There’s more to console methods than log
error
and warn
.
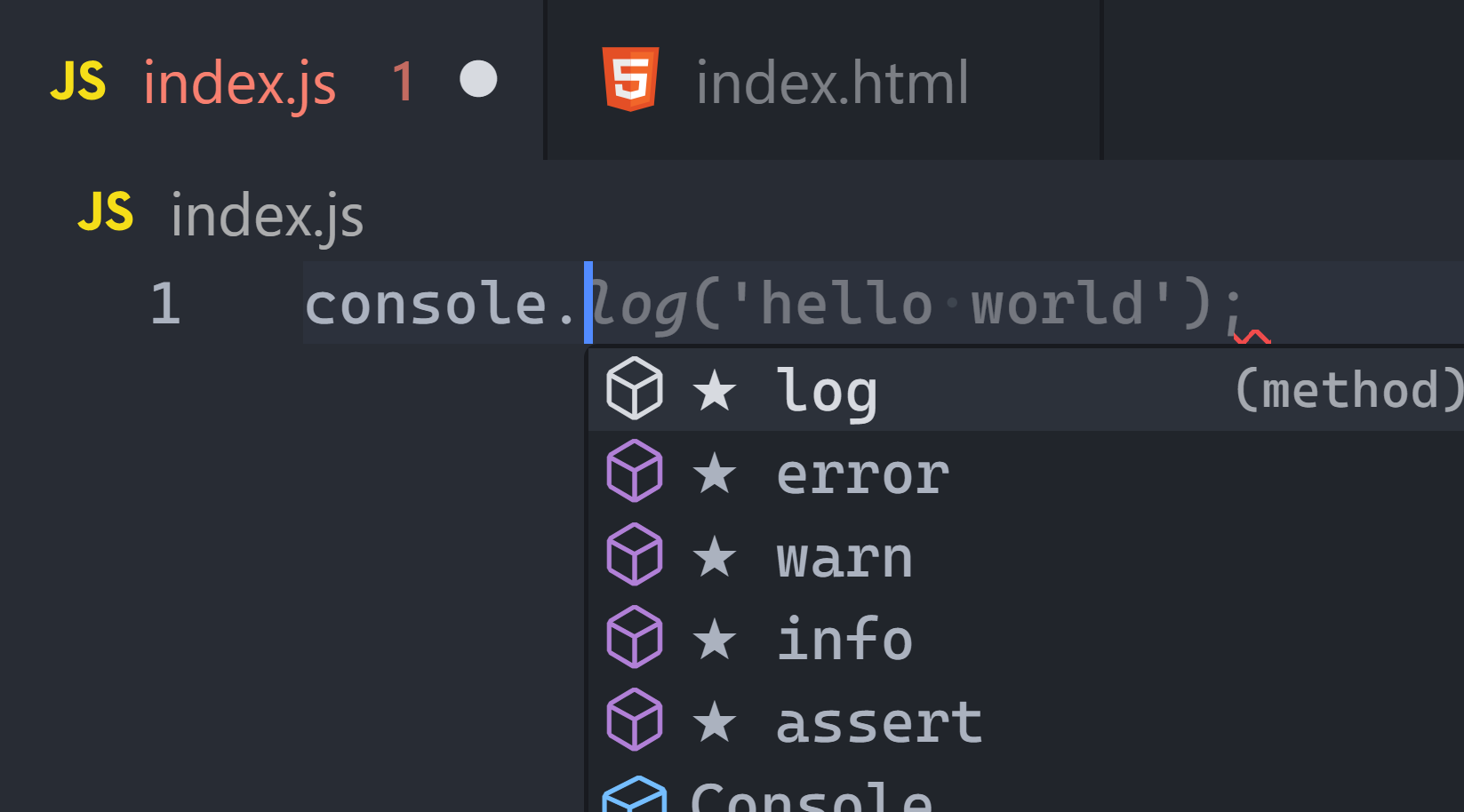
Did you know they’re actually up to 20?
And they do way more than logging text: colorful🎨 data visualization, debugging🔎, performance⚡ testing and so much more.
Check out these powerful 7:
1. table()
console.table()
: Easily display object array as table: one row per object; one col per prop.
