In this article, we’ll be looking at multiple ways to easily check if a string is a valid URL in JavaScript.
1. Catch Exception from URL() Constructor
To check if a string is a URL, pass the string to the URL()
constructor. If the string is a valid URL, a new URL
object will be created successfully. Otherwise, an error will be thrown. Using a try...catch
block, we can handle the error and perform the appropriate action when the URL is invalid.
For example:
function isValidUrl(string) {
try {
new URL(string);
return true;
} catch (err) {
return false;
}
}
console.log(isValidUrl('https://codingbeautydev.com')); // true
console.log(isValidUrl('app://codingbeautydev.com')); // true
console.log(isValidUrl('Coding Beauty')); // false
To check for a valid HTTP URL, we can use the protocol
property of the URL
object.
function isValidHttpUrl(string) {
try {
const url = new URL(string);
return url.protocol === 'http:' || url.protocol === 'https:';
} catch (err) {
return false;
}
}
console.log(isValidHttpUrl('https://codingbeautydev.com')); // true
console.log(isValidHttpUrl('app://codingbeautydev.com')); // false
console.log(isValidHttpUrl('Coding Beauty')); // false
The URL
protocol
property returns a string representing the protocol scheme of the URL, including the final colon (:
). HTTP URLs have a protocol
of either http:
or https:
.
2. Regex Matching
Alternatively, we can check if a string is a URL using a regular expression. We do this by calling the test()
method on a RegExp
object with a pattern that matches a string that is a valid URL.
function isValidUrl(str) {
const pattern = new RegExp(
'^([a-zA-Z]+:\\/\\/)?' + // protocol
'((([a-z\\d]([a-z\\d-]*[a-z\\d])*)\\.)+[a-z]{2,}|' + // domain name
'((\\d{1,3}\\.){3}\\d{1,3}))' + // OR ip (v4) address
'(\\:\\d+)?(\\/[-a-z\\d%_.~+]*)*' + // port and path
'(\\?[;&a-z\\d%_.~+=-]*)?' + // query string
'(\\#[-a-z\\d_]*)?$', // fragment locator
'i'
);
return pattern.test(str);
}
console.log(isValidUrl('https://codingbeautydev.com')); // true
console.log(isValidUrl('app://codingbeautydev.com')); // true
console.log(isValidUrl('Coding Beauty')); // false
The RegExp
test()
method searches for a match between a regular expression and a string. It returns true
if it finds a match. Otherwise, it returns false
.
To check for a valid HTTP URL, we can alter the part of the regex that matches the URL protocol:
function isValidHttpUrl(str) {
const pattern = new RegExp(
'^(https?:\\/\\/)?' + // protocol
'((([a-z\\d]([a-z\\d-]*[a-z\\d])*)\\.)+[a-z]{2,}|' + // domain name
'((\\d{1,3}\\.){3}\\d{1,3}))' + // OR ip (v4) address
'(\\:\\d+)?(\\/[-a-z\\d%_.~+]*)*' + // port and path
'(\\?[;&a-z\\d%_.~+=-]*)?' + // query string
'(\\#[-a-z\\d_]*)?$', // fragment locator
'i'
);
return pattern.test(str);
}
console.log(isValidHttpUrl('https://codingbeautydev.com')); // true
console.log(isValidHttpUrl('app://codingbeautydev.com')); // false
console.log(isValidHttpUrl('Coding Beauty')); // false
The https?
: pattern will only match either http:
or https:
.
