Here’s what we’ll be creating today:
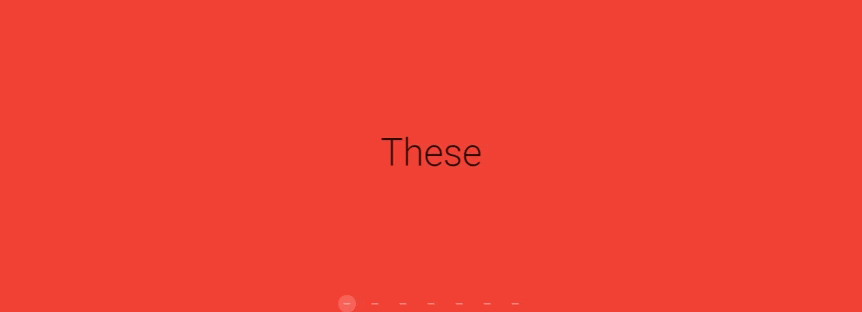
You’ll see how easy it is to build something like this, with the awesome power of Vuetify.
Just getting started with Vuetify?
Displaying the Colors
To start, we’ll display the colors. We’ll create a colors
variable, initialized to an array of all the colors of the rainbow — red, orange, yellow, green, blue, indigo, and violet.
To create the carousel we’ll use the v-carousel
component. We’ll use v-carousel-item
to add a slide to the carousel. Using the v-for
directive we loop through each color in colors
and add an item to the carousel to show it. We’ll do this by creating a v-sheet
component and setting its color
property.
src/App.vue
<template>
<v-app>
<v-carousel>
<v-carousel-item v-for="color in colors" :key="color">
<v-sheet :color="color" height="100%" tile>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
carousel: 0,
colors: ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', '#7f00ff'],
}),
};
</script>
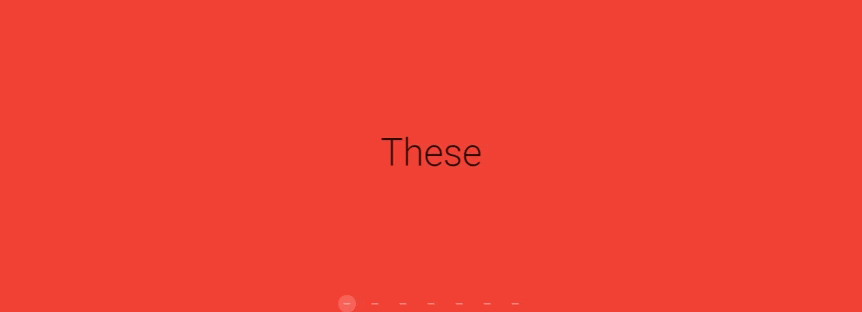
Showing the words
Now let’s display the sentence. It’s a 7-word sentence, so each word is displayed in one slide. We’ll position the word at the vertical and horizontal centre of the slide using v-row
. With the JavaScript string split()
method, we’ll divide the sentence into an array of the seven words.
src/App.vue
<template>
<v-app>
<v-carousel>
<v-carousel-item v-for="(color, i) in colors" :key="color">
<v-sheet :color="color" height="100%" tile>
<v-row class="fill-height" align="center" justify="center">
<div class="text-h2">
{{ words[i] }}
</div>
</v-row>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
carousel: 0,
colors: ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', '#7f00ff'],
words: 'These are the colours of the rainbow'.split(' '),
}),
};
</script>
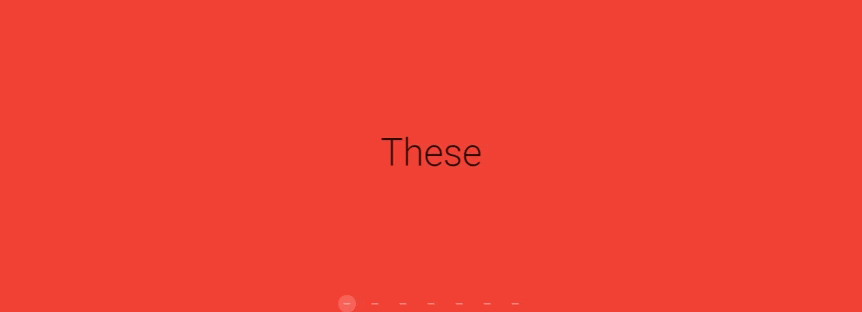
Changing the Delimiter Icon
Look at the icons that indicate each slide. They’re round. Let’s change them to short rectangles each, by changing the delimiter-icon
property of the carousel to mdi-minus
:
src/App.vue
<template>
<v-app>
<v-carousel delimiter-icon="mdi-minus">
<v-carousel-item v-for="(color, i) in colors" :key="color">
<v-sheet :color="color" height="100%" tile>
<v-row class="fill-height" align="center" justify="center">
<div class="text-h2">
{{ words[i] }}
</div>
</v-row>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
...