const fruits = [
{
name: 'Mango',
ripeness: 10,
sweetness: '😋',
},
{
name: 'Banana',
ripeness: 6,
sweetness: '😏',
},
{
name: 'Watermelon',
ripeness: 8,
sweetness: '🤔',
},
];
console.table(fruits);
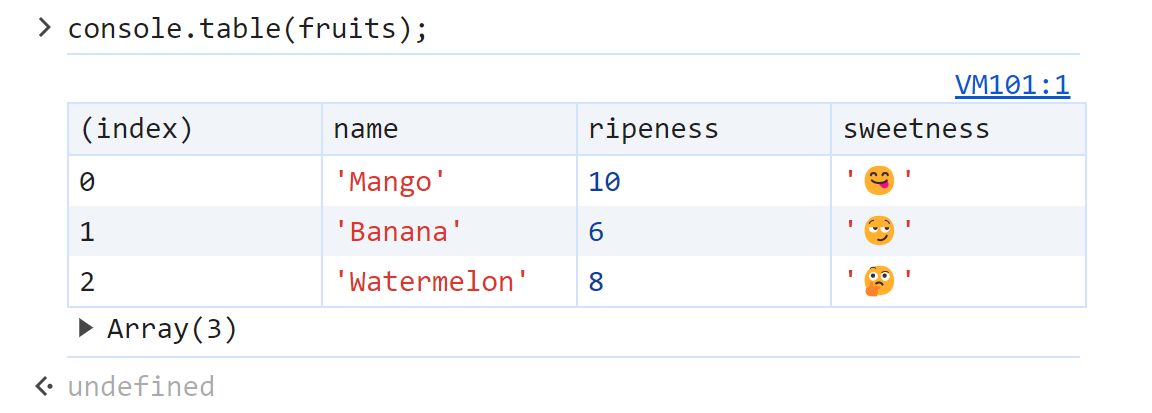
A bit different on Node:
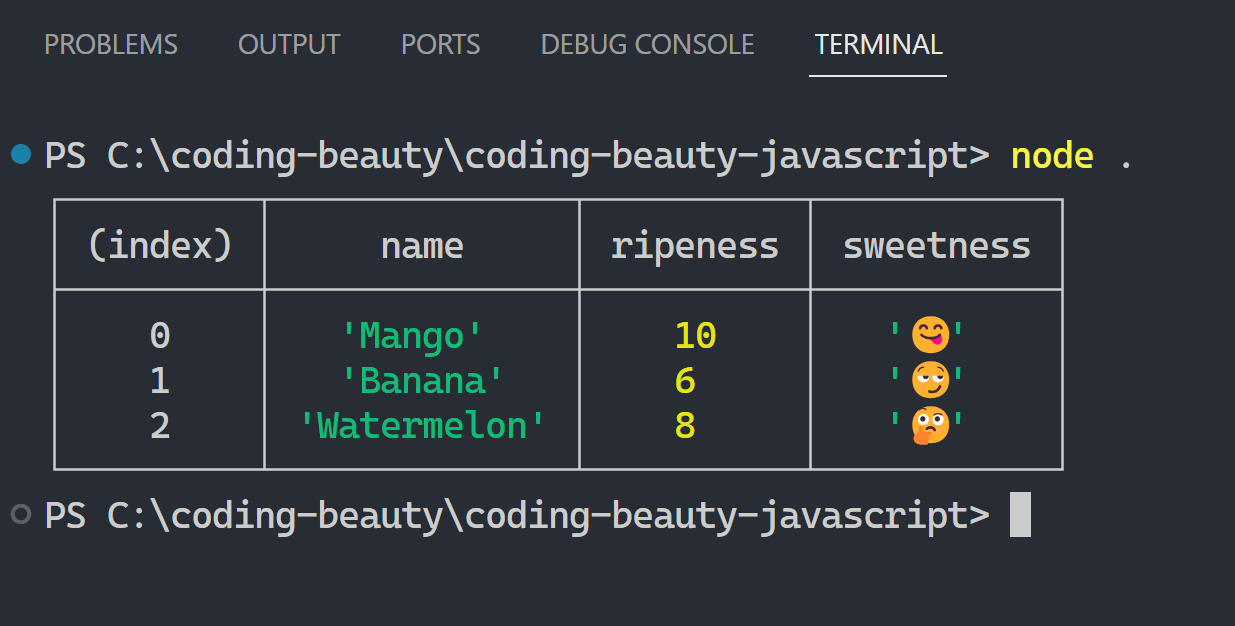
Both clearly better than using console.log()
:
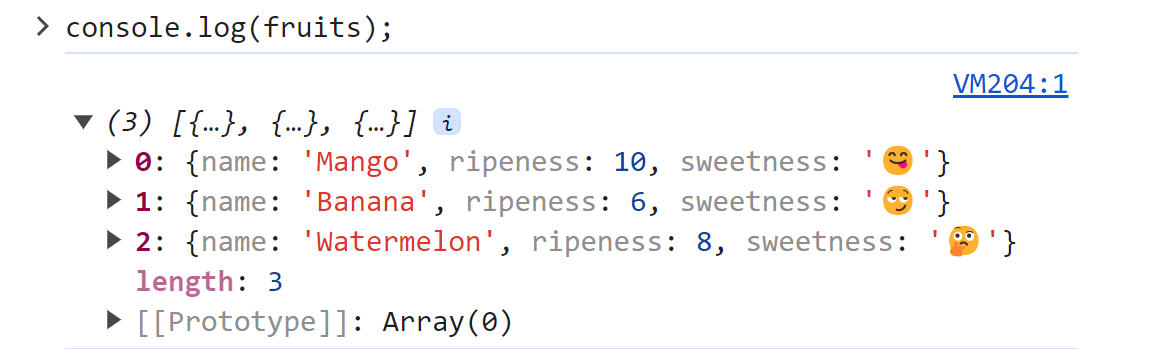
2. trace()
Where did we come from on the call stack? Let’s trace()
our steps back!