3. is-url and is-url-http NPM Packages
We can also use the is-url NPM package to quickly check if a string is a valid URL.
import isUrl from 'is-url';
console.log(isUrl('https://codingbeautydev.com')); // true
console.log(isUrl('app://codingbeautydev.com')); // true
console.log(isUrl('Coding Beauty')); // false
To quickly check if a string is a valid HTTP URL, we can use the is-url-http package from NPM.
import isUrlHttp from 'is-url-http';
console.log(isUrlHttp('https://codingbeautydev.com')); // true
console.log(isUrlHttp('app://codingbeautydev.com')); // false
console.log(isUrlHttp('Coding Beauty')); // false
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
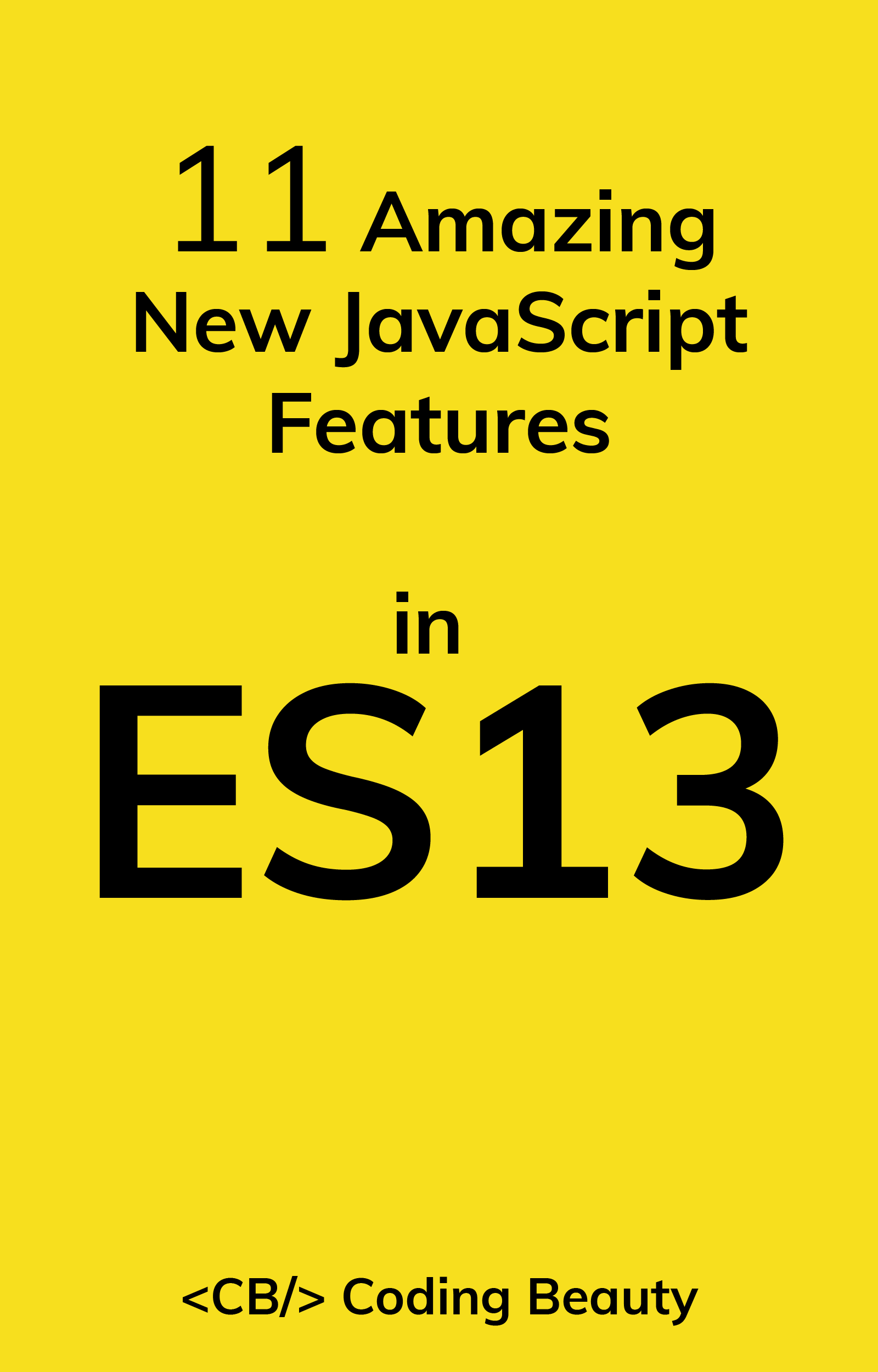