A flexbox layout simplifies the process of designing a flexible and responsive page layout structure without using float
or manual positioning. It allows responsive elements within a container to be automatically arranged depending on the size of the viewport. In this article, we’re going to learn about the various helper classes Vuetify provides to control the layout of flex containers with alignment, justification, wrapping, and more.
Vuetify Flexbox Container
Applying the d-flex
helper class to an element sets its display
to flex
, which turns it into a flexbox container transforming its direct children into flex items. As we’ll see later on, we can customize the interaction of these child elements with additional flex property utilities.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex pa-2"
outlined
tile
>
<div>A flexbox container</div>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Inline Flex
The d-inline-flex
class turns an element into an inline flexbox container.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-inline-flex pa-2"
outlined
tile
>
<div>Inline flexbox container</div>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

We can also customize the flex utilities to apply only for specific breakpoints with the following classes:
d-sm-flex
d-sm-inline-flex
d-md-flex
d-md-inline-flex
d-lg-flex
d-lg-inline-flex
d-xl-flex
d-xl-inline-flex
Vuetify Flex Direction
Vuetify provides flex helper classes for specifying the direction of the flex items within the container. These classes modify the flex-direction
CSS property of the element. They are:
- flex-row
- flex-row-reverse
- flex-column
- flex-column-reverse
flex-row
and flex-row-reverse
The flex-row
utility class displays the flexible items horizontally, as a row. flex-row-reverse
does the same, but in reverse order:
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex flex-row mb-6"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
Flex item {{ n }}
</v-card>
</v-card>
<v-card
class="d-flex flex-row-reverse"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
Flex item {{ n }}
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

flex-column
and flex-column-reverse
The flex-column
utility class displays the flex items vertically, in a column. flex-column-reverse
does the same, but in reverse order:
<template>
<v-app>
<div class="ma-4">
<div class="d-flex flex-column mb-6">
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
Flex item {{ n }}
</v-card>
</div>
<div class="d-flex flex-column-reverse">
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
Flex item {{ n }}
</v-card>
</div>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
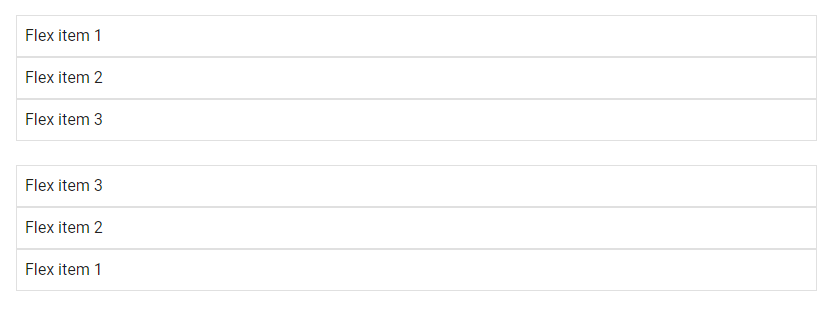
There are also responsive variations of these classes that set the flex-direction
property for certain breakpoints.
