To open a link in a new tab programmatically in React, call the window.open()
method with the link as an argument, e.g., window.open('https://codingbeautydev.com')
. The open()
method will open the link in a new browser tab.
In the following example, we programmatically open the link in a new tab at the end of a countdown:
App.js
import { useRef, useEffect, useState } from 'react';
export default function App() {
const [timeLeft, setTimeLeft] = useState(3);
const interval = useRef();
useEffect(() => {
interval.current = setInterval(() => {
// Decrease "timeLeft" by 1 every second
setTimeLeft((prev) => prev - 1);
}, 1000);
return () => clearInterval(interval.current);
}, []);
useEffect(() => {
// Open the link in a new tab when the countdown ends
if (timeLeft === 0) {
clearInterval(interval.current);
// 👇 Open link in new tab programmatically
window.open('https://codingbeautydev.com', '_blank', 'noreferrer');
}
}, [timeLeft]);
return (
<div>
The link will open in <b>{timeLeft}</b> second(s)
</div>
);
}
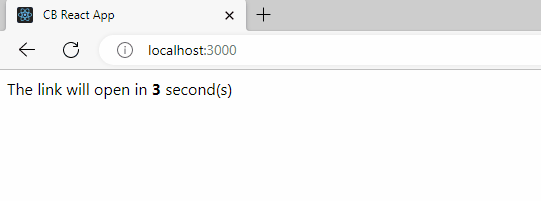
We use the open() method of the window
object to programmatically open a link in a new tab. This method has three optional parameters:
url
: The URL of the page to open in a new tab.target
: like thetarget
attribute of the<a>
element, this parameter’s value specifies where to open the linked document, i.e., the browsing context. It accepts all the values thetarget
attribute of the<a>
element accepts.windowFeatures
: A comma-separated list of feature options for the window.noreferrer
is one of these options.
Passing _blank
to the target
parameter makes the link get opened in a new tab.
Open link in new tab on button click
Being able to open a link in a new tab programmatically means that we can use a button in place of an anchor link to open a URL on click. We’d just set an onClick
event listener on the button element and call the window.open()
method in the listener.
For example:
App.js
export default function App() {
const openInNewTab = (url) => {
window.open(url, '_blank', 'noreferrer');
};
return (
<div>
<p>Visit codingbeautydev.com in a new tab</p>
<button
role="link"
onClick={() => openInNewTab('https://codingbeautydev.com')}
>
Visit
</button>
</div>
);
}
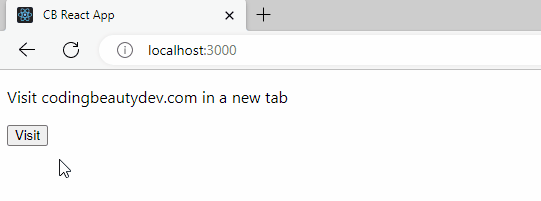
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
