In this article, we’ll learn how to use JavaScript to get the position of a month in the list of the 12 months from its name. That is, we’ll get 1
from January
, 2
from February
, 3
from March
, and so on.
To do this, create a date string from the name and pass this string to the Date()
constructor. Then use the getMonth()
method to get the month number. For example:
function getMonthNumberFromName(monthName) {
return new Date(`${monthName} 1, 2022`).getMonth() + 1;
}
console.log(getMonthNumberFromName('January')); // 1
console.log(getMonthNumberFromName('February')); // 2
console.log(getMonthNumberFromName('March')); // 3
We interpolate the month into a string that can be parsed by the Date()
constructor to create a new Date
object. Then we use the getMonth()
method to get the month of the date.
The getMonth()
method returns a zero-based index of the month, i.e., 0
for January
, 1
for February
, 2
for March
, etc.
const month1 = new Date('January 1, 2022').getMonth();
console.log(month1); // 0
const month2 = new Date('February 1, 2022').getMonth();
console.log(month2); // 1
This is why we add 1
to it and return the sum from as the result.
Our function will also work for abbreviated month names, as the Date()
constructor can also parse date strings with them.
function getMonthNumberFromName(monthName) {
return new Date(`${monthName} 1, 2022`).getMonth() + 1;
}
console.log(getMonthNumberFromName('Jan')); // 1
console.log(getMonthNumberFromName('Feb')); // 2
console.log(getMonthNumberFromName('Mar')); // 3
If the month name is such that the resulting date string can’t be parsed by the Date()
constructor, an invalid date will be created and getMonth()
will return NaN
.
const date = new Date('Invalid 1, 2022');
console.log(date); // Invalid Date
console.log(date.getMonth()); // NaN
This means our function will return NaN
as well:
function getMonthNumberFromName(monthName) {
return new Date(`${monthName} 1, 2022`).getMonth() + 1;
}
console.log(getMonthNumberFromName('Invalid')); // NaN
It might be better if we return -1
instead. We can check for an invalid date with the isNaN()
function.
function getMonthNumberFromName(monthName) {
const date = new Date(`${monthName} 1, 2022`);
if (isNaN(date)) return -1;
return date.getMonth() + 1;
}
console.log(getMonthNumberFromName('Invalid')); // -1
Alternatively, we could throw an error:
function getMonthNumberFromName(monthName) {
const date = new Date(`${monthName} 1, 2022`);
if (isNaN(date)) {
throw new Error('Invalid month name.');
}
return date.getMonth() + 1;
}
// Error: Invalid month name.
console.log(getMonthNumberFromName('Invalid'));
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
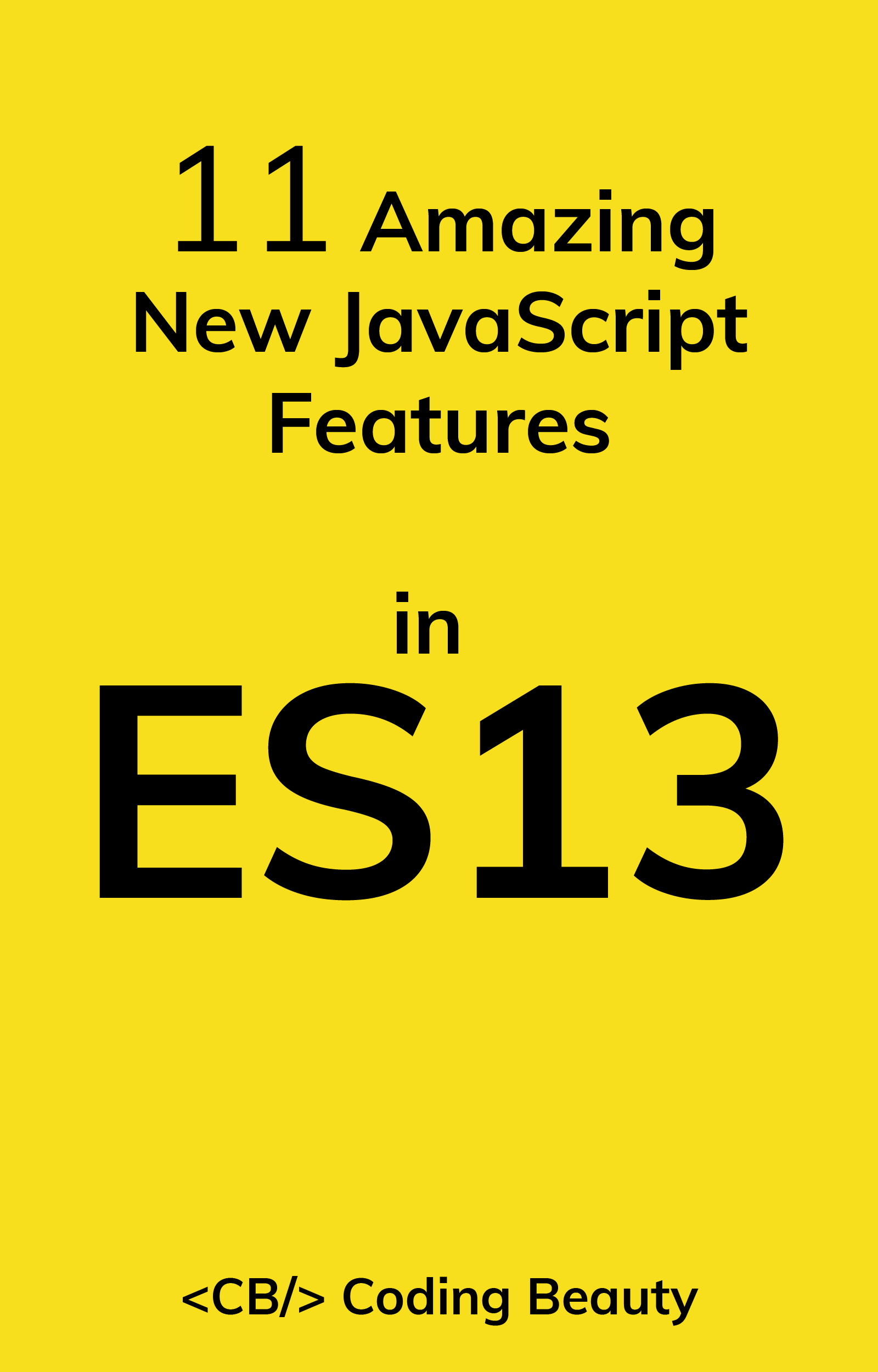