To open a link in a new tab in Vue, create an anchor (<a>
) element and set its target
attribute to _blank
, e.g., <a href="https://codingbeautydev.com" target="_blank"></a>.
The _blank
value specifies that the link should be opened in a new tab.
App.vue
<template>
<div id="app">
<a
href="https://codingbeautydev.com"
target="_blank"
rel="noreferrer"
>
Coding Beauty
</a>
<br /><br />
<a
href="https://codingbeautydev.com/blog"
target="_blank"
rel="noreferrer"
>
Coding Beauty Blog
</a>
</div>
</template>
The target
property of the anchor element specifies where to open the linked document. By default target
has a value of _self
, which makes the linked page open in the same frame or tab where it was clicked. To make the page open in a new tab, we set target
to _blank
.
We also set the rel
prop to noreferrer
for security purposes. It prevents the opened page from gaining any information about the page that it was opened from.
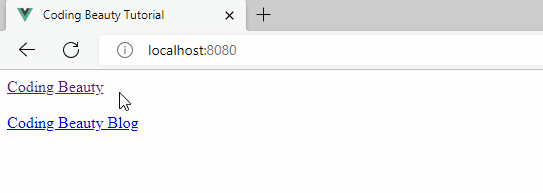
Open link in new tab on button click
Sometimes we’ll prefer a button instead of a link to open the new tab when clicked.
To open a link in a new tab on button click, create a button
element and set an click
event listener that calls the window.open()
method.
App.vue
<template>
<div id="app">
<p>
Click this button to visit Coding Beauty in a new tab
</p>
<button
role="link"
@click="openInNewTab('https://codingbeautydev.com')"
>
Click
</button>
</div>
</template>
<script>
export default {
methods: {
openInNewTab(url) {
window.open(url, '_blank', 'noreferrer');
},
},
};
</script>
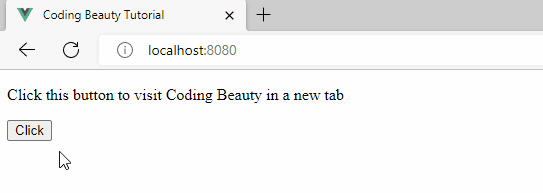
We use the open() method of the window
object to programmatically open a link in a new tab. This method has three optional parameters:
url
: The URL of the page to open in a new tab.target
: like thetarget
attribute of the<a>
element, this parameter’s value specifies where to open the linked document, i.e., the browsing context. It accepts all the values thetarget
attribute of the<a>
element accepts.windowFeatures
: A comma-separated list of feature options for the window.noreferrer
is one of these options.
Passing _blank
to the target
parameter makes the link get opened in a new tab.
When the button is clicked, the event listener is called, which in turn calls window.open()
, which opens the specified link in a new tab.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
