1. Date setMinutes() and getMinutes() Methods
To add minutes to a Date
in JavaScript, call the getMinutes()
method on the Date
to get the minutes, then call the setMinutes()
method on the Date
, passing the sum of getMinutes()
and the minutes to add.
function addMinutes(date, minutes) {
date.setMinutes(date.getMinutes() + minutes);
return date;
}
const date = new Date('2022-05-15T00:00:00.000Z');
const newDate = addMinutes(date, 10);
// 2022-05-15T00:10:00.000Z
console.log(newDate);
Our addMinutes()
function takes a Date
and the number of minutes to add as arguments, and returns the same Date
with the newly added minutes.
The Date
getMinutes()
method returns a number between 0 and 59 that represents the minutes of the Date
.
The Date
setMinutes()
method takes a number representing minutes and set the minutes of the Date
to that number.
If the minutes you specify would change the hour, day, month, or year of the Date
, setMinutes()
automatically updates the Date
information to reflect this.
// 12:00 AM on May 15, 2022
const date = new Date('2022-05-15T00:00:00.000Z');
date.setMinutes(date.getMinutes() + 150);
// 2:30 AM on May 15, 2022
console.log(date); // 2022-05-15T02:30:00.000Z
In this example, passing 150
to setMinutes()
increments the Date
hours by 2 (120 minutes) and sets the minutes to 30
.
Avoiding Side Effects
The setMinutes()
method mutates the Date
object it is called on. This introduces a side effect into our addMinutes()
function. To avoid modifying the passed Date
and create a pure function, make a copy of the Date
and call setMinutes()
on this copy, instead of the original:
function addMinutes(date, minutes) {
const dateCopy = new Date(date);
dateCopy.setMinutes(date.getMinutes() + minutes);
return dateCopy;
}
const date = new Date('2022-05-15T00:00:00.000Z');
const newDate = addMinutes(date, 10);
console.log(newDate); // 2022-05-15T00:10:00.000Z
// Original not modified
console.log(date); // 2022-05-15T00:00:00.000Z
Tip
Functions that don’t modify external state (i.e., pure functions) tend to be more predictable and easier to reason about. This makes it a good practice to limit the number of side-effects in your programs.
2. date-fns addMinutes()
Alternatively, you can use the pure addMinutes()
function from the date-fns
NPM package to quickly add minutes to a Date
.
import { addMinutes } from 'date-fns';
const date = new Date('2022-05-15T00:00:00.000Z');
const newDate = addMinutes(date, 10);
console.log(newDate); // 2022-05-15T00:10:00.000Z
// Original not modified.
console.log(date); // 2022-05-15T00:00:00.000Z
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
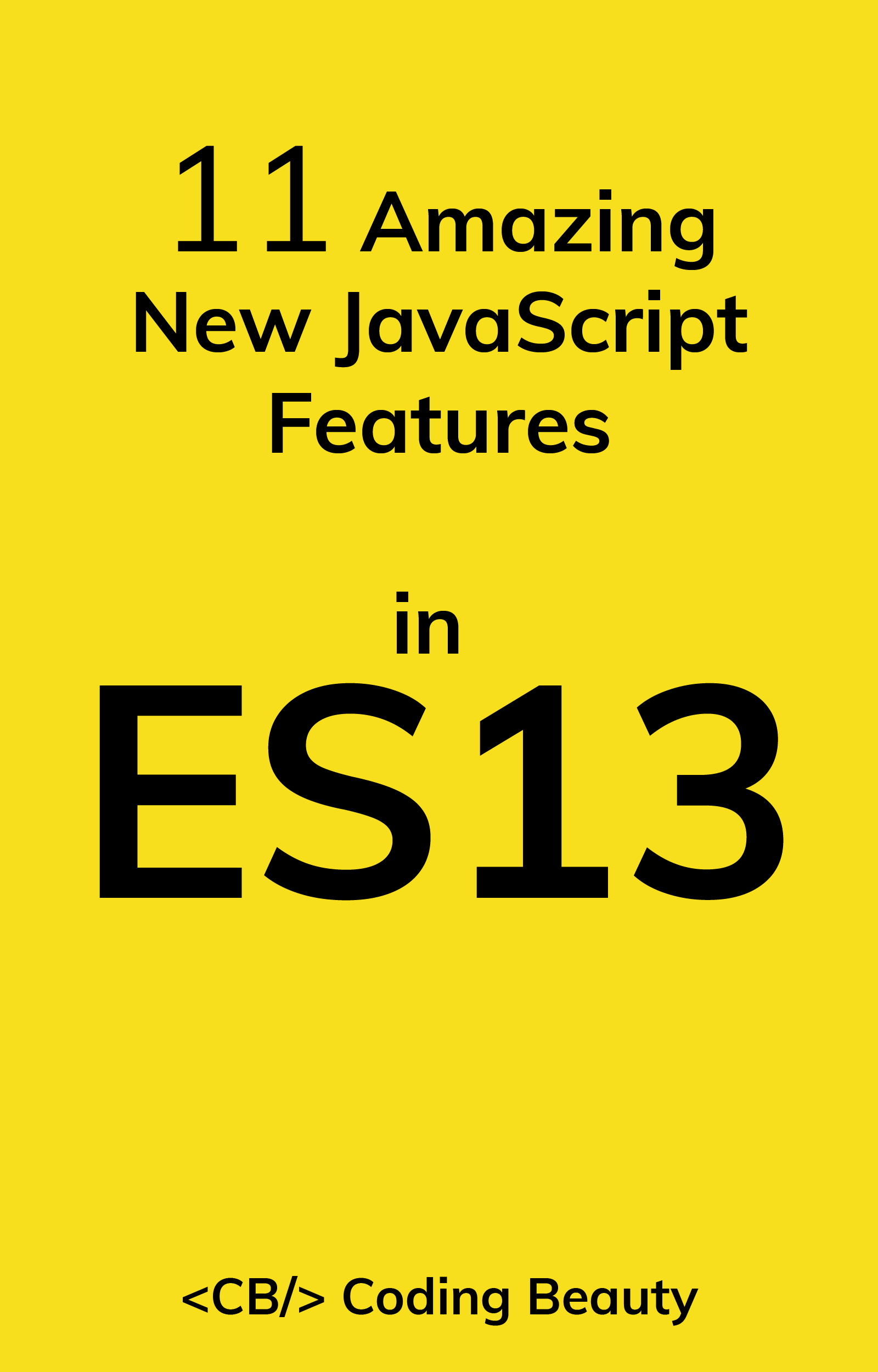