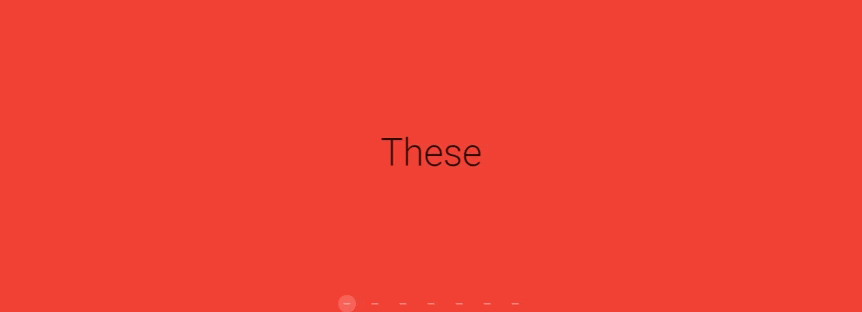
Displaying Arrows on Hover
Currently, the carousel arrows are always displayed. Let’s make them visible only when the user hovers over them with the mouse:
src/App.vue
<template>
<v-app>
<v-carousel delimiter-icon="mdi-minus" show-arrows-on-hover>
<v-carousel-item v-for="(color, i) in colors" :key="color">
<v-sheet :color="color" height="100%" tile>
<v-row class="fill-height" align="center" justify="center">
<div class="text-h2">
{{ words[i] }}
</div>
</v-row>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
...
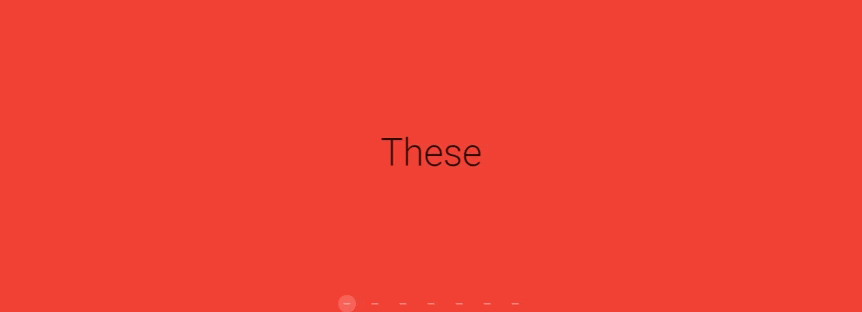
Hiding the delimiter background
You can see there’s a grey rectangle at the bottom of the carousel. This is the background of the delimiters. We don’t want this rectangle to be visible so let’s set the hide-delimiter-background
property to hide it:
src/App.vue
<template>
<v-app>
<v-carousel
delimiter-icon="mdi-minus"
show-arrows-on-hover
hide-delimiter-background
>
<v-carousel-item v-for="(color, i) in colors" :key="color">
<v-sheet :color="color" height="100%" tile>
<v-row class="fill-height" align="center" justify="center">
<div class="text-h2">
{{ words[i] }}
</div>
</v-row>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
...
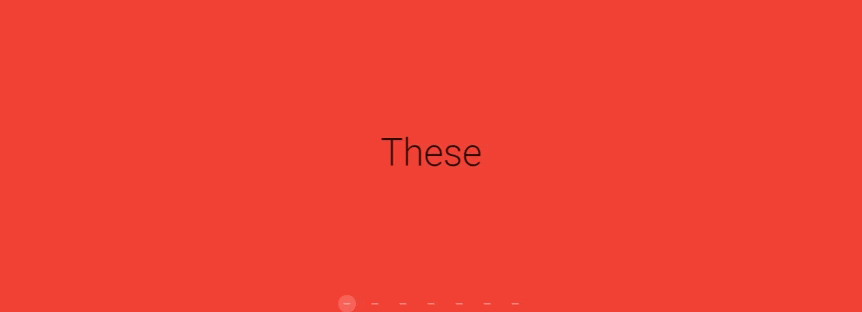
Setting Automatic Transitions
To make the carousel automatically transition to the next slide without having to click the right arrow, let’s set the cycle
property. The interval
determines the amount of time the carousel remains on one of its items before moving on to the next. Here we set an interval of one second.
src/App.vue
<template>
<v-app>
<v-carousel
delimiter-icon="mdi-minus"
show-arrows-on-hover
hide-delimiter-background
cycle
interval="1000"
>
<v-carousel-item v-for="(color, i) in colors" :key="color">
<v-sheet :color="color" height="100%" tile>
<v-row class="fill-height" align="center" justify="center">
<div class="text-h2">
{{ words[i] }}
</div>
</v-row>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
...
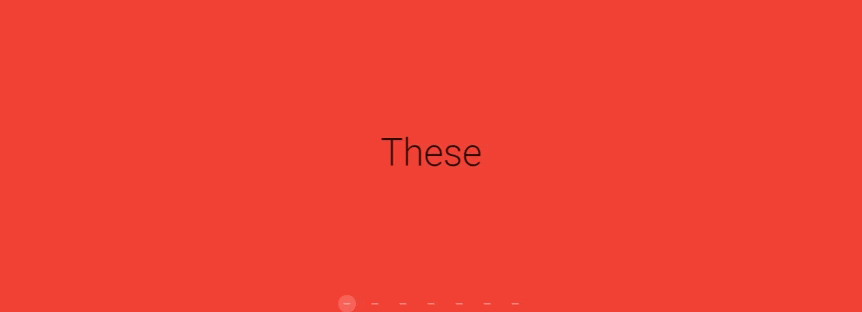
Changing the Transition
We can also change the animation the carousel uses when it wants to switch out the item it is currently displaying. By default, the current item slides out to the left as the next one simultaneously slides in. Let us make the current item fade out instead, while the incoming one fades in. This is the fade-transition
we set to the transition property of the v-carousel-item
. We’ll also do the same for the reverse transition — the current slide will fade out as the previous one fades in.
