To prevent a page refresh on form submit in Vue.js, use the .prevent
event modifier in the submit event listener.
<template>
<div id="app">
<form @submit.prevent="handleSubmit">
<label>
Email
<br />
<input
type="email"
v-model="email"
required
/>
</label>
<br />
<label>
Password
<br />
<input
type="password"
v-model="password"
required
/>
</label>
<br />
<button
type="submit"
style="margin-top: 16px"
>
Submit
</button>
</form>
</div>
</template>
<script>
export default {
name: 'App',
data() {
return {
email: '',
password: '',
};
},
methods: {
handleSubmit() {
this.email = '';
this.password = '';
alert('Form submitted');
},
},
};
</script>
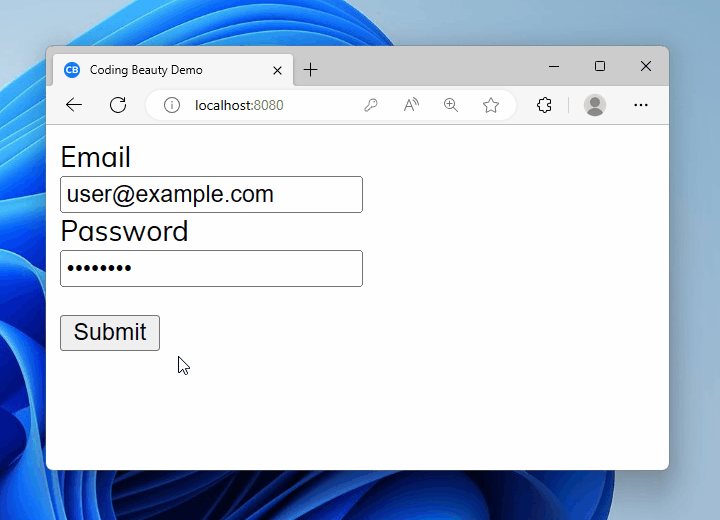
We use the .prevent
modifier to stop the default action of the event from happening. In this case, that action was a page refresh, so .prevent
stopped the page refresh on the form submission.
<form @submit.prevent="handleSubmit">
.prevent
is a Vue.js event modifier, which lets us access and modify event-related data.
We use the @submit
directive to add a submit
event listener to the form; this listener runs when the user clicks the button to submit the form.
In this case we only reset the form; in practical scenarios you’ll likely make an AJAX request to a server with the form data.
<template>
...
</template>
<script>
export default {
...
methods: {
async handleSubmit() {
const user = {
email: this.email,
password: this.password,
};
try {
const response = await axios.post('http://your-api-url.com', user);
console.log(response.data);
} catch (error) {
console.error(error);
}
this.email = '';
this.password = '';
}
}
};
</script>
For the button to submit the form, we must set its type
attribute to "submit"
<button type="submit" style="margin-top: 16px;">
Submit
</button>
With type="submit"
, the browser also submits the form when the user presses the Enter key in an input field.
Remove type="submit"
from the button to prevent page refresh
To prevent page refresh on form submit in Vue.js, you can also remove the type="submit"
from the button.
When is this useful? You may want to show an alert message or something before submission. In this case, we wouldn’t want type="submit"
since it submits the form on button click.
<template>
<div id="app">
<form>
<label>
Email
<br />
<input
type="email"
v-model="email"
required
/>
</label>
<br />
<label>
Password
<br />
<input
type="password"
v-model="password"
required
/>
</label>
<br />
<button
type="button"
@click="handleSubmit"
style="margin-top: 16px"
>
Submit
</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
email: '',
password: '',
};
},
methods: {
handleSubmit() {
// Perform custom actions before form submission
alert('Are you sure you want to submit the form?');
// Simulate form submission by clearing input fields
this.email = '';
this.password = '';
alert('Form submitted');
},
},
};
</script>
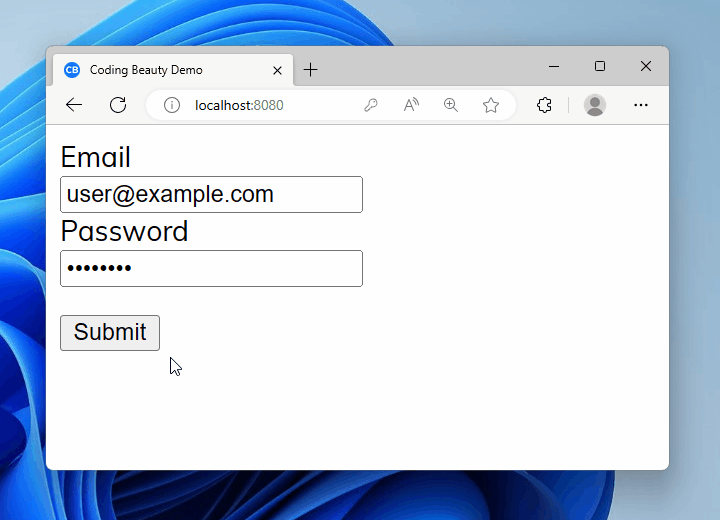
We used type="button"
on the button, but that’s as good as not having a type
attribute. It doesn’t do anything on click.
Key takeaways
- To prevent page refresh on form submit in Vue.js, use the
.prevent
modifier in the submit event listener. - Set
type="submit"
to ensure the form submits on button click or Enter press. Remove it when you don’t want this. - Remove
type="submit"
when something needs to happen after the button click before submitting the form, e.g., show a dialog, make an initial request, etc.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
