To convert an array to a string without commas in JavaScript, call the join()
method on the array, passing an empty string (''
) as an argument:
const arr = ['coffee', 'milk', 'tea'];
const withoutCommas = arr.join('');
console.log(withoutCommas); // coffeemilktea
The Array
join()
method returns a string containing each array element concatenated with the specified separator. If no separator is passed as an argument, it will join the array elements with commas:
const arr = ['coffee', 'milk', 'tea'];
const str = arr.join();
console.log(str); // coffee,milk,tea
We can specify other separators apart from an empty string, like hyphens and slashes:
const arr = ['coffee', 'milk', 'tea'];
const withHypens = arr.join('-');
console.log(withHypens); // coffee-milk-tea
const withSlashes = arr.join('/');
console.log(withSlashes); // coffee/milk/tea
const withSpaces = arr.join(' ');
console.log(withSpaces); // coffee milk tea
A separator can also contain multiple characters:
const arr = ['coffee', 'milk', 'tea'];
const withAnd = arr.join(' and ');
console.log(withAnd); // coffee and milk and tea
const withOr = arr.join(' or ');
console.log(withOr); // coffee or milk or tea
If an element in the array is undefined
, null
, or an empty array ([]
), it will be converted to an empty string (''
) before concatenation with the separator. For example:
const arr = ['coffee', null, 'milk', []];
const withComma = arr.join(',');
console.log(withComma); // coffee,,milk,
const withHyphen = arr.join('-');
console.log(withHyphen); // coffee--milk-
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
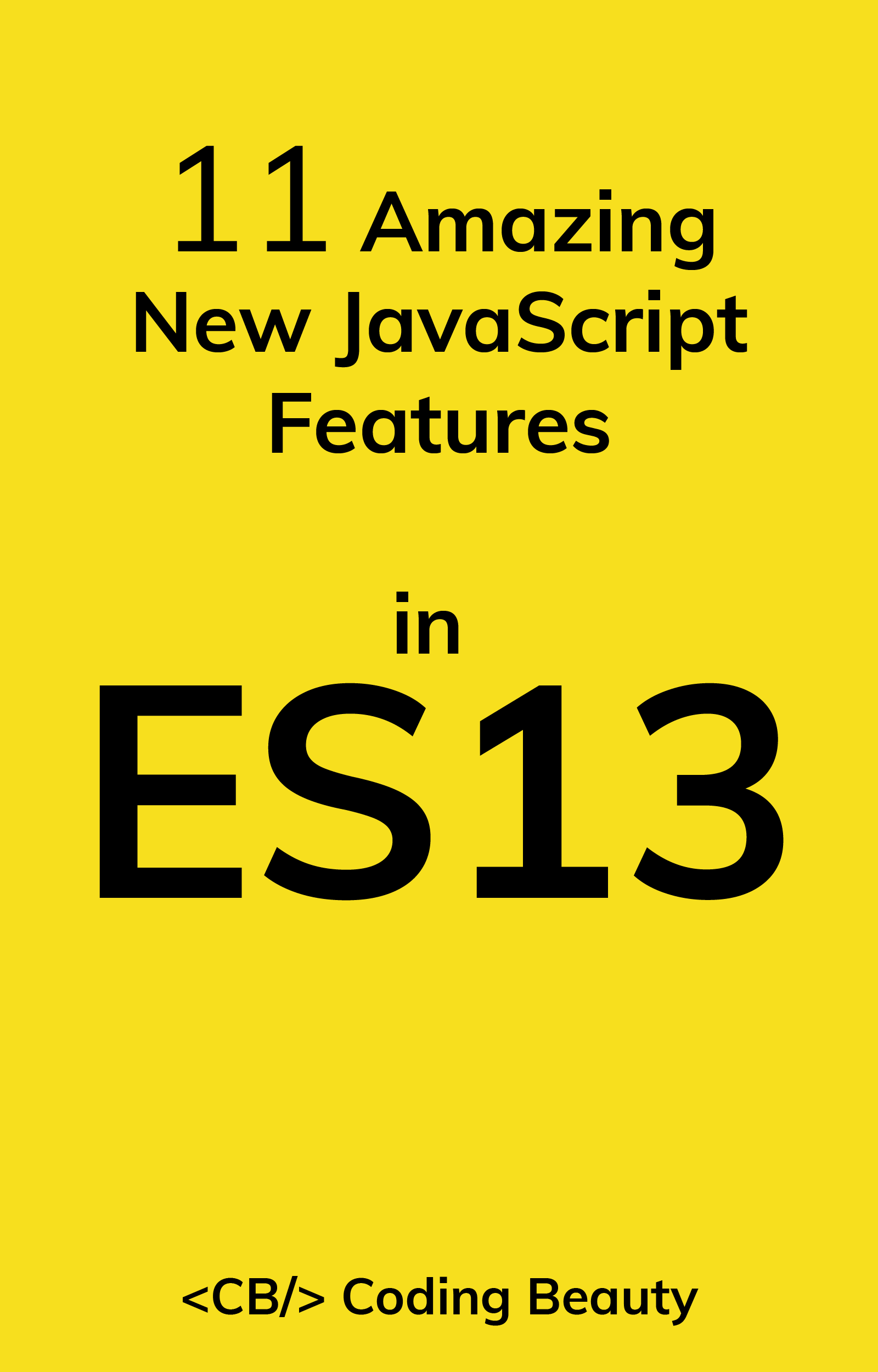