Fantastic for debugging:
function coder() {
goodCoder();
}
function goodCoder() {
greatCoder();
}
function greatCoder() {
greatSuperMegaGeniusCoder();
}
function greatSuperMegaGeniusCoder() {
console.log(
"You only see the greatness now, but it wasn't always like this!"
);
console.log(
'We\'ve been coding for generations -- let me show you...'
);
console.trace();
}
coder();
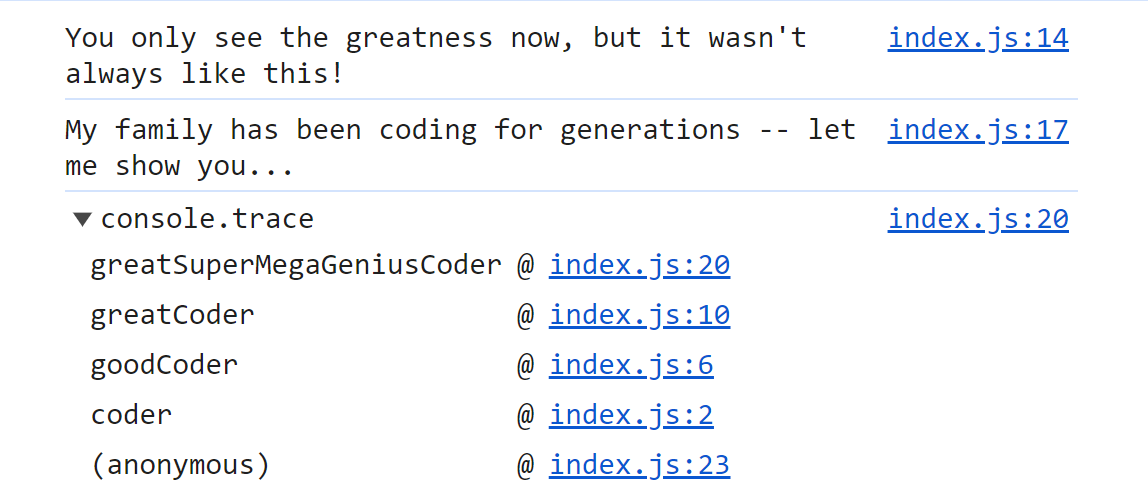
3. count()
console.count()
logs the number of times that the current call tocount()
has been executed.
Too indirect and recursive a definition for me! You may need to see an example to really get it…
function shout(message) {
console.count();
return message.toUpperCase() + '!!!';
}
shout('hey');
shout('hi');
shout('hello');
console.count() has an internal counter starting at 0. After each call it increments the counter by 1 and logs it…
Much better.
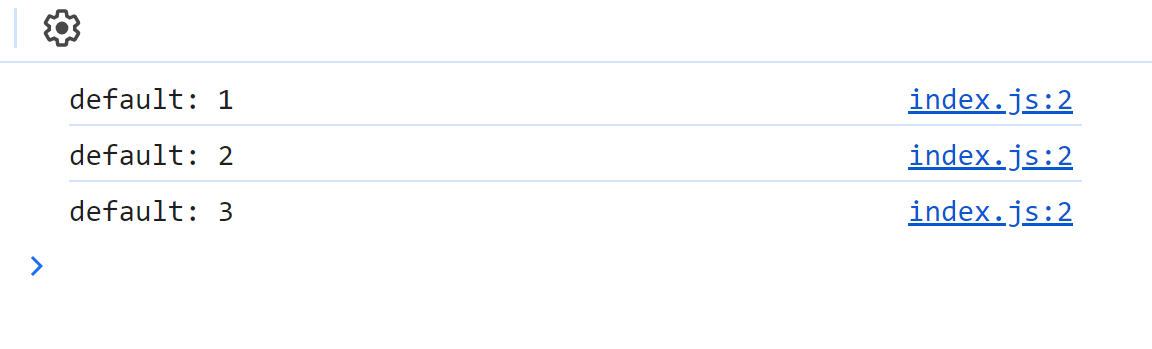
Where did default
come from? That’s the label for the counter. I’m guessing there’s an internal dictionary with a counter value for each label key.
console.count() has an internal counter starting at 0 for a new label. After each call it increments the counter by 1 and logs it…
We easily customize the label with the 1st argument to count()
.