<template>
<v-app>
<v-carousel
delimiter-icon="mdi-minus"
show-arrows-on-hover
hide-delimiter-background
cycle
interval="1000"
>
<v-carousel-item
v-for="(color, i) in colors"
:key="color"
transition="fade-transition"
reverse-transition="fade-transition"
>
<v-sheet :color="color" height="100%" tile>
<v-row class="fill-height" align="center" justify="center">
<div class="text-h2">
{{ words[i] }}
</div>
</v-row>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-app>
</template>
...
And just like that, we’ve made ourselves a nice rainbow carousel app with Vuetify!
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
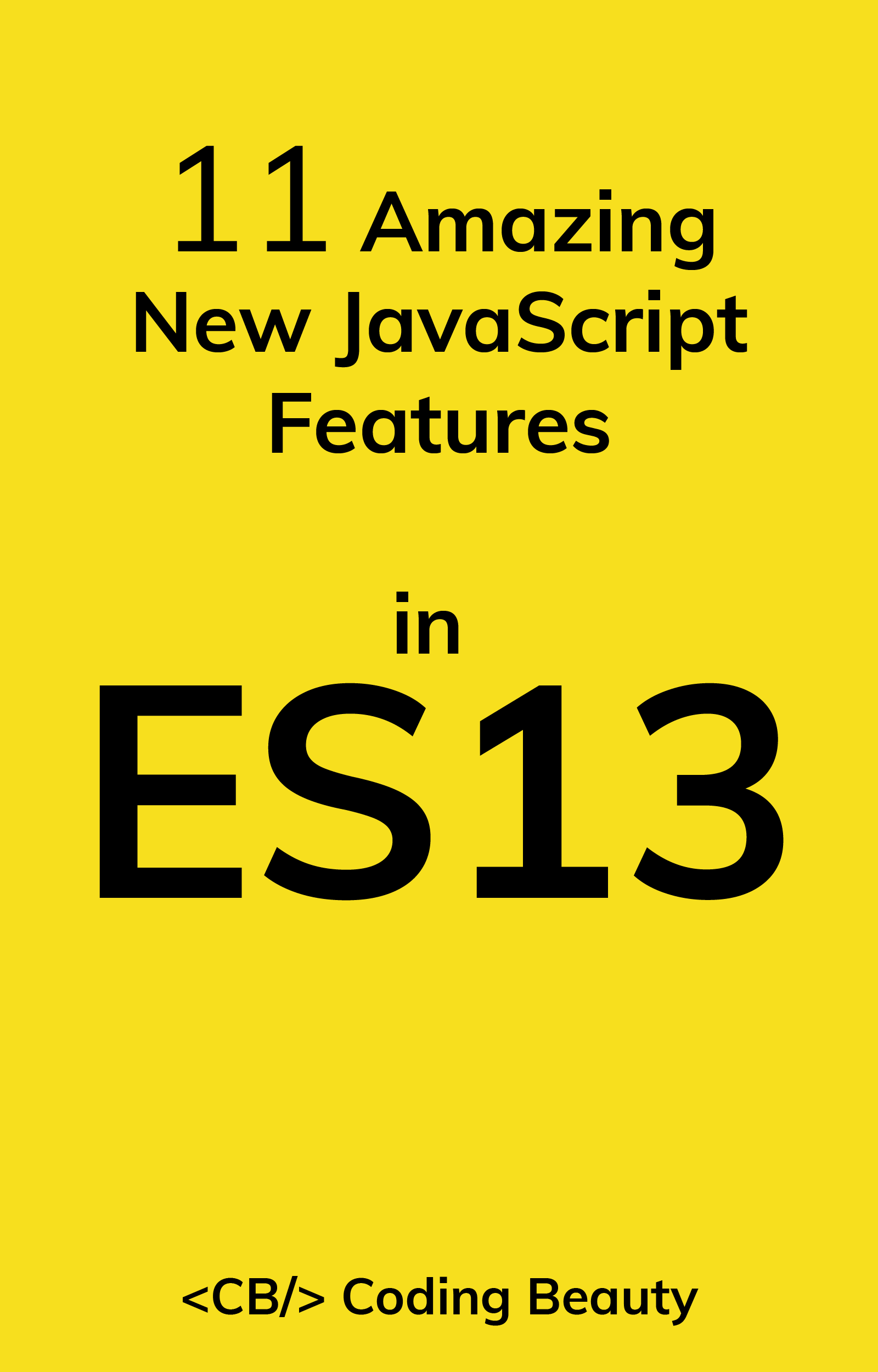