To subtract 6 months from a date:
- Call the
getMonth()
method on the date to get the months. - Subtract 6 from the return value of
getMonth()
. - Pass the result of the subtraction to the
setMonth()
method.
For example:
function subtract6Months(date) {
date.setMonth(date.getMonth() - 6);
return date;
}
// November 14, 2022
const date = new Date('2022-11-14T00:00:00.000Z');
const newDate = subtract6Months(date);
// May 14, 2022
console.log(newDate); // 2022-05-14T00:00:00.000Z
Our subtract6Months()
function takes a Date
object and the number of months to subtract as arguments. It returns the same Date
object with 6 months subtracted.
The Date getMonth() returns a zero-based number that represents the month of a particular date.
The Date setMonth() method sets the months of a date to a specified zero-based number.
Note: “Zero-based” here means that 0
is January, 1
is February, 2
is March, etc.
If the months subtracted would decrease the year of the date, setMonths()
will automatically update the date information to reflect this.
// January 10, 2022
const date = new Date('2022-01-10T00:00:00.000Z');
date.setMonth(date.getMonth() - 6);
// July 10, 2021
console.log(date); // 2021-07-10T00:00:00.000Z
In this example, we subtract 6 months from a date in January 2022. This makes the year automatically get rolled back to 2021 by setMonth()
.
Avoid side-effects
The setMonth()
mutates the Date
object it is called on. This introduces a side effect into our subtract6Months()
function. To avoid modifying the passed Date
and create a pure function, make a copy of the Date
and call setMonth()
on this copy, instead of the original.
function subtract6Months(date) {
// 👇 Make copy with "Date" constructor
const dateCopy = new Date(date);
dateCopy.setMonth(dateCopy.getMonth() - 6);
return dateCopy;
}
// August 13, 2022
const date = new Date('2022-08-13T00:00:00.000Z');
const newDate = subtract6Months(date);
// February 13, 2022
console.log(newDate); // 2022-02-13T00:00:00.000Z
// 👇 Original not modified
console.log(date); // 2022-08-13T00:00:00.000Z
Tip: Functions that don’t modify external state (i.e., pure functions) tend to be more predictable and easier to reason about, as they always give the same output for a particular input. This makes it a good practice to limit the number of side effects in your code.
2. date-fns subMonths()
function
Alternatively, we can use the subMonths()
function from the date-fns NPM package to quickly subtract 6 months from a date. It works like our pure subtractMonths()
function. subMonths()
takes a Date
object and the number of months to subtract as arguments. It returns a new Date
object with the months subtracted.
import { subMonths } from 'date-fns';
// July 26, 2022
const date = new Date('2022-07-26T00:00:00.000Z');
const newDate = subMonths(date, 6);
// January 26, 2022
console.log(newDate); // 2022-01-26T00:00:00.000Z
// Original not modified
console.log(date); // 2022-07-26T00:00:00.000Z
Note that the subMonths()
function returns a new Date
object without modifying the one passed to it.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
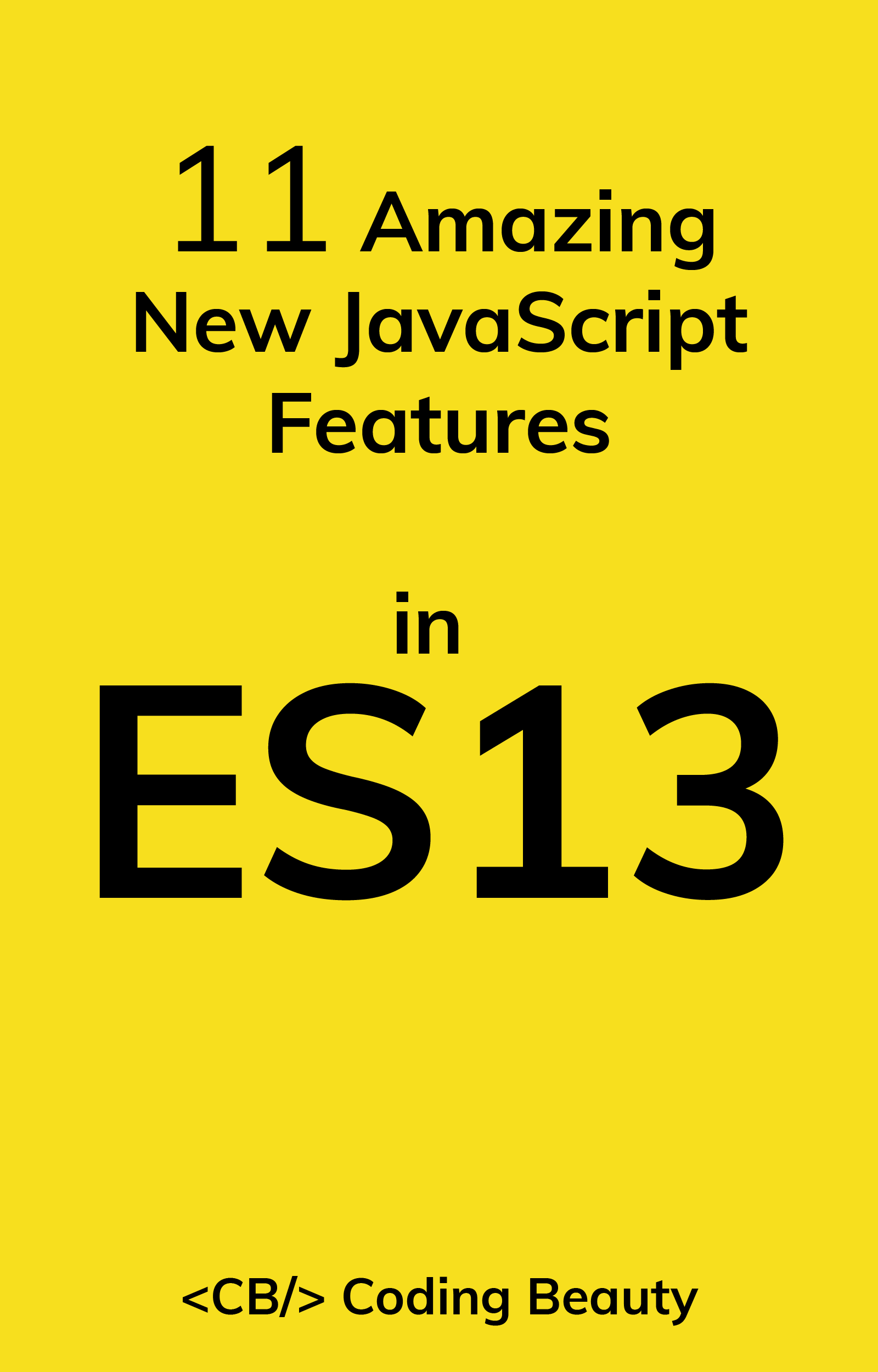