function shout(message) {
console.count(message);
return message.toUpperCase() + '!!!';
}
shout('hey');
shout('hi');
shout('hello');
shout('hi');
shout('hi');
shout('hello');
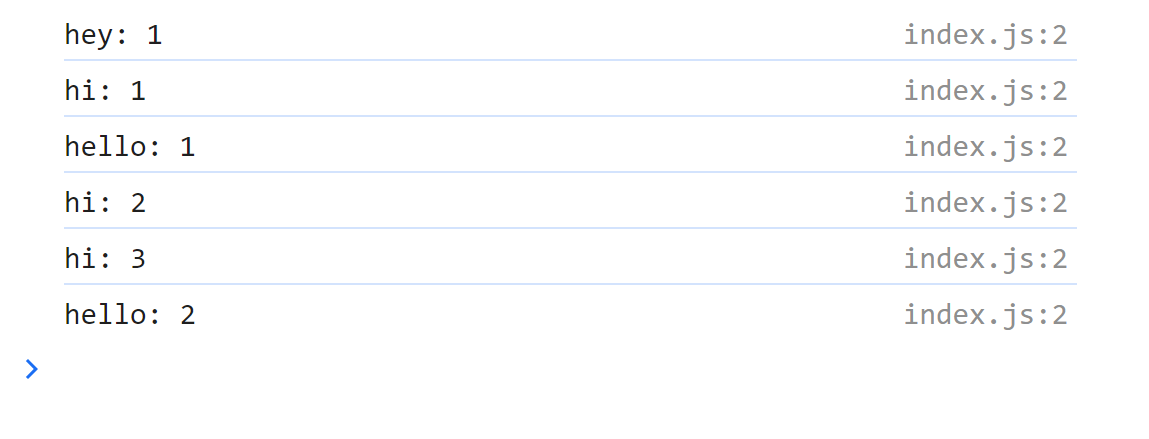
Now we have a different count for each message.
So countReset()
obviously resets a label’s internal counter to 0.
function shout(message) {
console.count(message);
return message.toUpperCase() + '!!!';
}
shout('hi');
shout('hello');
shout('hi');
shout('hi');
shout('hello');
console.log('resetting hi');
console.countReset('hi');
shout('hi');
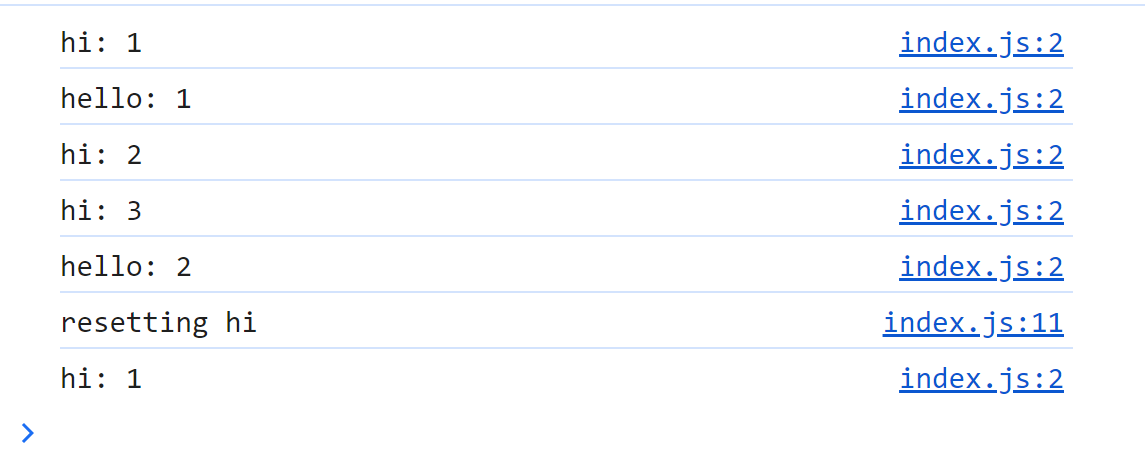
4. clear()
CLS for JavaScript.
