Let’s learn how to easily subtract any number of hours from a Date
object in JavaScript.
1. Date setHours and getHours() Methods
To subtract hours from a Date
:
- Call the
getHours()
method on theDate
to get the number of hours. - Subtract the hours.
- Pass the result of the subtraction to the
setHours()
method.
function subtractHours(date, hours) {
date.setHours(date.getHours() - hours);
return date;
}
// 8 AM on June 20, 2022
const date = new Date('2022-06-20T08:00:00.000Z');
const newDate = subtractHours(date, 2);
// 6 AM on June 20, 2022
console.log(date); // 2022-06-20T06:00:00.000Z
Our subtractHours()
function takes a Date
object and the number of hours to subtract as arguments. It returns the same Date
object with the hours subtracted.
The Date getHours()
method returns a number between 0
and 23
that represents the hours of a particular Date
.
The Date setHours()
method sets the hours of a Date
to a specified number.
If the hours we subtract would decrease the day, month, or year of the Date
, setHours()
automatically updates the Date
information to reflect this.
// 12 AM on June 20, 2022
const date = new Date('2022-06-20T00:00:00.000Z');
date.setHours(date.getHours() - 3);
// 9 PM on June 19, 2022 (previous day)
console.log(date); // 2022-06-19T21:00:00.000Z
In this example, decreasing the hours of the Date
by 3
decreases the day by 1 and sets the hours to 21
.
Avoiding Side Effects
The setHours()
method mutates the Date
object it is called on. This introduces a side effect into our subtractHours()
function. To avoid modifying the passed date and create a pure function, make a copy of the date and call setHours()
on this copy, instead of the original:
function subtractHours(date, hours) {
const dateCopy = new Date(date);
dateCopy.setHours(dateCopy.getHours() - hours);
return date;
}
// 8 AM on June 20, 2022
const date = new Date('2022-06-20T08:00:00.000Z');
const newDate = subtractHours(date, 2);
// 6 AM on June 20, 2022
console.log(date); // 2022-06-20T06:00:00.000Z
// Original not modified
console.log(newDate); // 2022-06-20T08:00:00.000Z
Tip: Functions that don’t modify external state (i.e., pure functions) tend to be more predictable and easier to reason about. This makes it a good practice to limit the number of side effects in your code.
2. date-fns subHours() Function
Alternatively, we can use the subHours()
function from the date-fns NPM package to quickly subtract hours from a Date
. It works similarly to our pure subtractHours()
function.
import { subHours } from 'date-fns';
// 8 AM on June 20, 2022
const date = new Date('2022-06-20T08:00:00.000Z');
const newDate = subHours(date, 2);
// 6 AM on June 20, 2022
console.log(date); // 2022-06-20T06:00:00.000Z
// Original not modified
console.log(newDate); // 2022-06-20T08:00:00.000Z
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
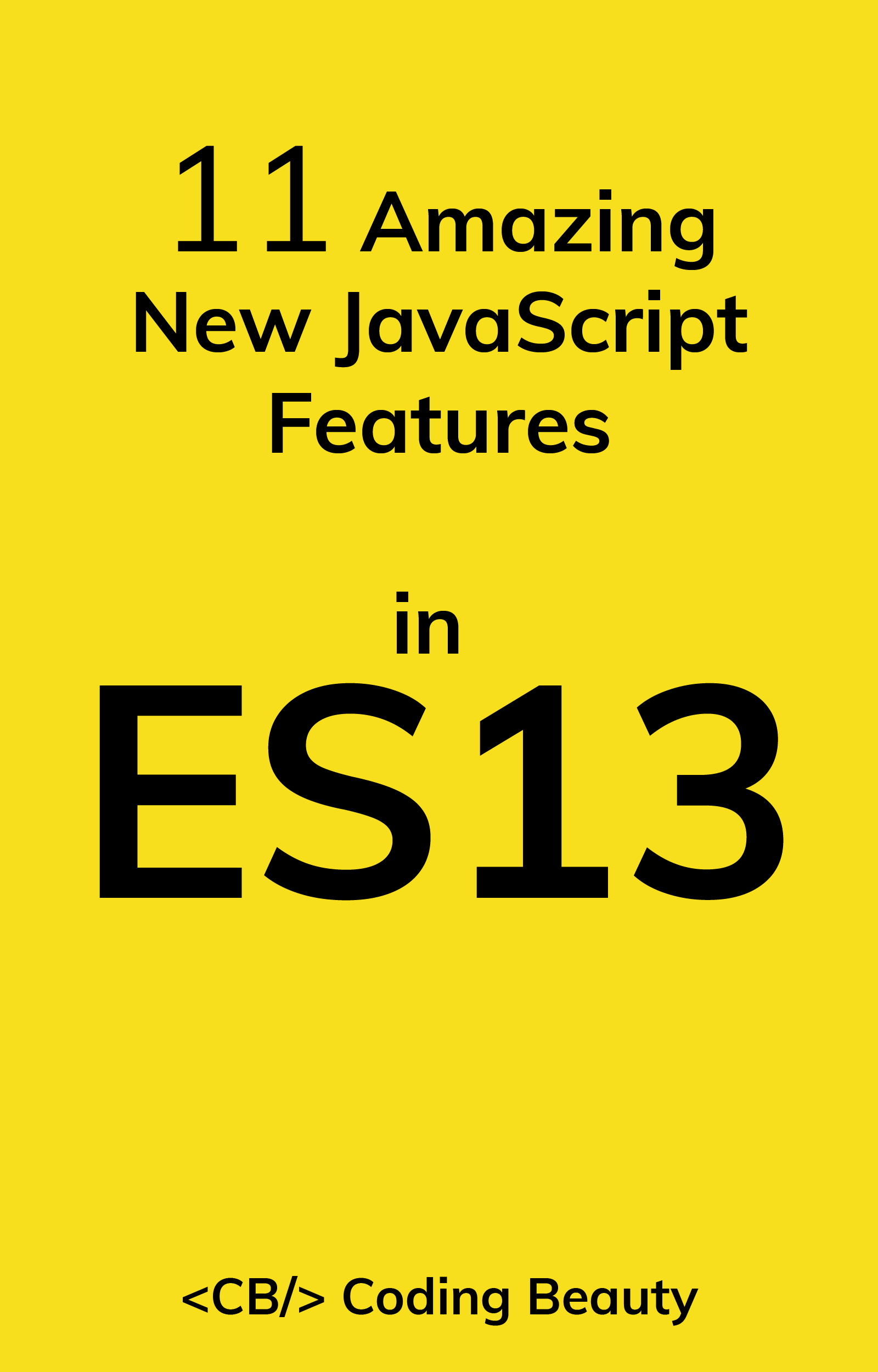