Here’s one:
const groupBy = (arr, groupFn) =>
arr.reduce(
(grouped, obj) => ({
...grouped,
[groupFn(obj)]: [...(grouped[groupFn(obj)] || []), obj],
}),
{}
);
and another:
const randomHexColor = () =>
`#${Math.random().toString(16).slice(2, 8).padEnd(6, '0')}`;
console.log(randomHexColor()); // #7a10ba (varies)
console.log(randomHexColor()); // #65abdc (varies)
Indeed, they are impressive when done right; a nice way to show off language mastery.
But exactly is a one-liner? Is it really code that takes only one line? If so, then can’t every piece of code qualify as a one-liner, if we just remove all the newline characters? Think about it.
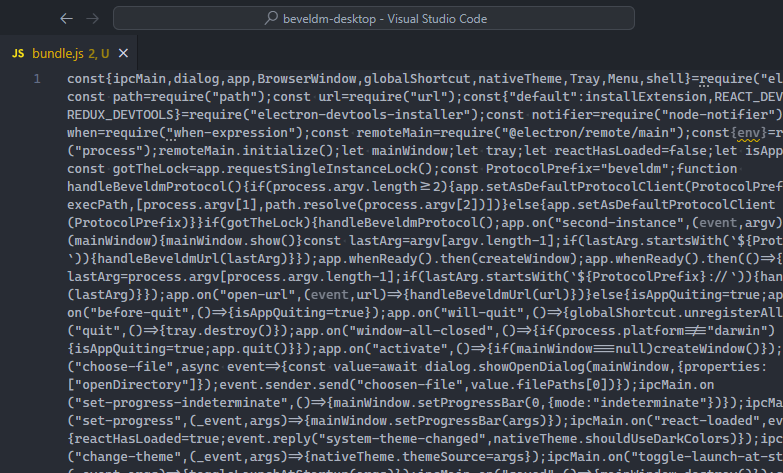
It seems like we need a more rigorous definition of what qualifies as a one-liner. And, after a few minutes of thought when writing a previous article on one-liners, I came up with this:
A one-liner is a code solution to a problem, implemented with a single statement in a particular programming language, optionally using only first-party utilities.
Tari Ibaba (😎)
You can clearly see those particular keywords that set this definition apart from others
1. “…single statement…”
Single line or single statement? I go with the later.
Because the thing is, we squeeze every program ever made in a single line of code if we wanted; all the whitespace and file separation is only for us and our fellow developers.
If you’ve used Uglify.js or a similar minifier, you know what it does to all those pitiful lines of code; why it’s called Uglify.
Uglify changes this:
/**
Obviously redudant comments here. Just meant to
emphasize what Uglify does
Class Person
*/
class Person {
/**
Constructor
*/
constructor(name, age) {
this.name = name;
this.age = age;
}
/**
Print Message
*/
printMessage() {
console.log(`Hello! My name is ${this.name} and I am ${this.age} years old.`);
}
}
/**
Creating Object
*/
var person = new Person('John Doe', 25);
/**
Printing Message
*/
person.printMessage();
to this:
class Person{constructor(e,n){this.name=e,this.age=n}printMessage(){console.log(`Hello! My name is ${this.name} and I am ${this.age} years old.`)}}var person=new Person("John Doe",25);person.printMessage();
Would you be impressed by someone who actually wrote code like in this minified way? I would say it’s just badly formatted code.
Would you call this a one-liner?
const sum = (a, b) => { const s1 = a * a; const s2 = b * b; return s1 + s2; }
Tools like the VS Code Prettier extension will easily split up those 3 statements into multiple lines:
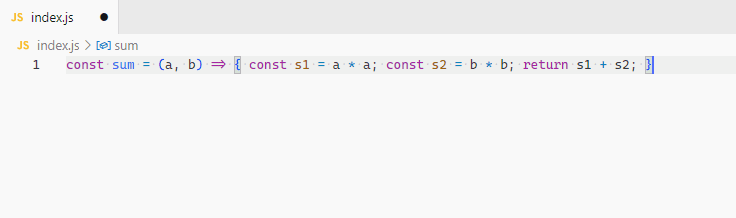
A true one-liner way to get the sum of two squares would be something like this:
const sum = (a, b) => a * a + b * b;
One short, succinct statement does the same job with equal clarity.
On the other hand, what about code that spans multiple lines but only uses one statement? Like this:
const capitalizeWithoutSpaces = (str) =>
str
.split('')
.filter((char) => char.trim())
.map((char) => char.toUpperCase())
.join('');
I would say this function’s body is far more qualified to be a one-line than the two examples we saw above; single statement.
“…particular programming language”
We need this part because of abstraction.
int sum(int a, int b) {
return a * a + b * b;
}
This is a one-liner, isn’t it? Very harmless-looking and easy to understand.
How about now? :
sum(int, int):
push rbp
mov rbp, rsp
mov DWORD PTR [rbp-4], edi
mov DWORD PTR [rbp-8], esi
mov eax, DWORD PTR [rbp-4]
imul eax, eax
mov edx, eax
mov eax, DWORD PTR [rbp-8]
imul eax, eax
add eax, edx
pop rbp
ret
Wait, this is just another piece of code, right? Well, it is, except that they do exactly the same thing, but in different languages. One in C++, and the other in Assembly.
Now imagine how much more an equivalent machine language program will have. Clearly, we can only our sum()
a one-liner function in the context of C++.