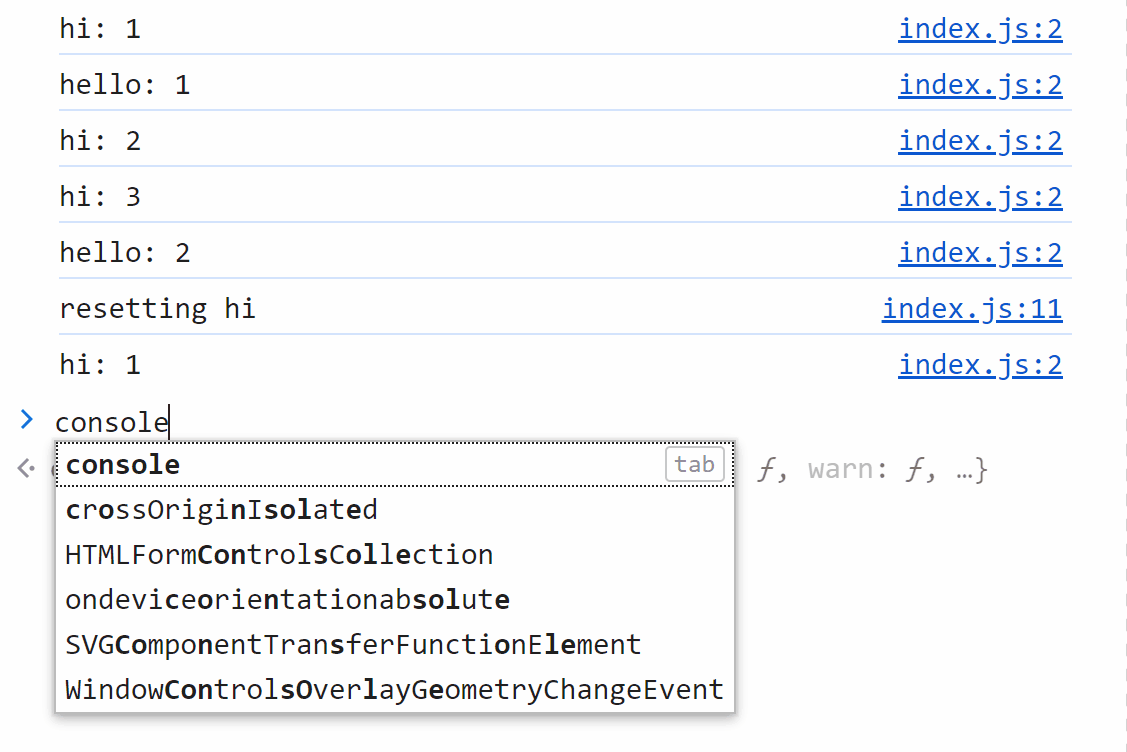
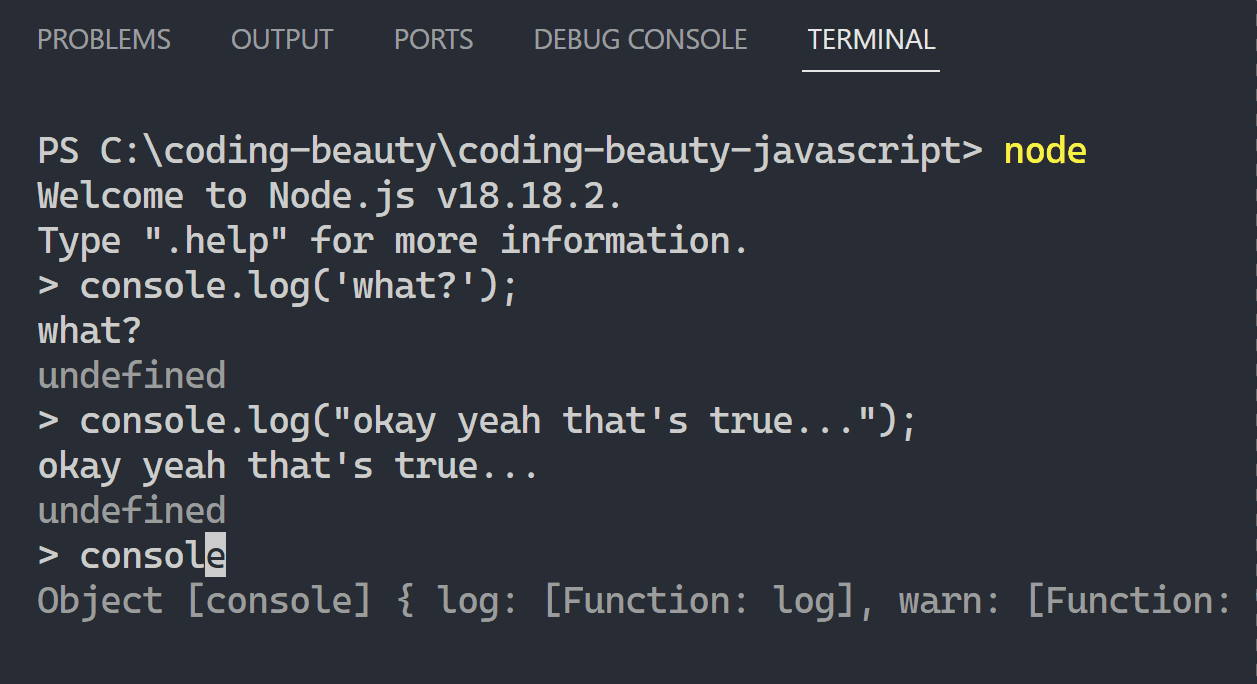
5. time()
+ timeLog()
+ timeEnd()
They work together to precisely measure how long a task takes.
57 microseconds, 11 seconds, 50 years? No problem.
time()
– start the timer.timeLog()
– how far has it gone?timeEnd()
– stop the timer.
