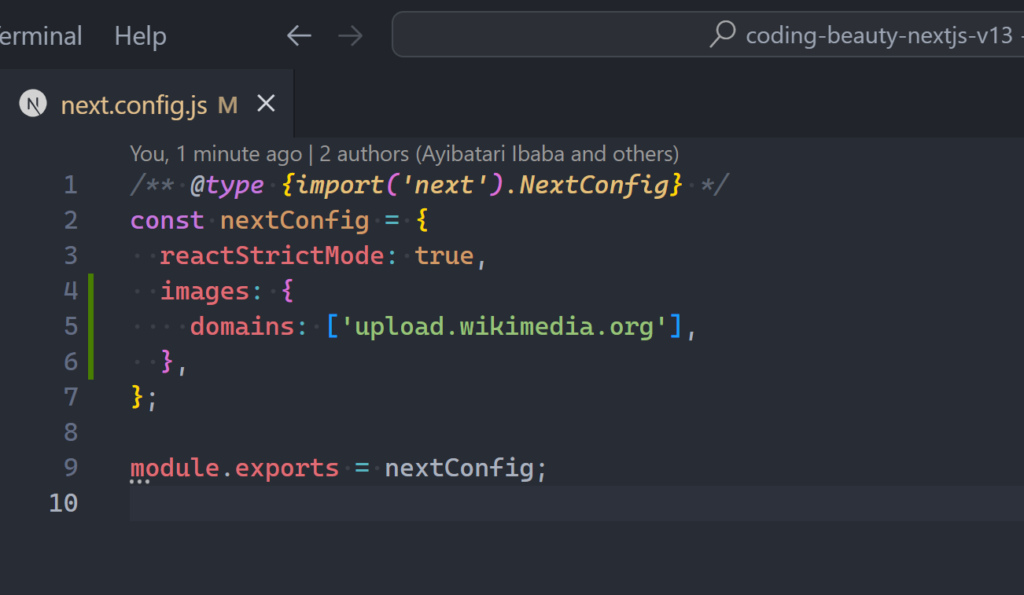
upload.wikimedia.org
are allowed in this Next.js app.But you try to display images from a domain not in the image.domains
list:
import React from 'react';
import { Metadata } from 'next';
import Image from 'next/image';
export const metadata: Metadata = {
title: 'Coding Beauty',
description: 'Tutorials by Coding Beauty',
};
export default function Page() {
return (
<main>
<Image
src="https://codingbeautydev.com/wp-content/uploads/2023/08/vscode-tips-tricks.png"
alt="Coding Beauty article featured image"
width={100}
height={100}
></Image>
10 essential VS Code tips & tricks for greater
productivity
</main>
);
}
remotePatterns
vs images.domains
property in next.config.js
In this case, you can follow the steps earlier in this article:
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
// other properties...
remotePatterns: [
{
protocol: 'https',
hostname: '**',
},
],
};
module.exports = nextConfig;
Or you can modify your next.config.js
to include codingbeautydev.com
:
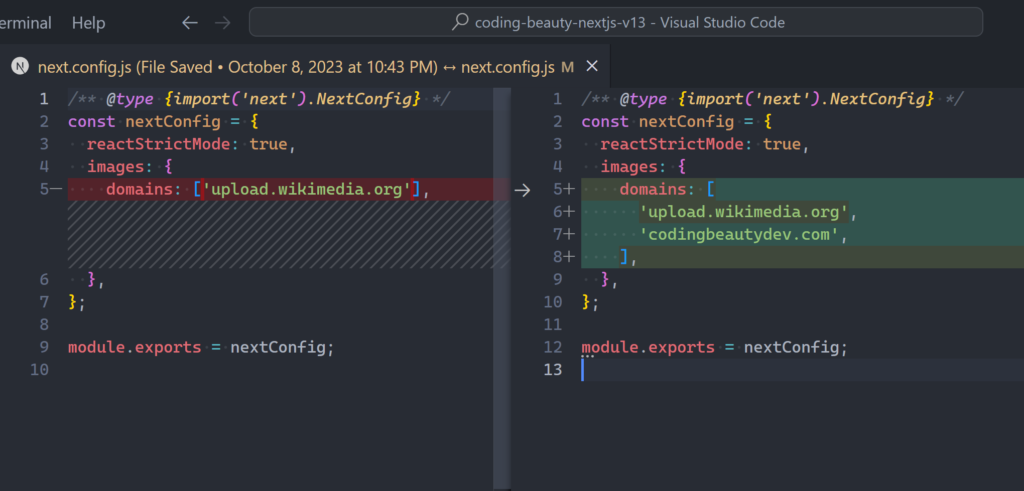
codingbeautydev.com
in this Next.js app.Of course, if you don’t have any domains
property, you just create one with the new domain as its single element.
