1. Comparing the String with an Empty String
To check if a string is empty in JavaScript, we can compare the string with an empty string (''
) in an if
statement.
For example:
function checkIfEmpty(str) {
if (str === '') {
console.log('String is empty');
} else {
console.log('String is NOT empty');
}
}
const str1 = 'not empty';
const str2 = ''; // empty
checkIfEmpty(str1); // outputs: String is NOT empty
checkIfEmpty(str2); // outputs: String is empty
To treat a string containing only whitespace as empty, call the trim()
method on the string before comparing it with an empty string.
function checkIfEmpty(str) {
if (str.trim() === '') {
console.log('String is empty');
} else {
console.log('String is NOT empty');
}
}
const str1 = 'not empty';
const str2 = ''; // empty
const str3 = ' '; // contains only whitespace
checkIfEmpty(str1); // outputs: String is NOT empty
checkIfEmpty(str2); // outputs: String is empty
checkIfEmpty(str3); // outputs: String is empty
The String
trim()
method removes all whitespace from the beginning and end of a string and returns a new string, without modifying the original.
const str1 = ' bread ';
const str2 = ' milk tea ';
console.log(str1.trim()); // 'bread'
console.log(str2.trim()); // 'milk tea'
Tip
Trimming a string when validating required fields in a form helps ensure that the user entered actual data instead of just whitespace.
How to Check if a String is Empty, null, or undefined
Depending on your scenario, you might want to consider that the string could be a nullish value (null
or undefined
). To check for this, use the string if
statement directly, like this:
function checkIfEmpty(str) {
if (str) {
console.log('String is NOT empty');
} else {
console.log('String is empty');
}
}
const str1 = 'not empty';
const str2 = ''; // empty
const str3 = null;
const str4 = undefined;
checkIfEmpty(str1); // outputs: String is NOT empty
checkIfEmpty(str2); // outputs: String is empty
checkIfEmpty(str3); // outputs: String is empty
checkIfEmpty(str4); // outputs: String is empty
If the string is nullish or empty, it will be coerced to false
in the if
statement. Otherwise, it will be coerced to true
.
To remove all whitespace and also check for a nullish value, use the optional chaining operator (?.
) to call the trim()
method on the string before using it in an if
statement.
function checkIfEmpty(str) {
if (str?.trim()) {
console.log('String is NOT empty');
} else {
console.log('String is empty');
}
}
const str1 = 'not empty';
const str2 = ''; // empty
const str3 = null;
const str4 = undefined;
const str5 = ' '; // contains only whitespace
checkIfEmpty(str1); // outputs: String is NOT empty
checkIfEmpty(str2); // outputs: String is empty
checkIfEmpty(str3); // outputs: String is empty
checkIfEmpty(str4); // outputs: String is empty
checkIfEmpty(str5); // outputs: String is empty
The optional chaining operator lets us call the trim() method on a null
or undefined
string without causing an error. Instead, it prevents the method call and returns undefined
.
const str1 = null;
const str2 = undefined;
console.log(str1?.trim()); // undefined
console.log(str2?.trim()); // undefined
2. Comparing the Length of the String with 0
Alternatively, we can access the length
property of a string and compare its value with 0
to check if the string is empty.
function checkIfEmpty(str) {
if (str.length === 0) {
console.log('String is empty');
} else {
console.log('String is NOT empty');
}
}
const str1 = 'not empty';
const str2 = ''; // empty
checkIfEmpty(str1); // outputs: String is NOT empty
checkIfEmpty(str2); // outputs: String is empty
To check for strings containing only whitespace with this approach, we would also call the trim()
method before comparing the length of the trimmed string with 0
.
