In JavaScript, working with the Document Object Model (DOM) often involves iterating through child elements of a parent element. This technique is essential for tasks such as:
- Manipulating elements based on their content or attributes
- Dynamically adding or removing elements
- Handling events for multiple elements
JavaScript offers several methods to achieve this, each with its own advantages and considerations.
Methods for looping
1. Use children
property
- Access the
children
property of the parent element to obtain a liveNodeList
of its direct child elements. - Iterate through the
NodeList
using afor
loop or other methods:
const parent = document.getElementById("myParent");
const children = parent.children;
for (let i = 0; i < children.length; i++) {
const child = children[i];
// Perform actions on the child element
console.log(child.textContent);
}
2. Use for..of
loop
Directly iterate over the NodeList
using the for...of
loop:
const parent = document.getElementById("myParent");
for (const child of parent.children) {
// Perform actions on the child element
console.log(child.tagName);
}
3. Use Array.from()
method
Convert the NodeList
into an array using Array.from()
, allowing the use of array methods like forEach()
:
const parent = document.getElementById("myParent");
const childrenArray = Array.from(parent.children);
childrenArray.forEach(child => {
// Perform actions on the child element
child.style.color = "red";
});
Key considerations
- Live
NodeList
: Thechildren
property returns a liveNodeList
, meaning changes to the DOM are reflected in theNodeList
. - Text Nodes: The
children
property includes text nodes, whilechildNodes
includes all types of nodes (elements, text, comments, etc.). Choose the appropriate property based on your needs. - Performance: For large DOM trees, using
Array.from()
might have a slight performance overhead due to array creation.
Choosing the right method
- For simple iterations, the
for...of
loop or thechildren
property with afor
loop are often sufficient. - If you need to use array methods or want to create a static copy of the child elements, use
Array.from()
. - Consider performance implications if dealing with large DOM structures.
By understanding these methods and their nuances, you’ll be able to effectively loop through child elements in JavaScript for various DOM manipulation tasks.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
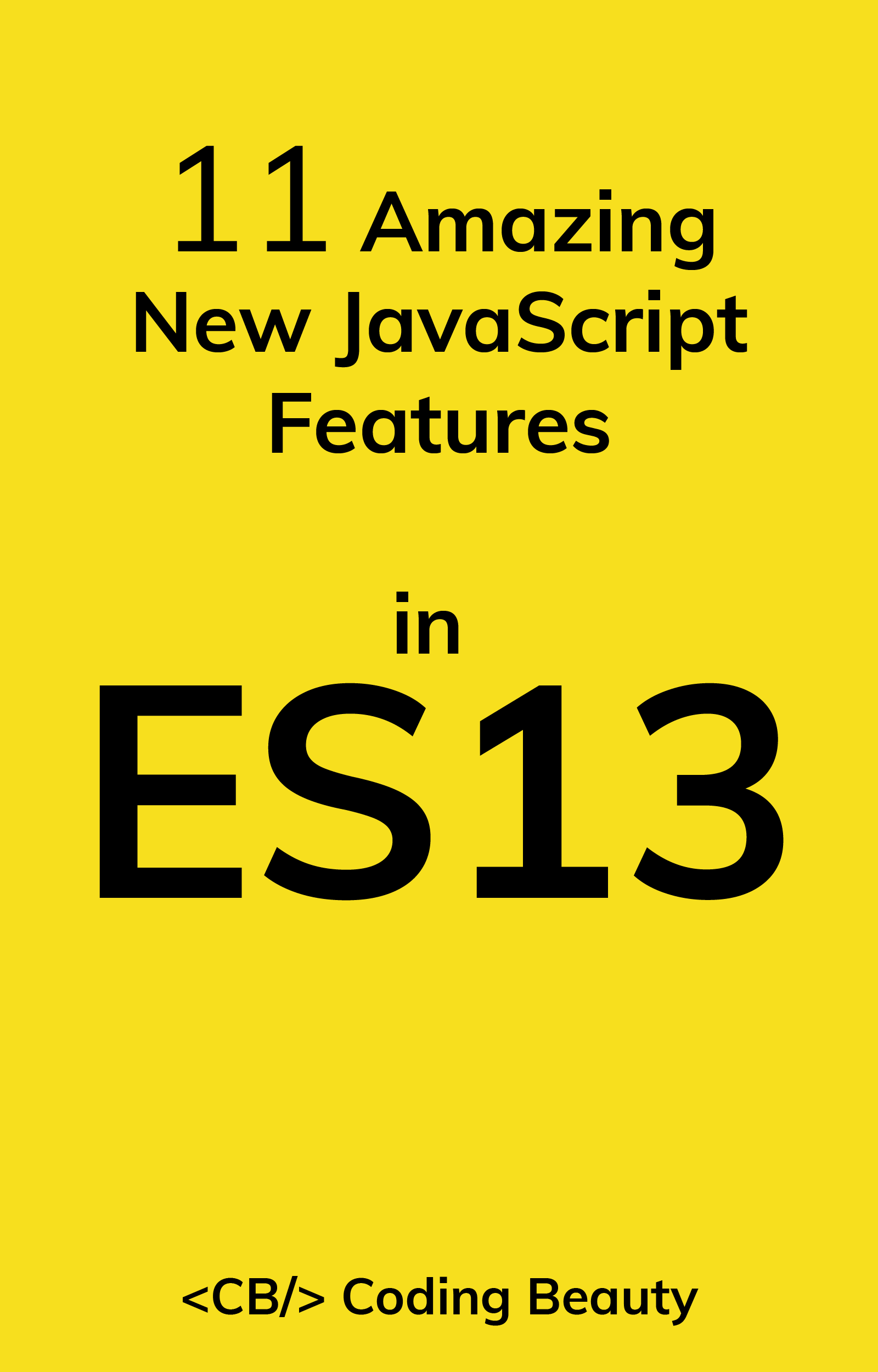