“…using only first-party utilities”
Once again, we need this part because of abstraction.
For us to consider a piece of code as a one-liner, it should only use built-in functions and methods that are part of the language’s standard library or core functionality. For example: array methods, the http
module in Node.js, the os
module in Python, and so on.
Without this, capitalizeWithoutSpaces()
below would easily pass as a JavaScript one-liner:
// Not a one-liner
const capitalizeWithoutSpaces = (str) =>
filter(str.split(''), (char) => char.trim())
.map((char) => char.toUpperCase())
.join('');
function filter(arr, callback) {
// Look at all these lines
const result = [];
for (const item of arr) {
if (callback(item)) {
result.push(item);
}
}
return result;
}
filter
could have contained many thousands of lines, yet capitalizeWithoutSpaces
would still be given one-liner status.
It’s kind of controversial because a lot of these so-called first-party utilities are abstractions themselves with logic spanning dozens of lines. But just like the “single statement” specifier, it makes it impossible to have an unlimited number of one-liners.
Final thoughts
The essence of a one-liner in programming extends beyond the literal interpretation of its name. It lies not only in the minimalism of physical lines but also in the elegance and sophistication of its execution. It often requires a sound comprehension of the language at hand, an ability to concisely solve a problem, and the art of utilizing the language’s core functionalities with finesse.
A one-liner isn’t merely about squeezing code into a single line; It is where the clarity of thought, the elegance of language mastery, and the succinctness of execution converge. It’s the realm where brevity meets brilliance and the art of coding truly shines.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
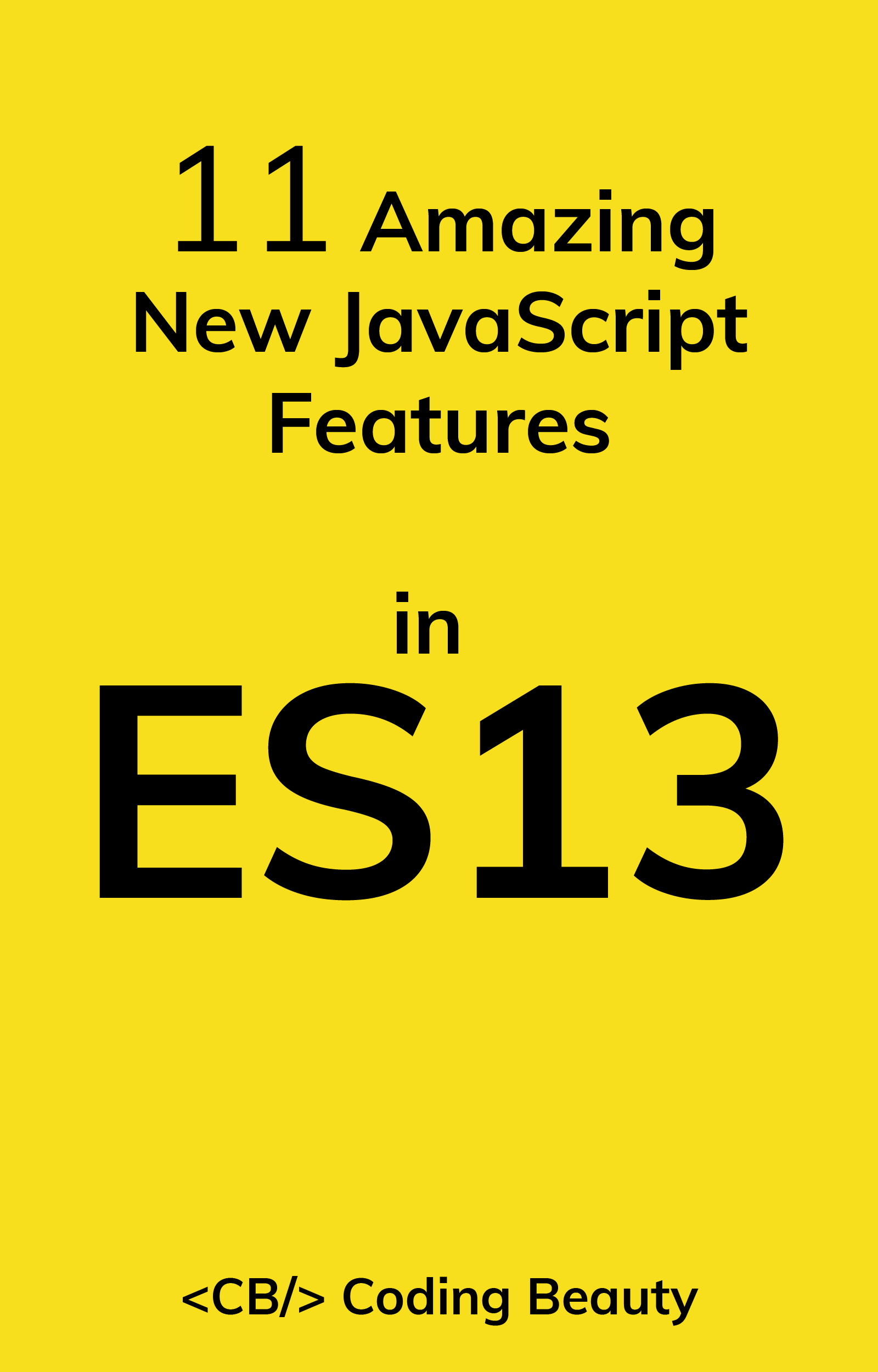