While the image.domains
is simple and straightforward, the remotePatterns
property gives us more flexibility with its support for wildcard pattern matching, and protocol, port, and pathname restriction.
These advanced restrictions remotePatterns
provide greater security when you don’t own all the content in the domain, which is why the Next.js team recommends it.
Why do we need to be explicit with domains for Next.js images?
So whether you use remotePatterns
or images.domains
, you still need to be explicit about the domains that a next/image
component can display.
By doing so, you can prevent the potential security risks of loading images from random, unverified URLs that could contain malicious code.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
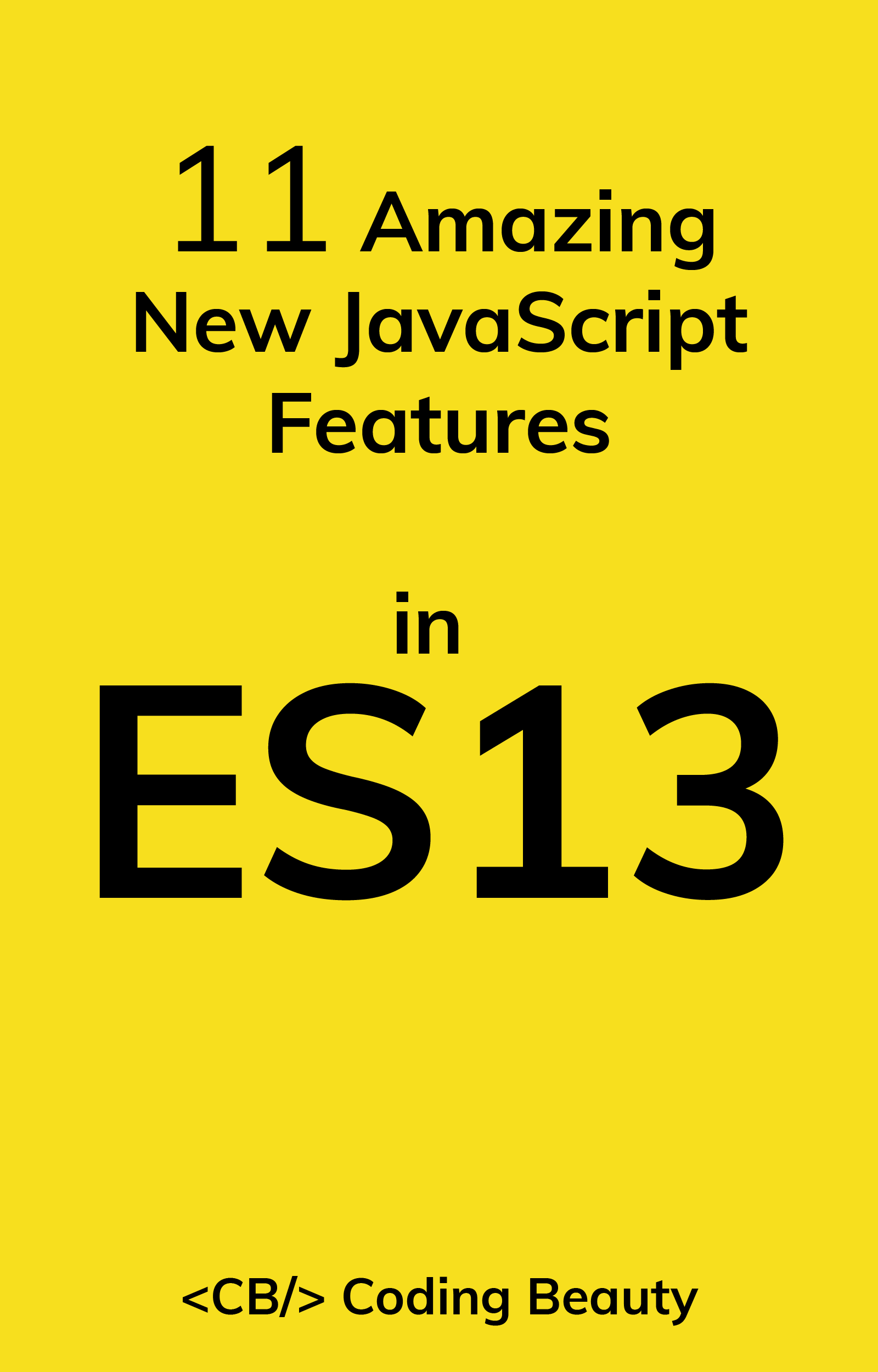