Let’s use them to compare the speed of all the famous JavaScript loop types.
console.time('for');
for (let i; i < 1000; i++) {
for (let i = 0; i < arr.length; i++);
}
console.timeLog('for');
for (let i; i < 1000000; i++) {
for (let i = 0; i < arr.length; i++);
}
console.timeEnd('for');
const arr1000 = [...Array(1000)];
const arr1000000 = [...Array(1000000)];
console.time('for of');
for (const item of arr1000);
console.timeLog('for of');
for (const item of arr1000000);
console.timeEnd('for of');
console.time('forEach');
arr1000.forEach(() => {});
console.timeLog('forEach');
arr1000000.forEach(() => {});
console.timeEnd('forEach');
for
starts out slow but it destroys the other as the list grows…
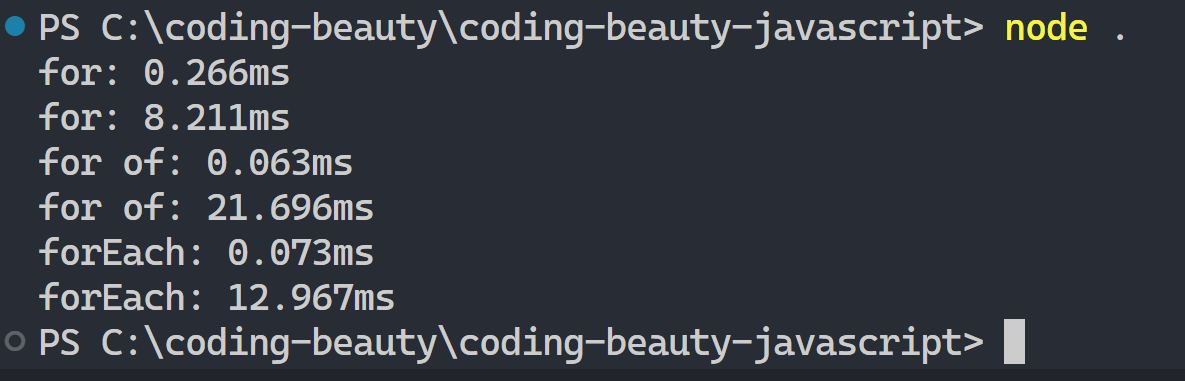
6. group()
+ groupCollapsed()
+ groupEnd()
Another great combo for grouping a bunch of console messages together; visually with indentation and functionally with a UI expander.
group()
– adds 1 further grouping level.groupCollapsed()
– likegroup()
but group starts out collapsed.groupEnd()
– go back to previous grouping level.
console.log('What can we do?');
console.group('Abilities');
console.log('Run👟 - solid HIIT stuff');
console.groupCollapsed('Code💻');
console.log('JavaScript');
console.log('Python');
console.log('etc of course');
console.groupEnd();
console.log('Eat🍉 - not junk tho...');
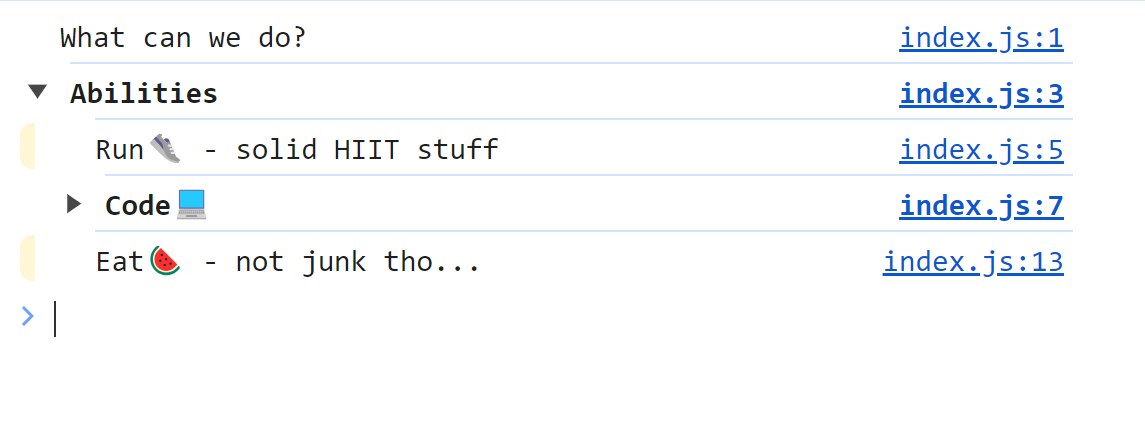
Just indentation on Node – so groupCollapsed()
has no use here.