flex-sm-row
flex-sm-row-reverse
flex-sm-column
flex-sm-column-reverse
flex-md-row
flex-md-row-reverse
flex-md-column
flex-md-column-reverse
flex-lg-row
flex-lg-row-reverse
flex-lg-column
flex-lg-column-reverse
flex-xl-row
flex-xl-row-reverse
flex-xl-column
flex-xl-column-reverse
Flex Justify Classes
We can modify the justify-content
flex property of the flexible container with the flex justify classes from Vuetify. justify-content modifies the flexbox items on the x-axis or y-axis for a flex-direction
of row
or column
respectively. The helper classes can set justify-content
to start
(browser default), end
, center
, space-between
, or space-around
. They are:
justify-start
justify-end
justify-center
justify-space-between
justify-space-around
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex justify-start mb-6"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
justify-start
</v-card>
</v-card>
<v-card
class="d-flex justify-end mb-6"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
justify-end
</v-card>
</v-card>
<v-card
class="d-flex justify-center mb-6"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
justify-center
</v-card>
</v-card>
<v-card
class="d-flex justify-space-between mb-6"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
justify-space-between
</v-card>
</v-card>
<v-card
class="d-flex justify-space-around mb-6"
color="grey lighten-4"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
justify-space-around
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
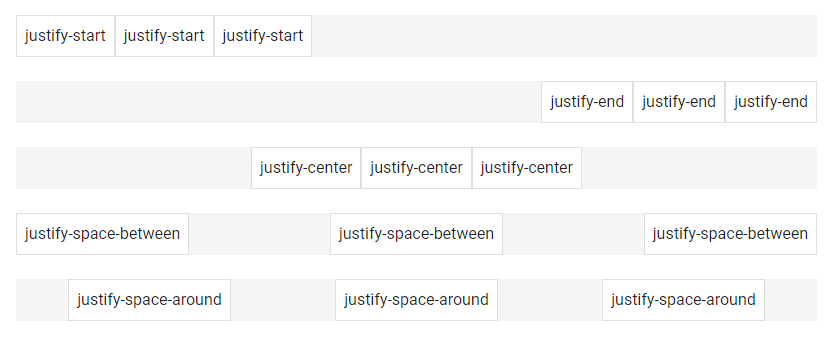
There are also responsive variations of these classes that set the justify-content
property for certain breakpoints:
justify-sm-start
justify-sm-end
justify-sm-center
justify-sm-space-between
justify-sm-space-around
justify-md-start
justify-md-end
justify-md-center
justify-md-space-between
justify-md-space-around
justify-lg-start
justify-lg-end
justify-lg-center
justify-lg-space-between
justify-lg-space-around
justify-xl-start
justify-xl-end
justify-xl-center
justify-xl-space-between
justify-xl-space-around
