1. Date
getMonth()
and setMonth()
methods
To subtract months from a date in JavaScript:
- Call the
getMonth()
method on theDate
to get the months. - Subtract the months.
- Pass the result of the subtraction to the
setMonth()
method.
For example:
function subtractMonths(date, months) {
date.setMonth(date.getMonth() - months);
return date;
}
// August 13, 2022
const date = new Date('2022-08-13T00:00:00.000Z');
const newDate = subtractMonths(date, 3);
// May 13, 2022
console.log(newDate); // 2022-05-13T00:00:00.000Z
Our subtractMonths()
function takes a Date
object and the number of months to subtract as arguments. It returns the same Date
object with the months subtracted.
The Date
getMonth()
method returns a zero-based number that represents the month of a particular date.
The Date
setMonth()
method sets the months of a date to a specified zero-based number.
Note: “Zero-based” here means that 0
is January, 1
is February, 2
is March, etc.
If the months subtracted would decrease the year of the date, setMonth()
will automatically update the date information to reflect this.
// January 10, 2022
const date = new Date('2022-01-10T00:00:00.000Z');
date.setMonth(date.getMonth() - 2);
// November 10, 2021: year decreased by 1
console.log(date); // 2021-11-10T00:00:00.000Z
In this example, we subtracted 2 months from a date in January 2022. This makes setMonth()
automatically roll the year back to 2021.
Avoid side-effects
The setMonth()
method mutates the Date
object it is called on. This introduces a side effect into our subtractMonths()
function. To avoid modifying the passed Date
and create a pure function, make a copy of the Date
and call setMonth()
on this copy, instead of the original.
function subtractMonths(date, months) {
// π Make copy with "Date" constructor
const dateCopy = new Date(date);
dateCopy.setMonth(dateCopy.getMonth() - months);
return dateCopy;
}
// August 13, 2022
const date = new Date('2022-08-13T00:00:00.000Z');
const newDate = subtractMonths(date, 3);
// May 13, 2022
console.log(newDate); // 2022-05-13T00:00:00.000Z
// π Original not modified
console.log(date); // 2022-08-13T00:00:00.000Z
Tip: Functions that donβt modify external state (i.e., pure functions) tend to be more predictable and easier to reason about, as they always give the same output for a particular input. This makes it a good practice to limit the number of side effects in your code.
2. date-fns
subMonths()
function
Alternatively, we can use the subMonths()
function from the date-fns
NPM package to quickly subtract months from a date. It works like our pure subtractMonths()
function.
import { subMonths } from 'date-fns';
// June 27, 2022
const date = new Date('2022-06-27T00:00:00.000Z');
const newDate = subMonths(date, 4);
// February 27, 2022
console.log(newDate); // 2022-02-27T00:00:00.000Z
// π Original not modified
console.log(date); // 2022-06-27T00:00:00.000Z
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
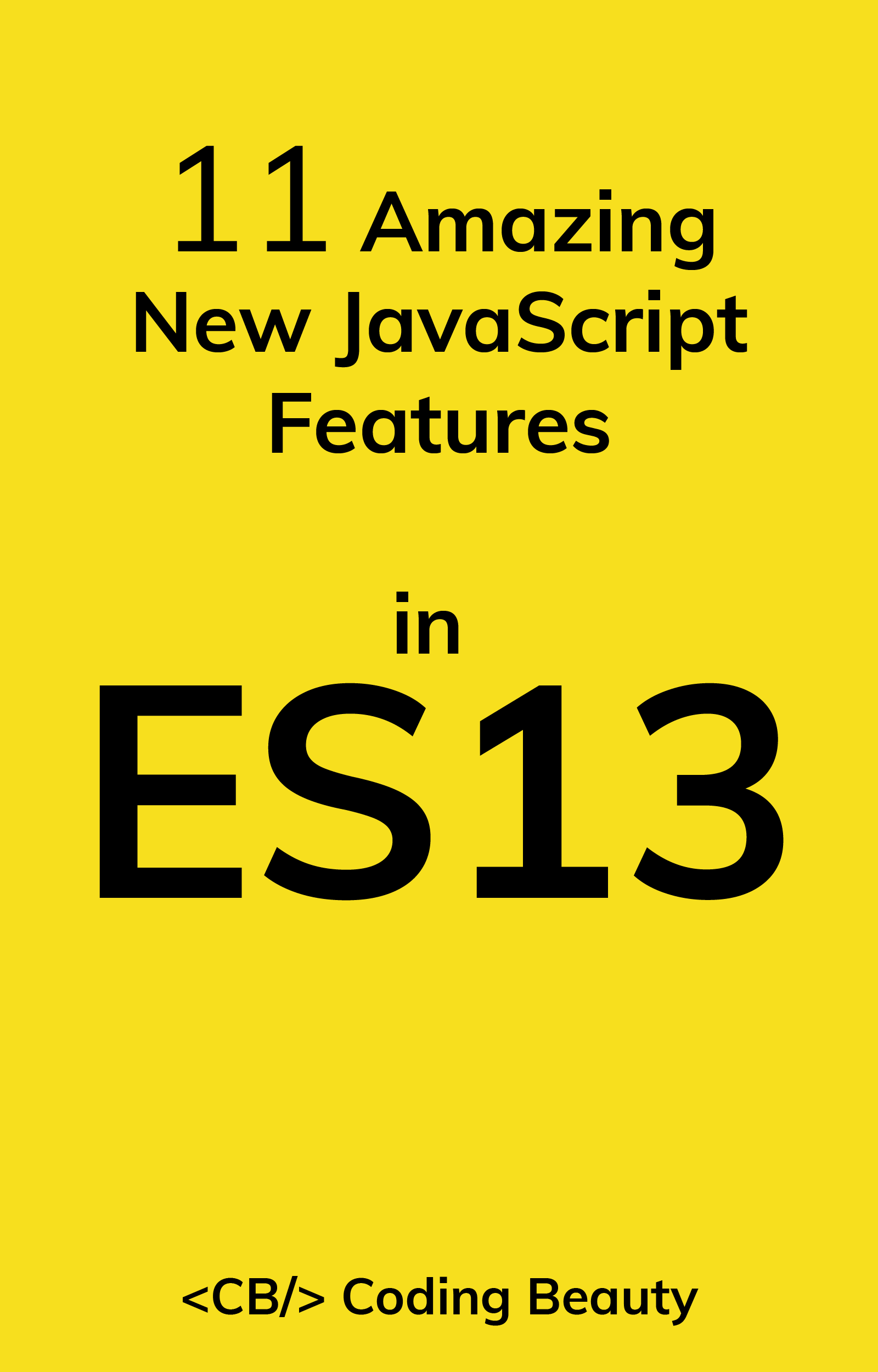