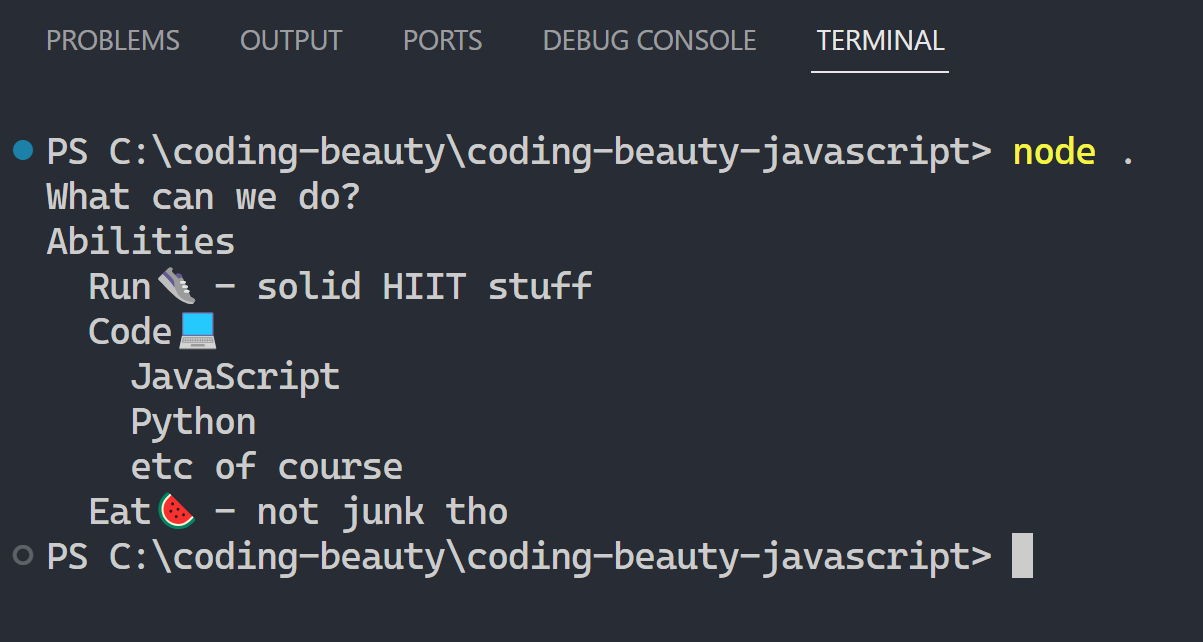
7. dir()
dir()
is a genius way to inspect an object in the console and see ALL it’s properties and methods.
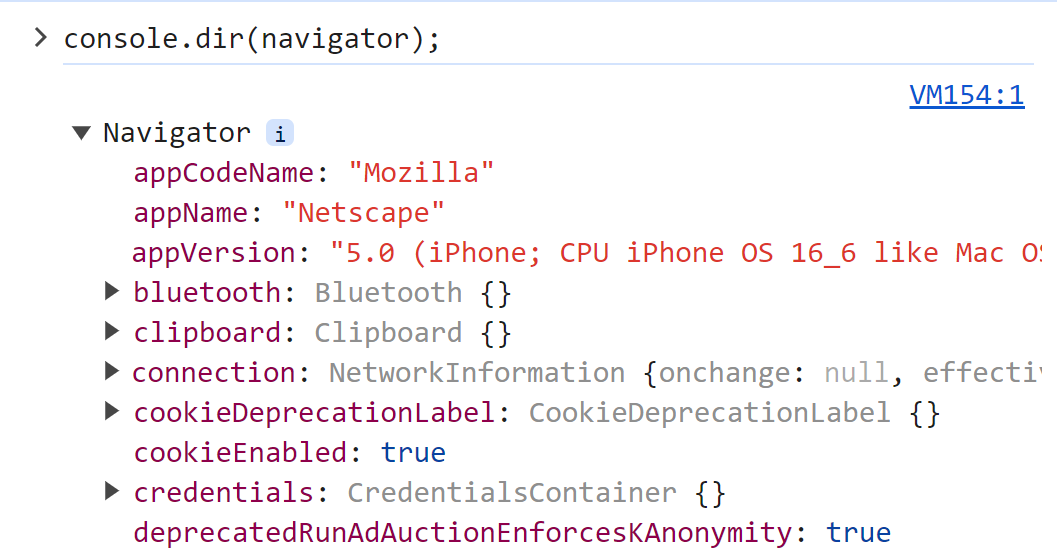
Actually just like console.log()
? 🤔 But console.dir()
is specially designed for this particular purpose.
