Let’s be honest: you probably don’t care about Sets! At least until now…
They’ve been here since ES6 but they’re usually relegated to making sure a list has no duplicates.
const array = [1, 4, 3, 2, 3, 1];
const noDuplicates = [...new Set(array)];
console.log(noDuplicates); // [1, 4, 3, 2]
With these 7 upcoming built-in Set methods, we could find ourselves using them a LOT more often.
1. union()
The new Set union()
method gives us all the unique items in both sets.
const creation = new Set(['coding', 'writing', 'painting']);
const joy = new Set(['crying', 'laughing', 'coding']);
console.log(creation.union(joy));
// Set { 'coding', 'crying', 'writing', 'laughing', 'painting' }
And since it’s immutable and returns a copy, you can chain it indefinitely:
const odd = new Set([21, 23, 25]);
const even = new Set([20, 22, 24]);
const prime = new Set([23, 29]);
console.log(odd.union(even).union(prime));
// Set(7) { 21, 23, 25, 20, 22, 24, 29 }
2. intersection()
What elements are in both sets?
const mobile = new Set(['javascript', 'java', 'swift', 'dart']);
const backend = new Set(['php', 'python', 'javascript', 'java']);
const frontend = new Set(['javascript', 'dart']);
console.log(mobile.intersection(backend));
// Set { javascript, java }
console.log(mobile.intersection(backend).intersection(frontend));
// Set { javascript }
3. difference()
difference()
does A – B to return all the elements in A that are not in B:
const joy = new Set(['crying', 'laughing', 'coding']);
const pain = new Set(['crying', 'screaming', 'coding']);
console.log(joy.difference(pain));
// Set { 'laughing' }
4. symmetricDifference()
As symmetric implies, this method gets the set difference both ways. That’s (A – B) U (B – A).