Vuetify Flex Align Classes
We also have flex utility classes from Vuetify that modify the align-items
CSS property of the flex container. align-items
modifies the flexbox items on the y-axis or x-axis for a flex-direction of row
or column
respectively. The helper classes can set align-items
to start
, end
, center
, baseline
or stretch
(browser default). They are:
align-start
align-end
align-center
align-baseline
align-stretch
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-start mb-6"
color="grey lighten-2"
flat
height="100"
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
align-start
</v-card>
</v-card>
<v-card
class="d-flex align-end mb-6"
color="grey lighten-2"
flat
height="100"
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
align-end
</v-card>
</v-card>
<v-card
class="d-flex align-center mb-6"
color="grey lighten-2"
flat
height="100"
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
align-center
</v-card>
</v-card>
<v-card
class="d-flex align-baseline mb-6"
color="grey lighten-2"
flat
height="100"
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
align-baseline
</v-card>
</v-card>
<v-card
class="d-flex align-stretch mb-6"
color="grey lighten-2"
flat
height="100"
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
align-stretch
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
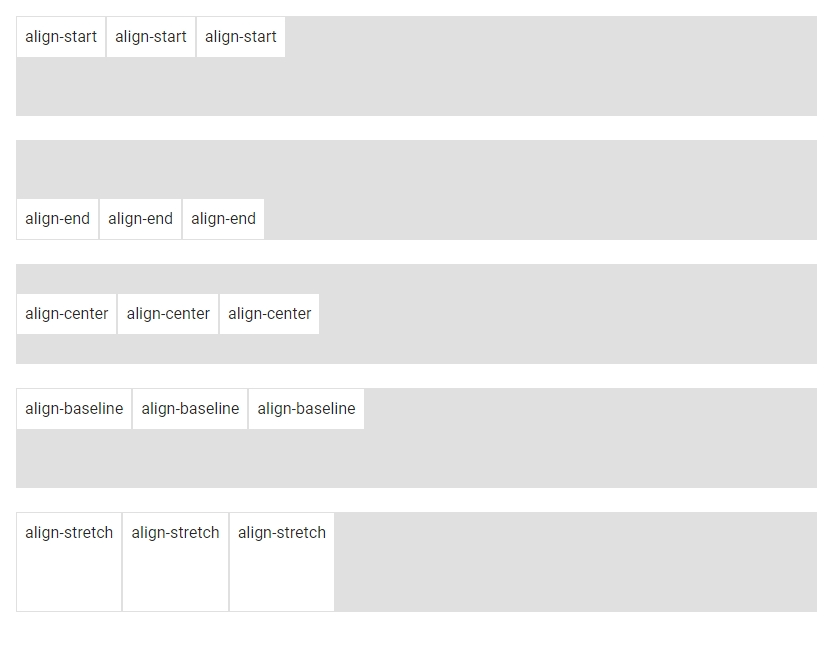
They are also responsive variations of these classes that set the align-items
property for certain breakpoints:
align-sm-start
align-sm-end
align-sm-center
align-sm-baseline
align-sm-stretch
align-md-start
align-md-end
align-md-center
align-md-baseline
align-md-stretch
align-lg-start
align-lg-end
align-lg-center
align-lg-baseline
align-lg-stretch
align-xl-start
align-xl-end
align-xl-center
align-xl-baseline
align-xl-stretch
Flex Align Self Classes
We use the flex align self helper classes to modify the align-self
flex property of the element. align-self modifies the flexbox items on the x-axis
or y-axis
for a flex-direction
of row
or column
respectively. The helper classes can set align-self
to start
, end
