A circular progress bar is used to pass on information to the user about some ongoing operation. Just like horizontal progress bars, they visually signify how far the operation has advanced. Read on to learn about the Vuetify progress circular component and the various ways in which we can customize it.
The Vuetify Progress Circular Component
Vuetify provides the v-progress-circular component for creating
circular progress bars. We use the value
prop to set the progress bar value to a number between 0
and 100
inclusive.
<template>
<v-app>
<div class="d-flex justify-center ma-4">
<div class="d-flex justify-space-between" style="width: 60%">
<v-progress-circular></v-progress-circular>
<v-progress-circular :value="20"></v-progress-circular>
<v-progress-circular :value="40"></v-progress-circular>
<v-progress-circular :value="60"></v-progress-circular>
<v-progress-circular :value="80"></v-progress-circular>
<v-progress-circular :value="100"></v-progress-circular>
</div>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Circular Progress Bar Colors in Vuetify
The Vuetify progress circular component provides the color
prop for customizing the color of the circular progress bar.
<template>
<v-app>
<div class="d-flex justify-center ma-4">
<div class="d-flex justify-space-between" style="width: 60%">
<v-progress-circular :value="20" color="green"></v-progress-circular>
<v-progress-circular :value="40" color="primary"></v-progress-circular>
<v-progress-circular :value="60" color="red"></v-progress-circular>
<v-progress-circular
:value="80"
color="purple accent-4"
></v-progress-circular>
<v-progress-circular
:value="100"
color="yellow darken-3"
></v-progress-circular>
</div>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Indeterminate Circular Progress Bars in Vuetify
Similar to the Vuetify progress linear component, v-progress-circular
can be set to an indeterminate mode. It animates continuously when indeterminate:
<template>
<v-app>
<div class="d-flex justify-center ma-4">
<div class="d-flex justify-space-between" style="width: 60%">
<v-progress-circular indeterminate color="lime"></v-progress-circular>
<v-progress-circular indeterminate color="red"></v-progress-circular>
<v-progress-circular
indeterminate
color="primary"
></v-progress-circular>
<v-progress-circular
indeterminate
color="yellow darken-3"
></v-progress-circular>
<v-progress-circular
indeterminate
color="purple accent-4"
></v-progress-circular>
</div>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Vuetify Progress Circular Rotate
The rotate
prop allows us to customize the origin of the circular progress bar.
<template>
<v-app>
<div class="d-flex justify-center ma-4">
<div class="d-flex justify-space-between" style="width: 60%">
<v-progress-circular
:rotate="360"
:size="100"
:width="15"
:value="value"
color="purple accent-4"
>
{{ value }}
</v-progress-circular>
<v-progress-circular
:rotate="-90"
:size="100"
:width="15"
:value="value"
color="primary"
>
{{ value }}
</v-progress-circular>
<v-progress-circular
:rotate="90"
:size="100"
:width="15"
:value="value"
color="green"
>
{{ value }}
</v-progress-circular>
<v-progress-circular
:rotate="180"
:size="100"
:width="15"
:value="value"
color="red accent-2"
>
{{ value }}
</v-progress-circular>
</div>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data() {
return {
interval: {},
value: 0,
};
},
beforeDestroy() {
clearInterval(this.interval);
},
mounted() {
this.interval = setInterval(() => {
this.value = this.value === 100 ? 0 : this.value + 10;
}, 1000);
},
};
</script>

Vuetify Progress Circular Size and Thickness
The size
prop of v-progress-circular
allows us to modify the height of the circular progress bar, while the width
prop enables customization of the thickness of the progress bar.