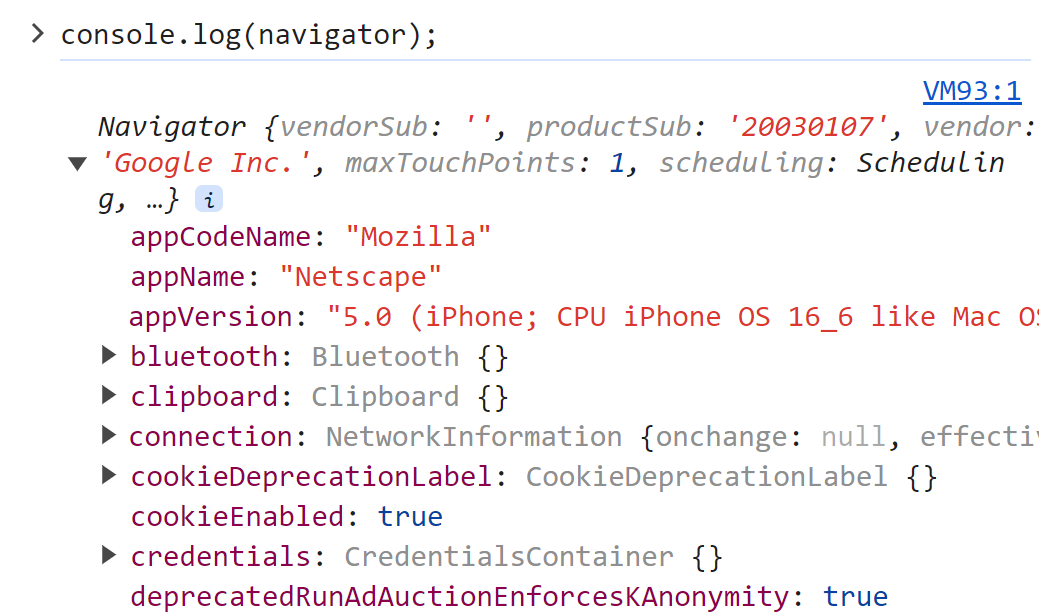
But watch what happens when you log()
vs dir()
HTML element objects:
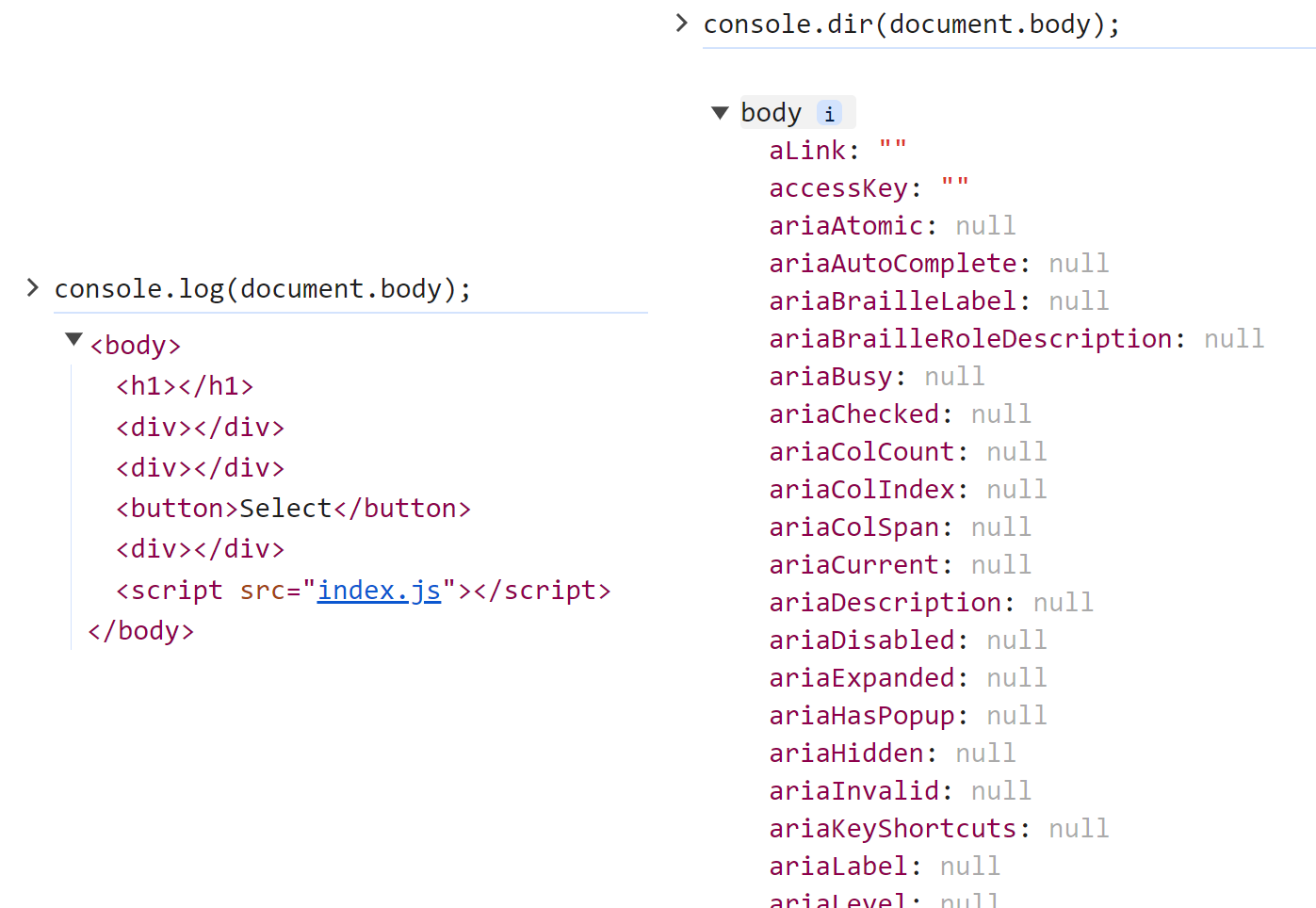
log()
shows it as a HTML tag hierarchy, but dir()
shows it as an object with every single property it has and could ever dream of having.
Final thoughts
So they’re plenty of console methods apart from console.log()
. Some of them spice things up in the console UI with better visualization; others are formidable tools for debugging and performance testing.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
