To get the value of an input on change in React, set an onChange
event handler on the input, then use the target.value
property of the Event
object passed to the handler to get the input value.
For example:
App.js
import { useState } from 'react';
export default function App() {
const [message, setMessage] = useState('');
const handleChange = (event) => {
// 👇 Get input value from "event"
setMessage(event.target.value);
};
return (
<div>
<input
type="text"
id="message"
name="message"
onChange={handleChange}
/>
<h2>Message: {message}</h2>
</div>
);
}
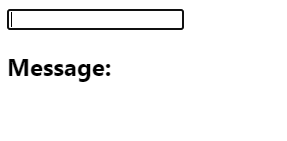
Here we create a state variable (message
) to track the input value. By setting an onChange
event handler, the handler function will get called whenever the text in the input field changes.
The handler function will be called with an Event object containing data and allowing actions related to the event. The target property of this object lets us access the object representing the input element.
This input element object has a value
property which returns the text currently in the input field. So we call the setMessage()
function with this property as an argument to update the message
variable with the input value, and this reflects on the page after the DOM is updated.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
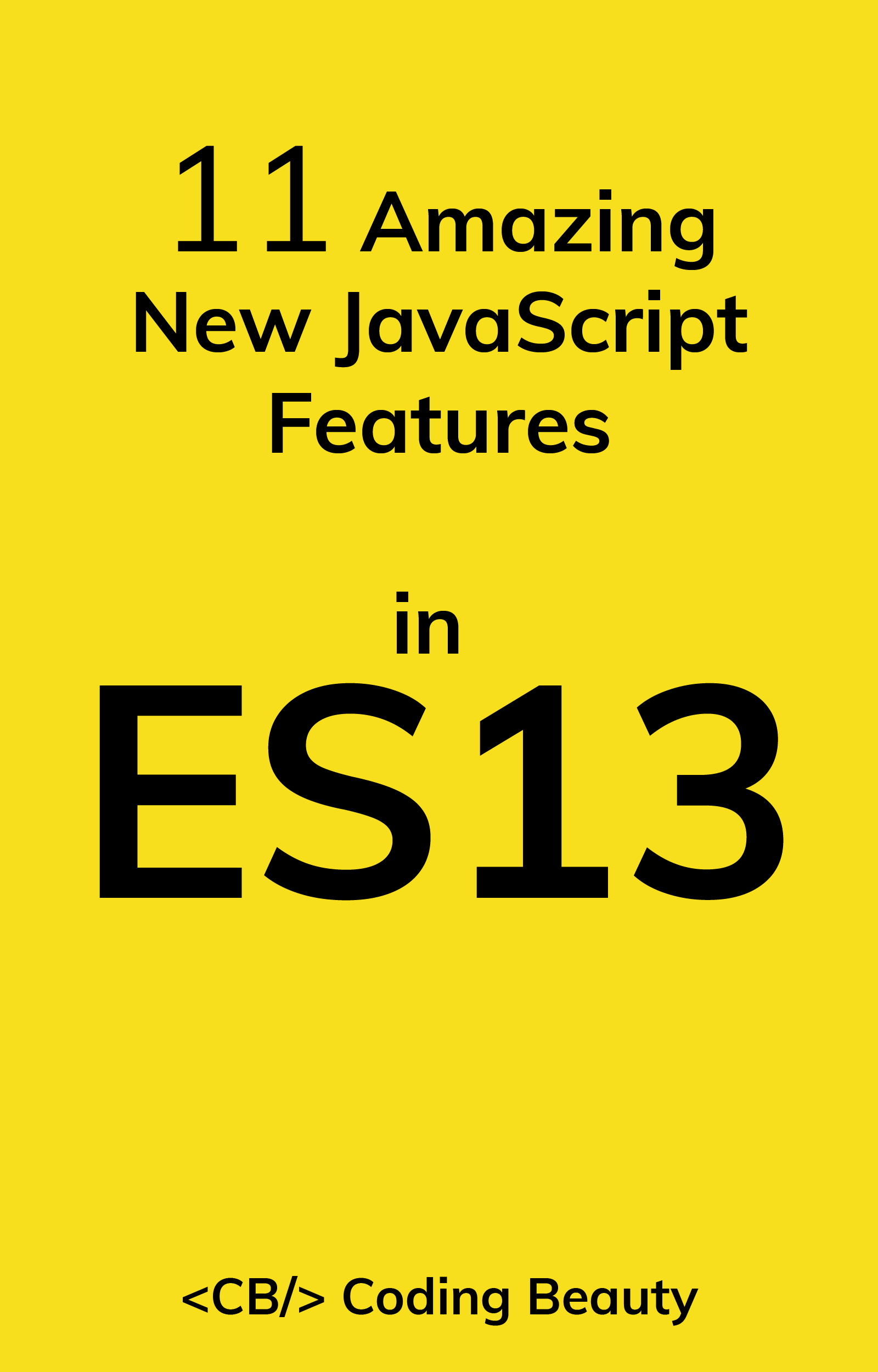