<template>
<v-app>
<div class="d-flex justify-center ma-4">
<div class="d-flex justify-space-between align-center" style="width: 60%">
<v-progress-circular
:size="50"
color="red accent-2"
indeterminate
></v-progress-circular>
<v-progress-circular
:width="3"
color="primary"
indeterminate
></v-progress-circular>
<v-progress-circular
:size="70"
:width="9"
color="orange"
indeterminate
></v-progress-circular>
<v-progress-circular
:width="3"
color="green"
indeterminate
></v-progress-circular>
<v-progress-circular
:size="50"
color="purple accent-4"
indeterminate
></v-progress-circular>
</div>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data() {
return {
interval: {},
value: 0,
};
},
beforeDestroy() {
clearInterval(this.interval);
},
mounted() {
this.interval = setInterval(() => {
this.value = this.value === 100 ? 0 : this.value + 10;
}, 1000);
},
};
</script>

Conclusion
A circular progress bar conveys information to the user about the current progress on an ongoing process in an application. Use the Vuetify progress circular component (v-progress-circular
) to create and customize circular progress bars.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
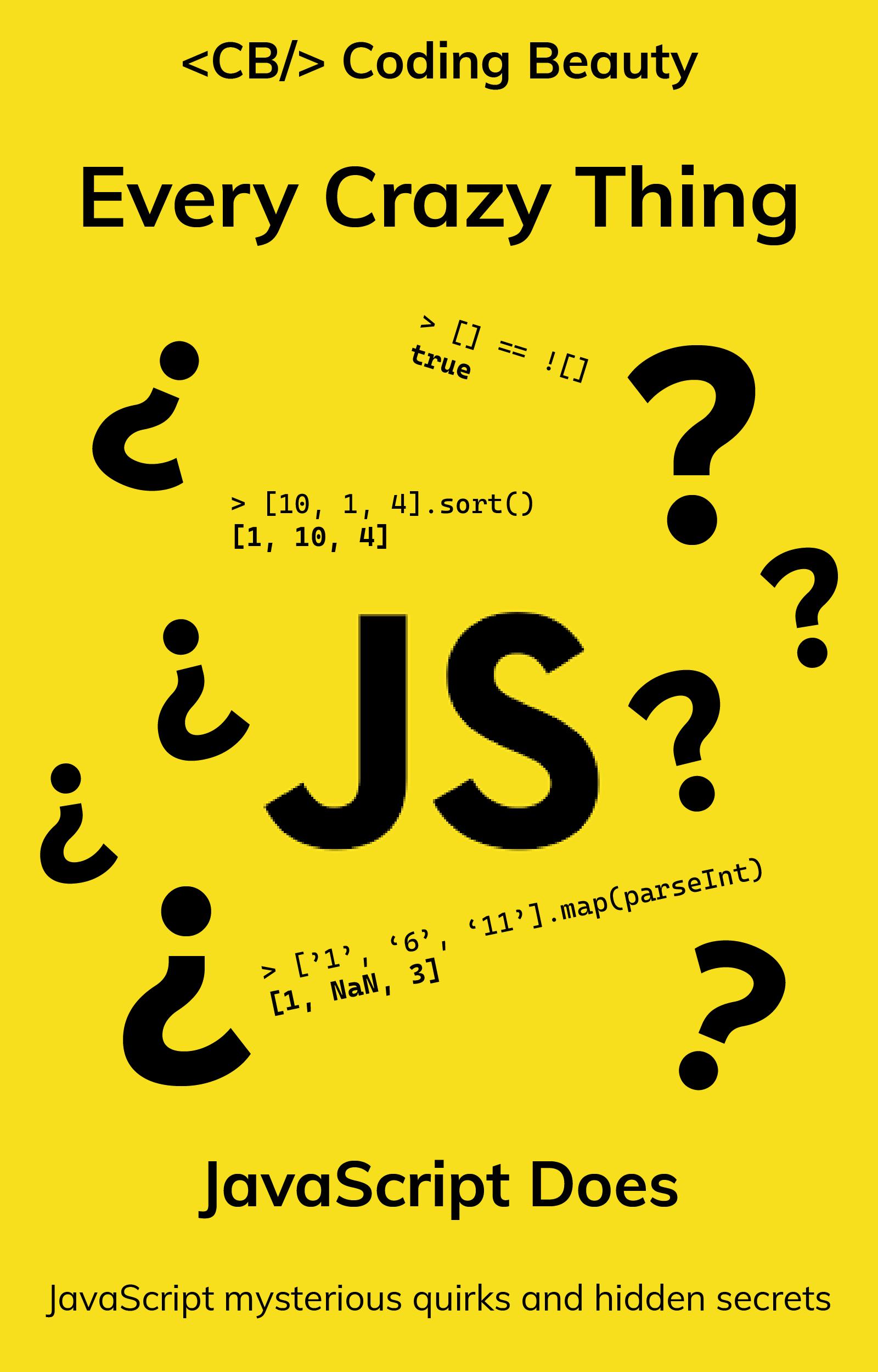