, center
, baseline
or stretch
(browser default). They are:
align-self-start
align-self-end
align-self-center
align-self-baseline
align-self-stretch
<template>
<v-app>
<div class="ma-4">
<v-card
v-for="j in justify"
:key="j"
class="d-flex mb-6"
color="grey lighten-2"
flat
height="100"
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
:class="[n === 2 && `align-self-${j}`]"
outlined
tile
>
{{ n === 2 ? 'Aligned flex item' : 'Flex item' }}
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
justify: [
'start',
'end',
'center',
'baseline',
'auto',
'stretch',
],
}),
};
</script>
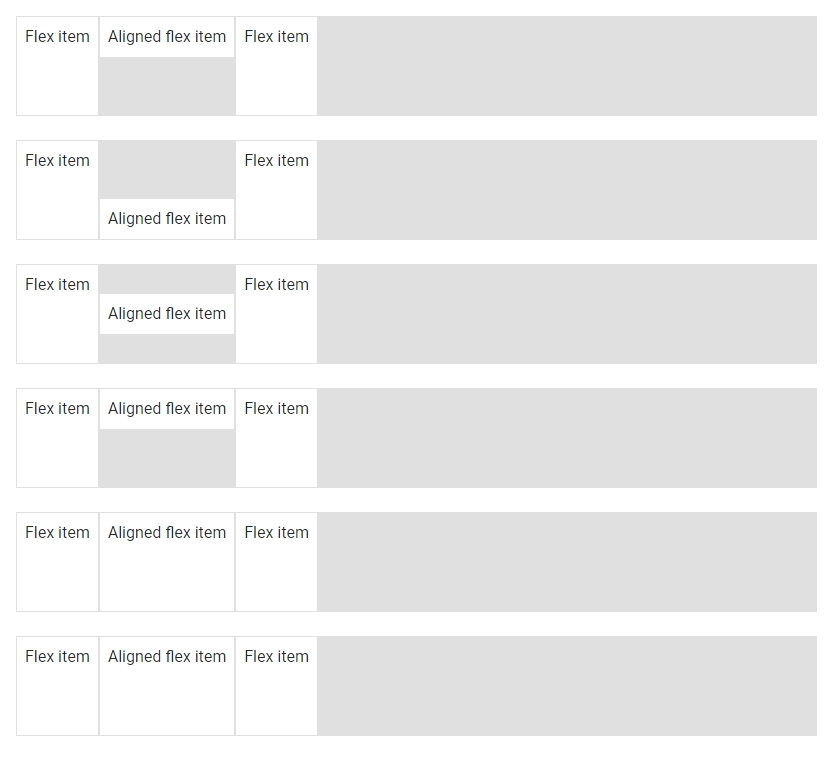
Vuetify also provides responsive variations of these classes that set the align-self
property for certain breakpoints:
align-self-sm-start
align-self-sm-end
align-self-sm-center
align-self-sm-baseline
align-self-sm-auto
align-self-sm-stretch
align-self-md-start
align-self-md-end
align-self-md-center
align-self-md-baseline
align-self-md-auto
align-self-md-stretch
align-self-lg-start
align-self-lg-end
align-self-lg-center
align-self-lg-baseline
align-self-lg-auto
align-self-lg-stretch
align-self-xl-start
align-self-xl-end
align-self-xl-center
align-self-xl-baseline
align-self-xl-auto
align-self-xl-stretch
Auto Margins
We can apply one of the margin helper classes from Vuetify to a flex container to control the positioning of flex items on the x-axis or y-axis for a flex direction of row
or column
respectively.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex mb-6"
color="grey lighten-2"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
<v-card
class="d-flex mb-6"
color="grey lighten-2"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
:class="n === 1 && 'mr-auto'"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
<v-card
class="d-flex mb-6"
color="grey lighten-2"
flat
tile
>
<v-card
v-for="n in 3"
:key="n"
:class="n === 3 && 'ml-auto'"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
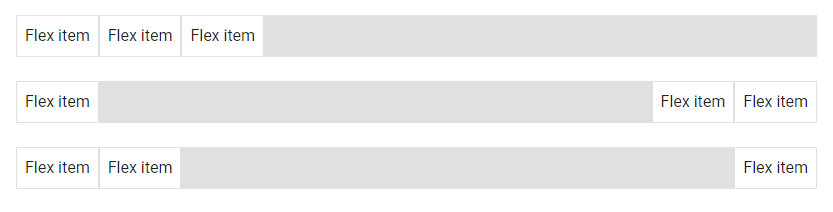
Using align-items
If we set flex-direction
to column
and specify a value for align-items