All the items in 1 and only 1 of the sets:
const joy = new Set(['crying', 'laughing', 'coding']);
const pain = new Set(['crying', 'screaming', 'coding']);
console.log(joy.symmetricDifference(pain));
// Set { 'laughing', 'screaming' }
5. isSubsetOf()
Purpose is clear: check if all elements of a set are in another set.
const colors = new Set(['indigo', 'teal', 'cyan', 'violet']);
const purpleish = new Set(['indigo', 'violet']);
const secondary = new Set(['orange', 'green', 'violet']);
console.log(purpleish.isSubsetOf(colors)); // true
console.log(secondary.isSubsetOf(colors)); // false
console.log(colors.isSubsetOf(colors)); // true
6. isSupersetOf()
Check if one set contains all the elements in another set: As good as swapping the two sets in isSubsetOf()
:
const colors = new Set(['salmon', 'cyan', 'yellow', 'aqua']);
const blueish = new Set(['cyan', 'aqua']);
const primary = new Set(['red', 'yellow', 'blue']);
console.log(colors.isSupersetOf(blueish)); // true
console.log(colors.isSupersetOf(primary)); // false
console.log(colors.isSupersetOf(colors)); // true
7. isDisjointFrom()
isDisjointFrom
: Do these sets share zero common elements?
const ai = new Set(['python', 'c++']);
const mobile = new Set(['java', 'js', 'dart', 'kotlin']);
const frontend = new Set(['js', 'dart']);
console.log(ai.isDisjointFrom(mobile)); // true
console.log(mobile.isDisjointFrom(frontend)); // false
Use them now
With core-js
polyfills:
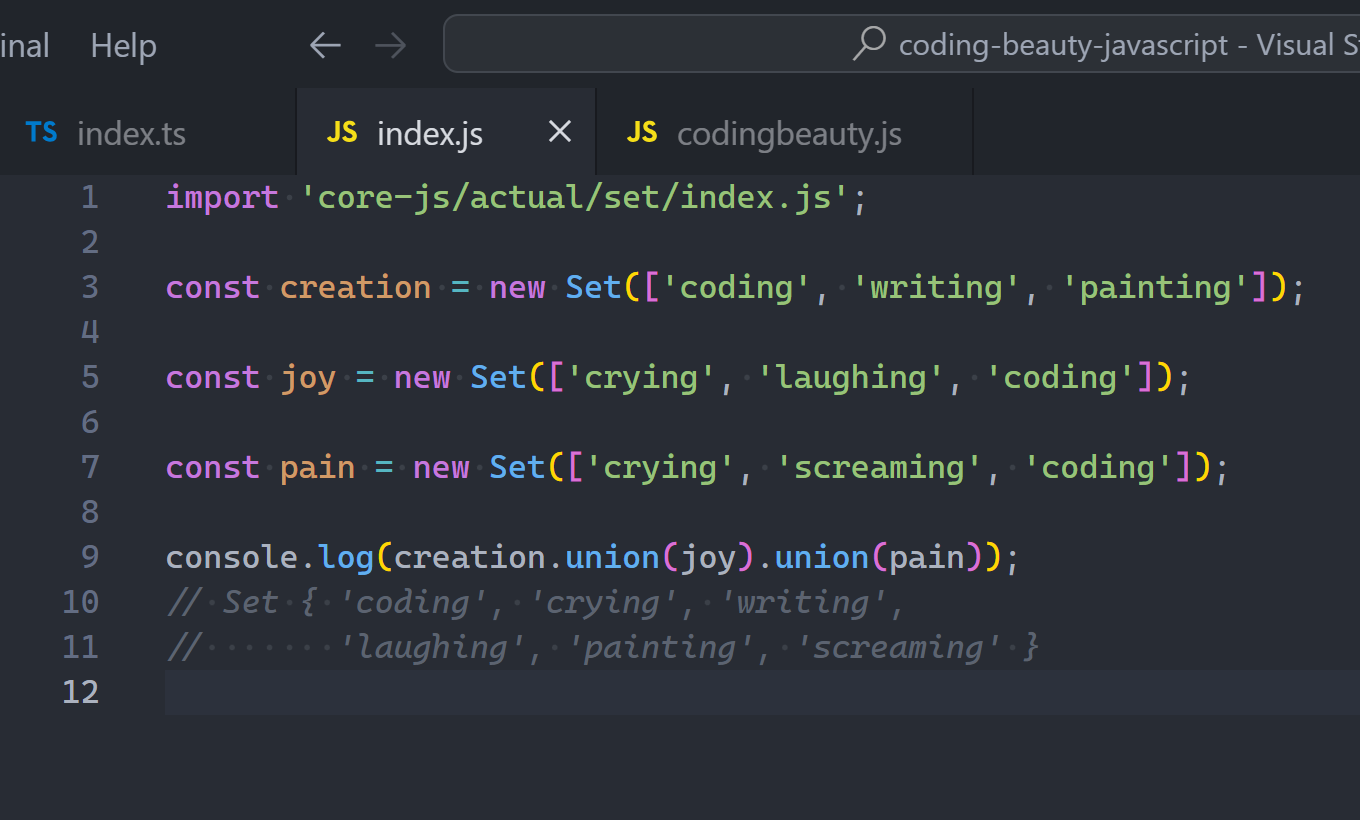
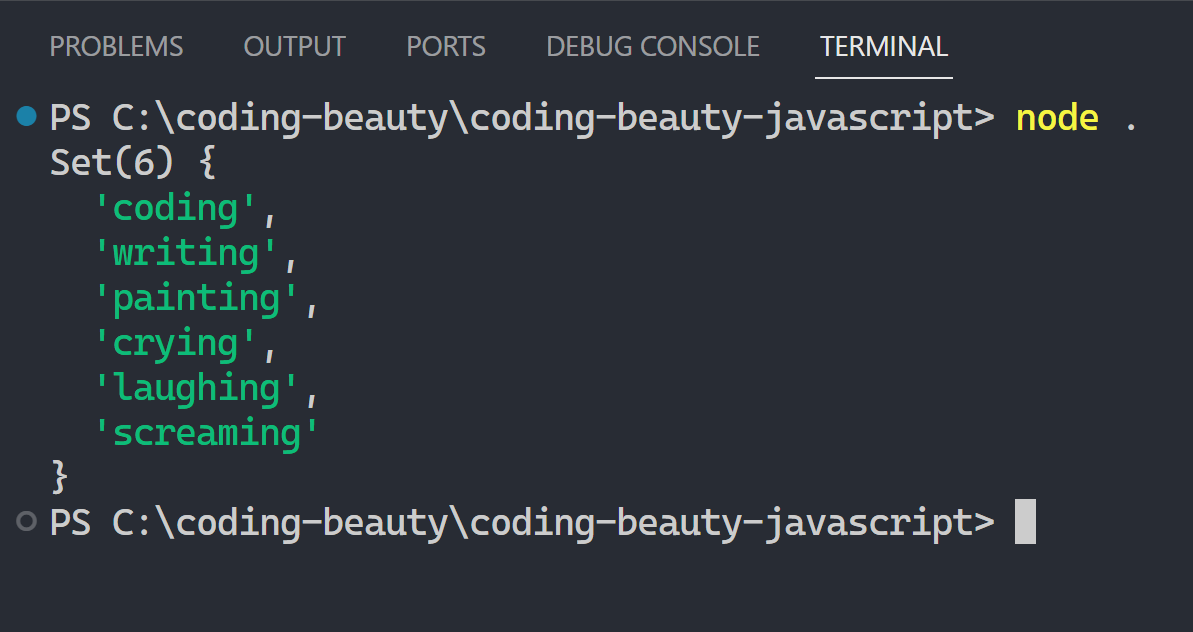
Otherwise you get blasted with errors from TypeScript & Node.js — they’re not yet in the official JavaScript standard.