function checkIfEmpty(str) {
if (str.trim().length === 0) {
console.log('String is empty');
} else {
console.log('String is NOT empty');
}
}
const str1 = 'not empty';
const str2 = ''; // empty
const str3 = ' '; // contains only whitespace
checkIfEmpty(str1); // outputs: String is NOT empty
checkIfEmpty(str2); // outputs: String is empty
checkIfEmpty(str3); // outputs: String is empty
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
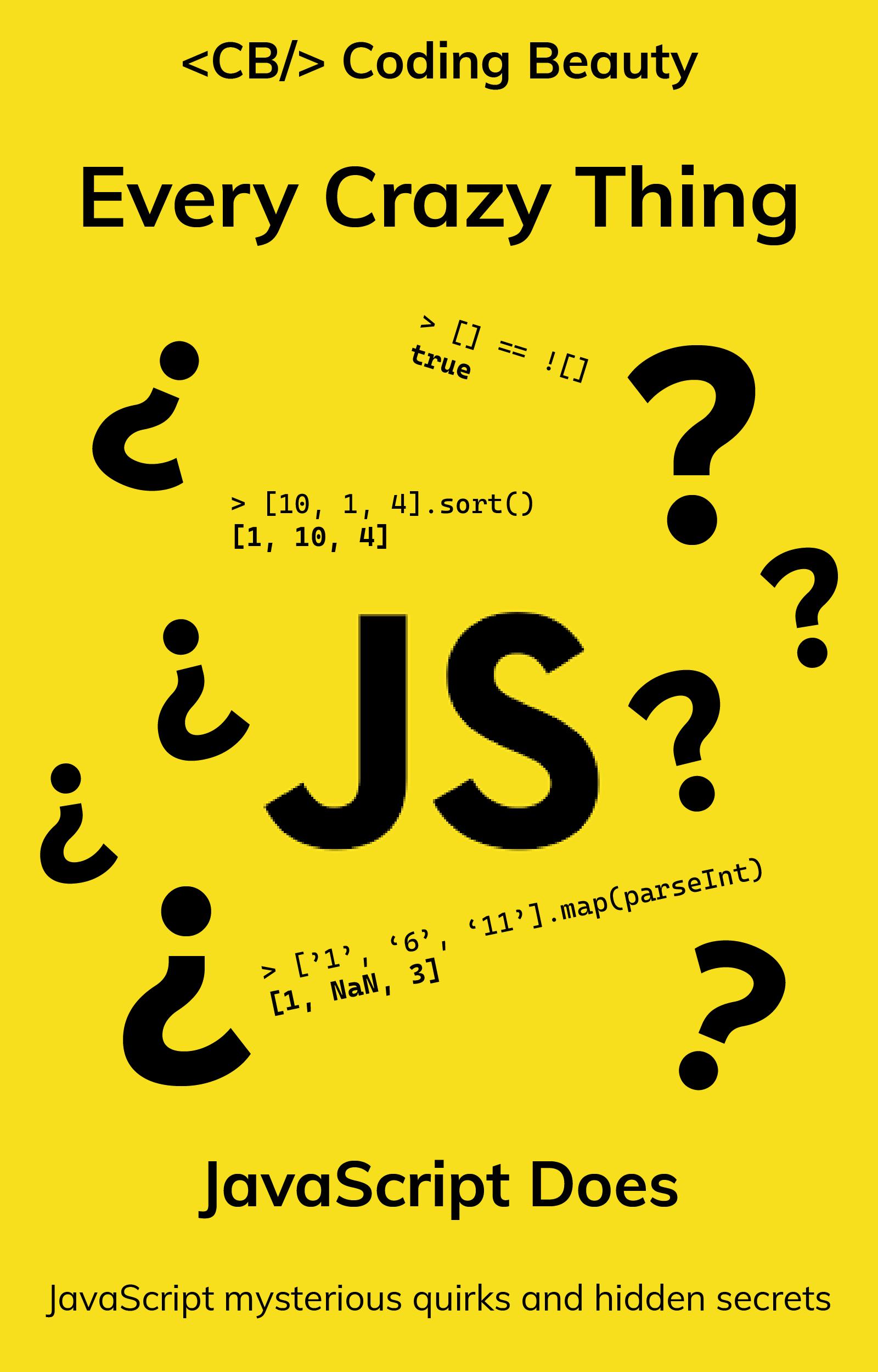