, we can use either the mb-auto
or mt-auto
helper class to adjust flex item positioning:
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-start flex-column mb-6"
color="grey lighten-2"
flat
tile
height="200"
>
<v-card
v-for="n in 3"
:key="n"
:class="n === 1 && 'mb-auto'"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
<v-card
class="d-flex align-end flex-column"
color="grey lighten-2"
flat
tile
height="200"
>
<v-card
v-for="n in 3"
:key="n"
:class="n === 3 && 'mt-auto'"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
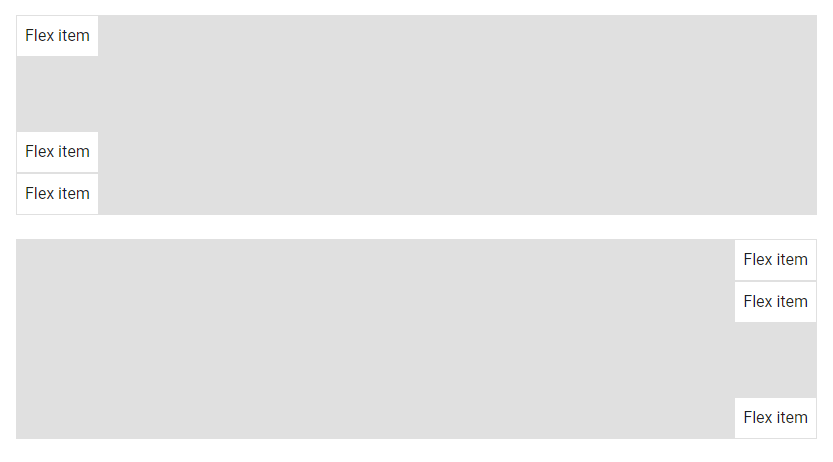
Vuetify Flex Wrap Classes
By default, the d-flex
class does not provide wrapping (it behaves similar to setting flex-wrap
to nowrap
). We can change this by applying one of the flex-wrap helper classes to the container. The classes are:
flex-nowrap
flex-wrap
flex-wrap-reverse
flex-nowrap
flex-nowrap
specifies that the items should not wrap.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex flex-nowrap py-3"
color="grey lighten-2"
flat
tile
width="125"
>
<v-card
v-for="n in 5"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

flex-wrap
flex-wrap
makes the items wrap if necessary.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex flex-wrap"
color="grey lighten-2"
flat
tile
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item {{ n }}
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
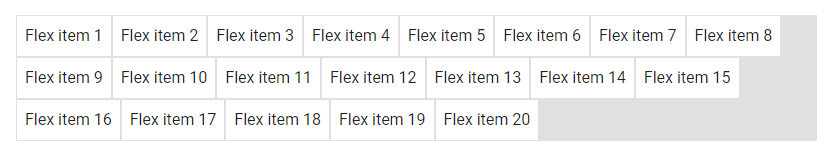
flex-wrap-reverse
flex-wrap-reverse
specifies that the flex items will wrap if necessary, in reverse order.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex flex-wrap-reverse"
color="grey lighten-2"
flat
tile
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item {{ n }}
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
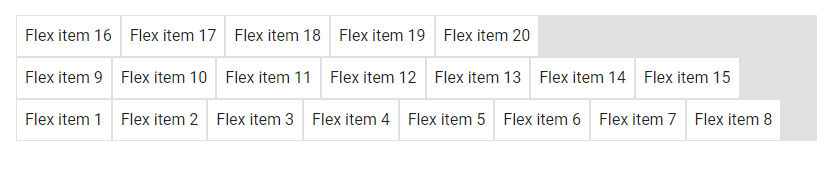
Vuetify also provides responsive variations of these classes to set the flex-wrap
property for certain breakpoints:
flex-sm-nowrap
flex-sm-wrap
flex-sm-wrap-reverse
flex-md-nowrap
flex-md-wrap
flex-md-wrap-reverse
flex-lg-nowrap
flex-lg-wrap
flex-lg-wrap-reverse
flex-xl-nowrap
