Summary: target
is the innermost element in the DOM that triggered the event, while currentTarget
is the element that the event listener is attached to.
We use the HTML DOM Event
object to get more information about an event and carry out certain actions related to it. Events like click
, mousedown
, and keyup
all have different types of Event
s associated with them. Two key Event
properties are target
and currentTarget
. These properties are somewhat similar and sometimes return the same object, but it’s important that we understand the difference between them so we know which one is better suited for different cases.
An event that occurs on a DOM element bubbles if it is triggered for every single ancestor of the element in the DOM tree up until the root element. When accessing an Event
in an event listener, the target
property returns the innermost element in the DOM that triggered the event (from which bubbling starts). The currentTarget
property, however, will return the element to which the event listener is attached.
Let’s use a simple example to illustrate this. We’ll have three nested div
elements that all listen for the click event with the same handler function.
<!DOCTYPE html>
<html>
<head>
<style>
#div1 {
height: 200px;
width: 200px;
background-color: red;
}
#div2 {
height: 150px;
width: 150px;
background-color: green;
}
#div3 {
height: 100px;
width: 100px;
background-color: blue;
}
</style>
</head>
<body>
<div id="div1" onclick="handleClick(event)">
<div id="div2" onclick="handleClick(event)">
<div id="div3" onclick="handleClick(event)"></div>
</div>
</div>
<script>
function handleClick(event) {
console.log(
`target: ${event.target.id}, currentTarget: ${event.currentTarget.id}`
);
}
</script>
</body>
</html>
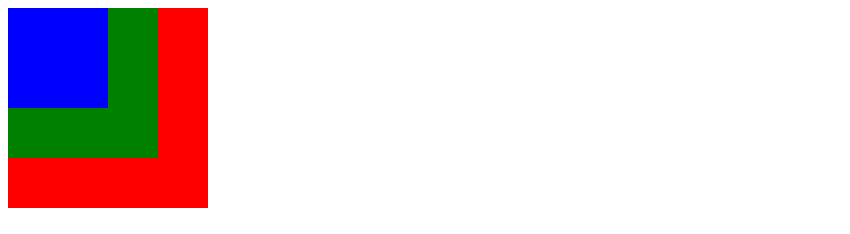
Well, what happens when you click the blue and innermost div
? You get this output in your console:
target: div3, currentTarget: div3
target: div3, currentTarget: div2
target: div3, currentTarget: div1
Clicking the div triggered the event which invoke the handler for div3
. The click
event bubbles, so it was propagated to the two outer divs. As expected, the target
stayed the same in all the listeners but currentTarget
was different in each listener as they were attached to elements at different levels of the DOM hierarchy.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
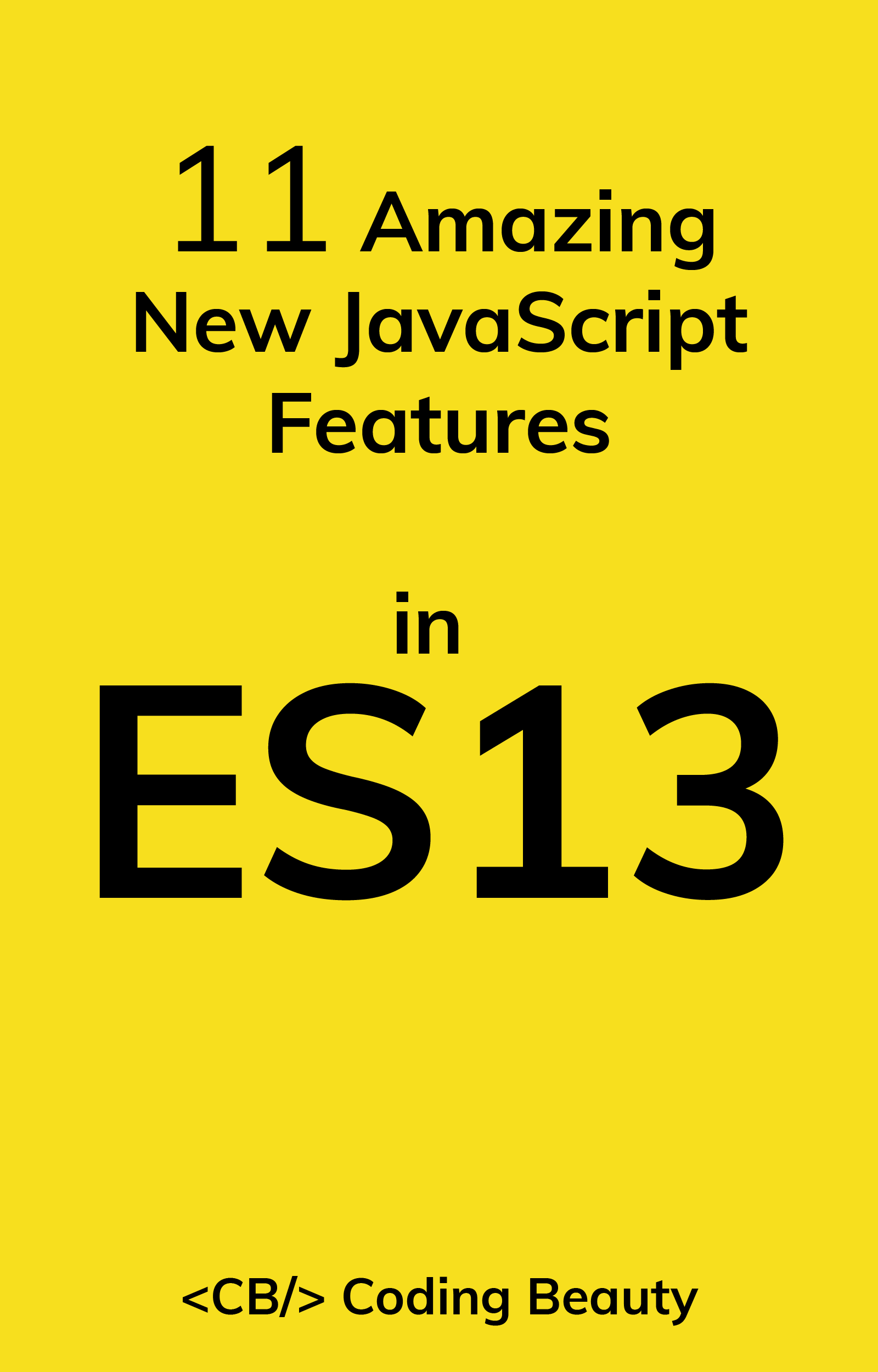