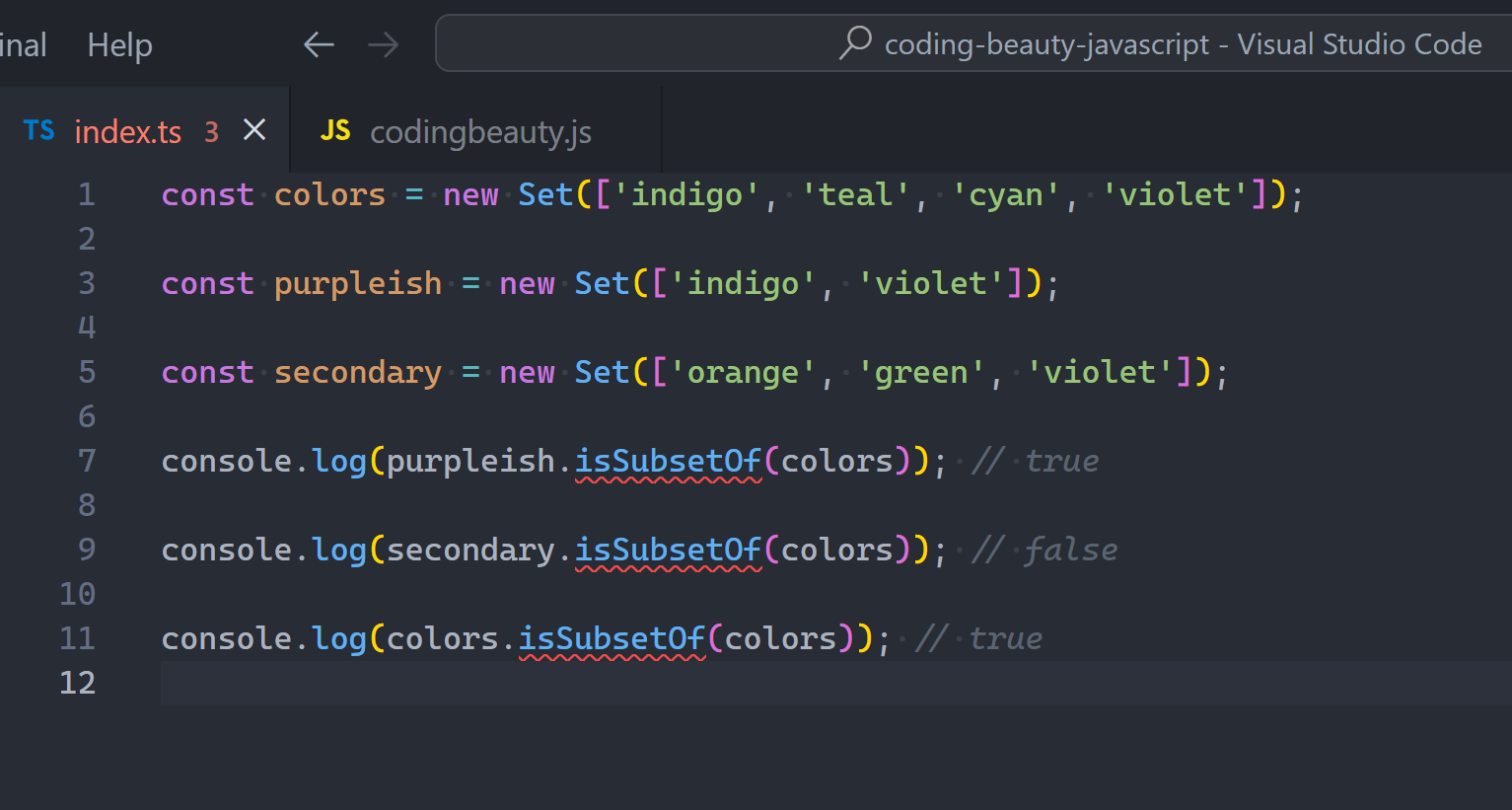
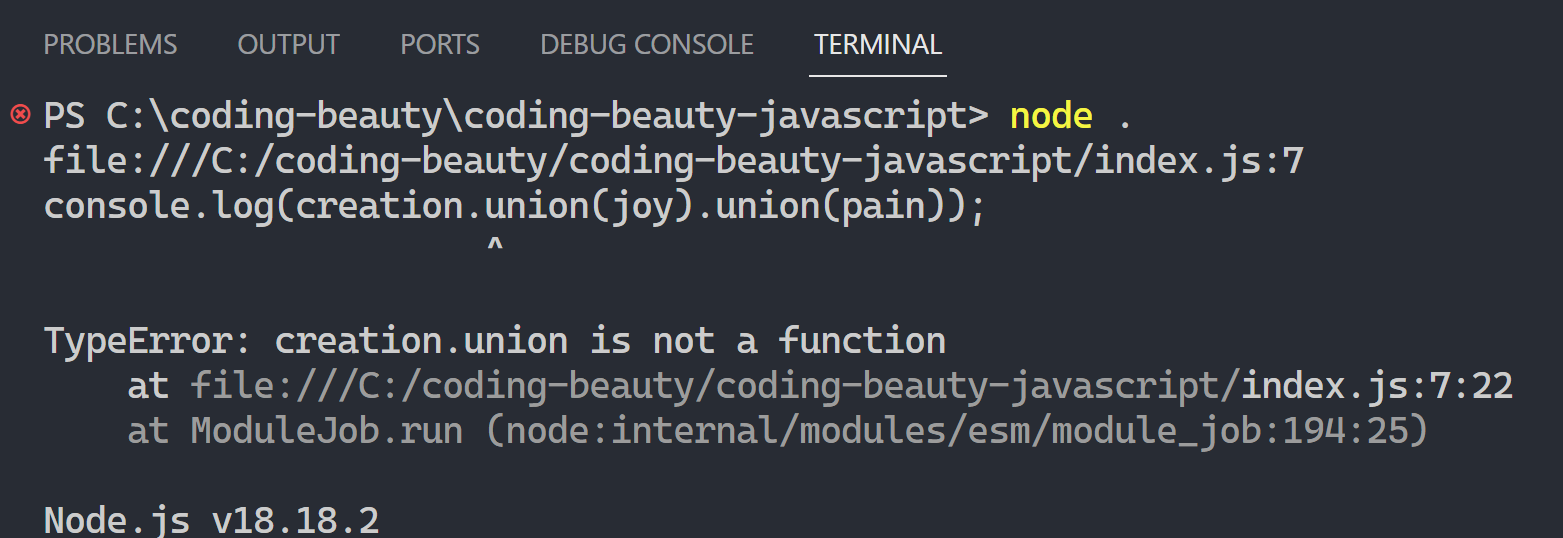
Wrap up
So these are our 7 new Set methods — no more need for 3rd parties like _.intersection()
(Lodash!)
const unique = new Set(['salmon', 'cyan', 'cherry', 'aqua']);
const blueish = new Set(['cyan', 'aqua', 'blue']);
const primary = new Set(['red', 'green', 'blue']);
console.log(unique.union(blueish)); // Set { 'salmon', 'cyan', 'cherry', 'aqua', 'blue' }
console.log(unique.intersection(blueish)); // Set { 'cyan', 'aqua' }
console.log(unique.difference(blueish)); // Set { 'salmon', 'cherry' }
console.log(unique.symmetricDifference(blueish)); // Set { 'salmon', 'cherry', 'blue' }
console.log(primary.isSubsetOf(unique)); // false
console.log(new Set(['red', 'green']).isSubsetOf(primary)); // true
console.log(unique.isSupersetOf(new Set(['salmon', 'aqua']))); // true
console.log(unique.isSupersetOf(blueish)); // false
console.log(unique.isDisjointFrom(primary)); // true
console.log(unique.isDisjointFrom(blueish)); // false
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