flex-xl-wrap
flex-xl-wrap-reverse
Vuetify Flex Order Classes
We can use one of the flex order utility classes to customize the visual order of the flexbox items within their container.
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex flex-wrap"
color="grey lighten-2"
flat
tile
>
<v-card
class="order-2 pa-2"
outlined
tile
>
First flex item
</v-card>
<v-card
class="order-3 pa-2"
outlined
tile
>
Second flex item
</v-card>
<v-card
class="order-1 pa-2"
outlined
tile
>
Third flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

These classes set the order
flex property of the container. They are:
order-first
order-0
order-1
order-2
order-3
order-4
order-5
order-6
order-7
order-8
order-9
order-10
order-11
order-12
order-last
Vuetify also provides responsive variants of these classes that set the order
property for certain breakpoints:
order-sm-first
order-sm-0
order-sm-1
order-sm-2
order-sm-3
order-sm-4
order-sm-5
order-sm-6
order-sm-7
order-sm-8
order-sm-9
order-sm-10
order-sm-11
order-sm-12
order-sm-last
order-md-first
order-md-0
order-md-1
order-md-2
order-md-3
order-md-4
order-md-5
order-md-6
order-md-7
order-md-8
order-md-9
order-md-10
order-md-11
order-md-12
order-md-last
order-lg-first
order-lg-0
order-lg-1
order-lg-2
order-lg-3
order-lg-4
order-lg-5
order-lg-6
order-lg-7
order-lg-8
order-lg-9
order-lg-10
order-lg-11
order-lg-12
order-lg-last
- order-xl-first
order-xl-0
order-xl-1
order-xl-2
order-xl-3
order-xl-4
order-xl-5
order-xl-6
order-xl-7
order-xl-8
order-xl-9
order-xl-10
order-xl-11
order-xl-12
order-xl-last
Vuetify Flex Align Content
Vuetify provides flex align content classes that we can use to set the align-content
CSS property of the flex container. The align-content
modifies the flexbox items on the x-axis or y-axis for a flex-direction of row
or column
respectively. The helper classes can set align-content
to start
(browser default), end
, center
, between
, around
or stretch
. They are:
align-content-start
align-content-end
align-content-center
align-content-between
align-content-around
align-content-stretch
align-content-start
