In this article, we’ll be looking at some ways to easily convert a Map
to an object in JavaScript.
1. Object.fromEntries()
To convert a Map to an object, we can use the Object.fromEntries()
method, passing the Map
as an argument. For example:
const map = new Map([
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
]);
const obj = Object.fromEntries(map);
// { user1: 'John', user2: 'Kate', user3: 'Peter' }
console.log(obj);
Note: Object.fromEntries()
can transform any list of key-value pairs into an object. For example, it can directly transform the array of key-value pairs that we passed to the Map()
constructor:
const arr = [
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
];
const obj = Object.fromEntries(arr);
// { user1: 'John', user2: 'Kate', user3: 'Peter' }
console.log(obj);
2. Iterate over Map and Create Keys in Object
Another way to convert a Map
to an object is iterate over the entries of the Map and create a new key on the object for each of them. For each entry, we set the key name and value to the entry name and value respectively. For example:
const map = new Map([
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
]);
const obj = {};
map.forEach((value, key) => {
obj[key] = value;
});
// { user1: 'John', user2: 'Kate', user3: 'Peter' }
console.log(obj);
Note: We can also iterate over the Map
with the for...of
loop:
const map = new Map([
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
]);
const obj = {};
for (const [key, value] of map) {
obj[key] = value;
}
// { user1: 'John', user2: 'Kate', user3: 'Peter' }
console.log(obj);
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
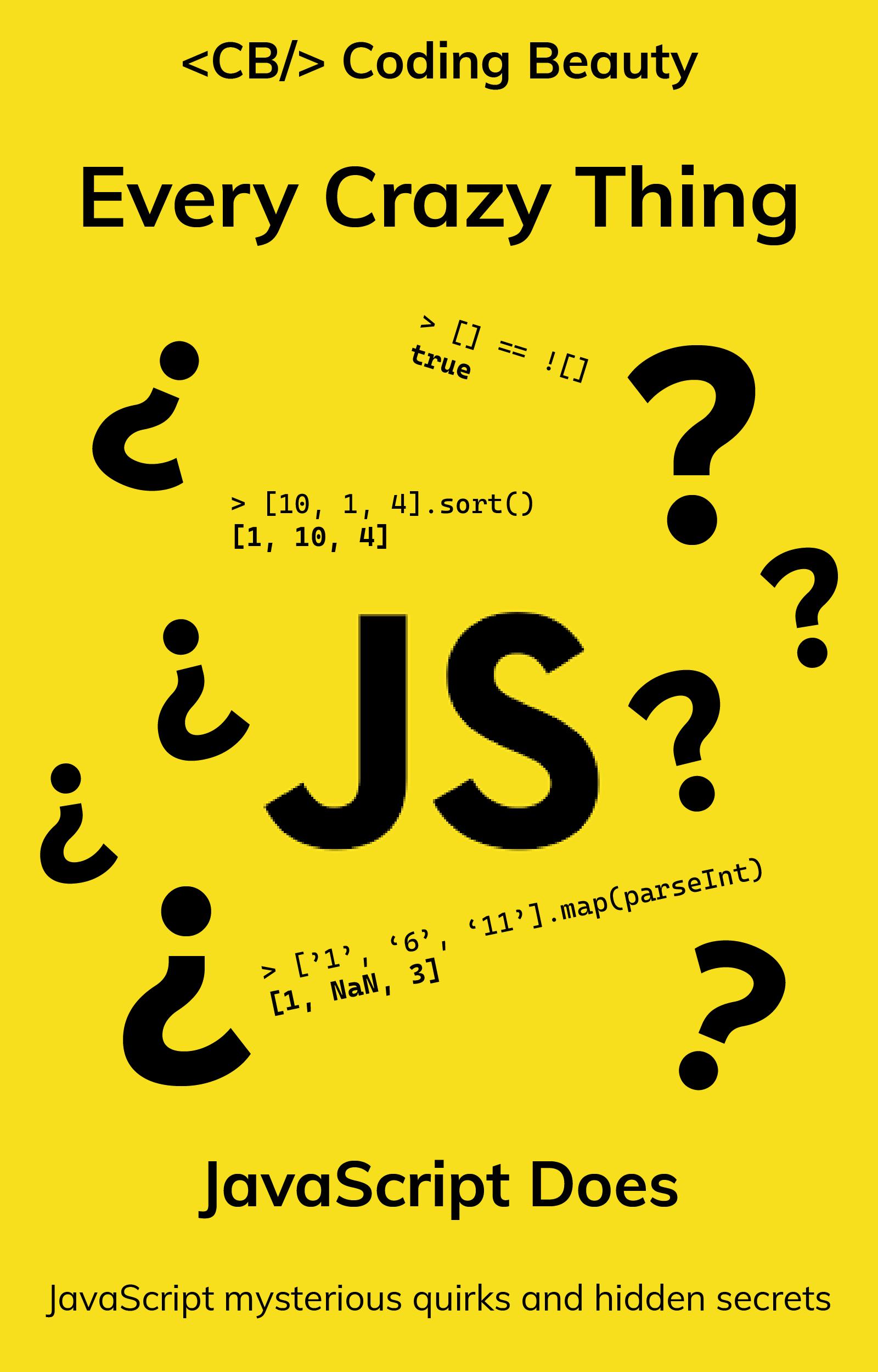