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-content-start flex-wrap"
color="grey lighten-2"
flat
tile
min-height="200"
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
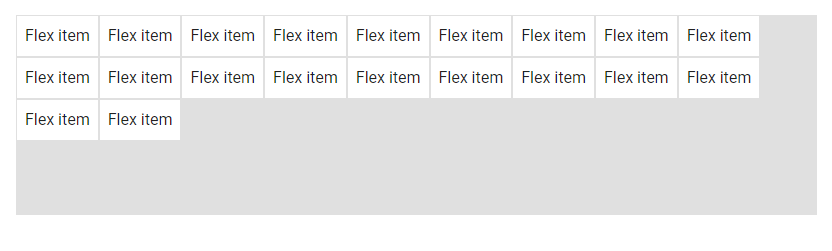
align-content-end
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-content-end flex-wrap"
color="grey lighten-2"
flat
tile
min-height="200"
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
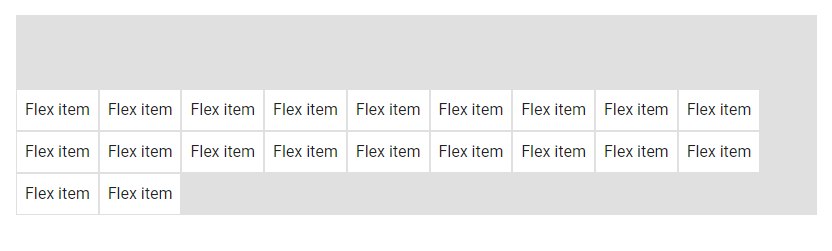
align-content-center
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-content-center flex-wrap"
color="grey lighten-2"
flat
tile
min-height="200"
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
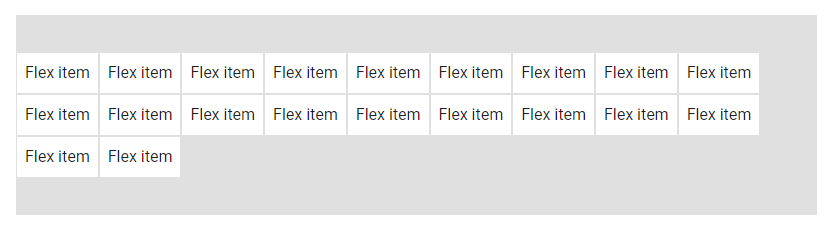
align-content-space-between
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-content-space-between flex-wrap"
color="grey lighten-2"
flat
tile
min-height="200"
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
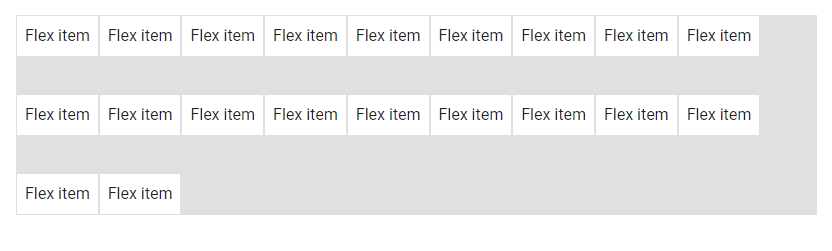
align-content-space-around
<template>
<v-app>
<div class="ma-4">
<v-card
class="d-flex align-content-space-around flex-wrap"
color="grey lighten-2"
flat
tile
min-height="200"
>
<v-card
v-for="n in 20"
:key="n"
class="pa-2"
outlined
tile
>
Flex item
</v-card>
</v-card>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
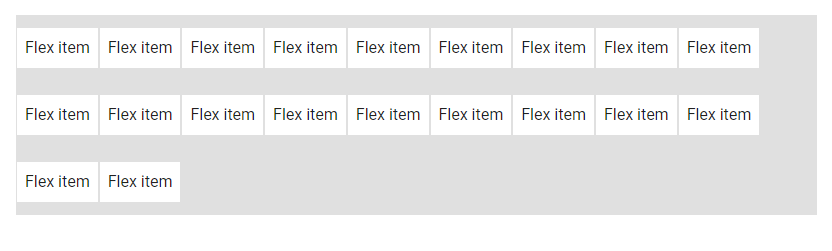
There are also responsive variations of theses classes that set the align-content
property for certain breakpoints:
align-sm-content-start
align-sm-content-end
align-sm-content-center
align-sm-content-space-between
align-sm-content-space-around
align-sm-content-stretch
align-md-content-start
align-md-content-end
align-md-content-center
align-md-content-space-between
align-md-content-space-around
align-md-content-stretch
align-lg-content-start
align-lg-content-end
align-lg-content-center
align-lg-content-space-between
align-lg-content-space-around
align-xl-content-start
align-xl-content-end
align-xl-content-center
align-xl-content-space-between
align-xl-content-space-around
align-xl-content-stretch
Vuetify Flex Grow and Shrink Classes
Vuetify also comes with helper classes that set the flex-grow
and flex-shrink
of a flexbox container. These utility classes are in the form flex-{condition}-{value}
, where condition
can be either grow
or shrink
and value
can be either 0
or 1
. The grow
condition will allow an element to grow to fill available space, while shrink
will allow an element to shrink down only to the space needed for its contents if there is not enough space in its container. The value 0
will prevent the condition from happening, while 1
will allow it. The classes are:
flex-grow-0
flex-grow-1
flex-shrink-0
flex-shrink-1
<template>
<v-app>
<div class="ma-4">
<v-container class="grey lighten-5">
<v-row
no-gutters
style="flex-wrap: nowrap"
>
<v-col
cols="2"
class="flex-grow-0 flex-shrink-0"
>
<v-card
class="pa-2"
outlined
tile
>
I'm 2 column wide
</v-card>
</v-col>
<v-col
cols="1"
style="min-width: 100px; max-width: 100%"
class="flex-grow-1 flex-shrink-0"
>
<v-card
class="pa-2"
outlined
tile
>
I'm 1 column wide and I grow to take all the
space
</v-card>
</v-col>
<v-col
cols="5"
style="min-width: 100px"
class="flex-grow-0 flex-shrink-1"
>
<v-card
class="pa-2"
outlined
tile
>
I'm 5 column wide and I shrink if there's not
enough space
</v-card>
</v-col>
</v-row>
</v-container>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

There are also responsive variations of these classes that set the flex-grow
and flex-shrink
properties for certain breakpoints:
flex-sm-grow-0
flex-sm-grow-1
flex-sm-shrink-0
flex-sm-shrink-1
flex-md-grow-0
flex-md-grow-1
flex-md-shrink-0
flex-md-shrink-1
flex-lg-grow-0
flex-lg-grow-1
flex-lg-shrink-0
flex-lg-shrink-1
flex-xl-grow-0
flex-xl-grow-1
flex-xl-shrink-0
flex-xl-shrink-1
Conclusion
Flexbox layouts allow responsive items inside a container to be automatically arranged depending on the screen size. Vuetify comes with various flexbox utility classes for controlling the layout of flex containers with alignment, justification, wrapping, and more.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
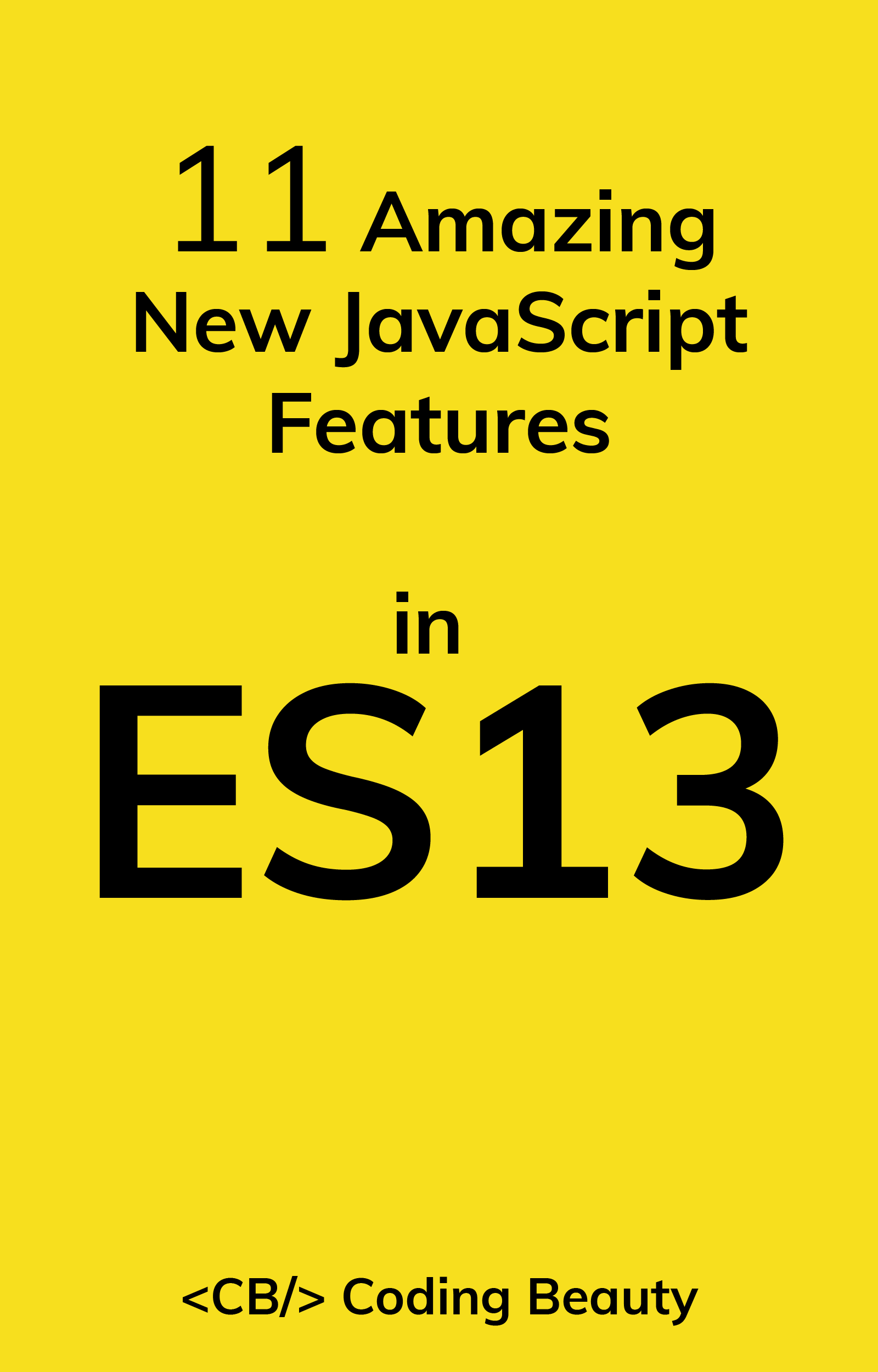