A table is a great way of presenting structured information in an easily understandable format. In this post, we’re going to learn how to use the Vuetify data table component, which is used for displaying tabular data and comes with useful features such as sorting, searching, pagination, and more.
The Vuetify Data Table Component (v-data-table
)
Vuetify provides the v-data-table
component for creating data tables. The headers
prop of this component is an array of objects used to configure the data table headers. Each object in this array has a text
property, which determines the header name that shows up for a particular column. We also pass an array of objects (desserts
) to the items
prop. Each object in desserts
corresponds to a row in the data table, and each property represents a table cell for that row. The property names of these objects have to match one of the value
property values of the objects in the headers
array, i.e. (a key name must be one of name
, calories
, fat
, carbs
, protein
, and iron
).
We use the items-per-page
property to paginate the data table. Here we set it to 5, so we have 2 pages for the 10 desserts.
<template>
<v-app>
<v-data-table
:headers="headers"
:items="desserts"
:items-per-page="5"
class="elevation-1"
></v-data-table>
</v-app>
</template>
<script>
export default {
data() {
return {
headers: [
{
text: 'Dessert (100g serving)',
align: 'start',
sortable: false,
value: 'name',
},
{ text: 'Calories', value: 'calories' },
{ text: 'Fat (g)', value: 'fat' },
{ text: 'Carbs (g)', value: 'carbs' },
{ text: 'Protein (g)', value: 'protein' },
{ text: 'Iron (%)', value: 'iron' },
],
desserts: [
{
name: 'Frozen Yogurt',
calories: 159,
fat: 6.0,
carbs: 24,
protein: 4.0,
iron: '1%',
},
{
name: 'Ice cream sandwich',
calories: 237,
fat: 9.0,
carbs: 37,
protein: 4.3,
iron: '1%',
},
{
name: 'Eclair',
calories: 262,
fat: 16.0,
carbs: 23,
protein: 6.0,
iron: '7%',
},
{
name: 'Cupcake',
calories: 305,
fat: 3.7,
carbs: 67,
protein: 4.3,
iron: '8%',
},
{
name: 'Gingerbread',
calories: 356,
fat: 16.0,
carbs: 49,
protein: 3.9,
iron: '16%',
},
{
name: 'Jelly bean',
calories: 375,
fat: 0.0,
carbs: 94,
protein: 0.0,
iron: '0%',
},
{
name: 'Lollipop',
calories: 392,
fat: 0.2,
carbs: 98,
protein: 0,
iron: '2%',
},
{
name: 'Honeycomb',
calories: 408,
fat: 3.2,
carbs: 87,
protein: 6.5,
iron: '45%',
},
{
name: 'Donut',
calories: 452,
fat: 25.0,
carbs: 51,
protein: 4.9,
iron: '22%',
},
{
name: 'KitKat',
calories: 518,
fat: 26.0,
carbs: 65,
protein: 7,
iron: '6%',
},
],
};
},
};
</script>
And here’s our table:
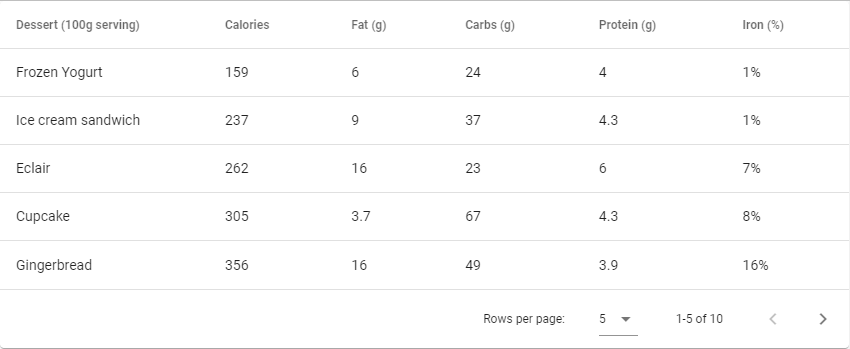
Vuetify Data Table Dense
Setting the dense
prop makes a data table more compact, as the name implies:
<template>
<v-app>
<v-data-table
dense
:headers="headers"
:items="desserts"
item-key="name"
class="elevation-1"
></v-data-table>
</v-app>
</template>
<script>
export default {
data: () => ({
desserts: [
{
name: 'Frozen Yogurt',
calories: 159,
fat: 6.0,
carbs: 24,
protein: 4.0,
iron: '1%',
},
{
name: 'Ice cream sandwich',
calories: 237,
fat: 9.0,
carbs: 37,
protein: 4.3,
iron: '1%',
},
{
name: 'Eclair',
calories: 262,
fat: 16.0,
carbs: 23,
protein: 6.0,
iron: '7%',
},
{
name: 'Cupcake',
calories: 305,
fat: 3.7,
carbs: 67,
protein: 4.3,
iron: '8%',
},
{
name: 'Gingerbread',
calories: 356,
fat: 16.0,
carbs: 49,
protein: 3.9,
iron: '16%',
},
{
name: 'Jelly bean',
calories: 375,
fat: 0.0,
carbs: 94,
protein: 0.0,
iron: '0%',
},
{
name: 'Lollipop',
calories: 392,
fat: 0.2,
carbs: 98,
protein: 0,
iron: '2%',
},
{
name: 'Honeycomb',
calories: 408,
fat: 3.2,
carbs: 87,
protein: 6.5,
iron: '45%',
},
{
name: 'Donut',
calories: 452,
fat: 25.0,
carbs: 51,
protein: 4.9,
iron: '22%',
},
{
name: 'KitKat',
calories: 518,
fat: 26.0,
carbs: 65,
protein: 7,
iron: '6%',
},
],
headers: [
{
text: 'Dessert (100g serving)',
align: 'start',
sortable: false,
value: 'name',
},
{ text: 'Calories', value: 'calories' },
{ text: 'Fat (g)', value: 'fat' },
{ text: 'Carbs (g)', value: 'carbs' },
{ text: 'Protein (g)', value: 'protein' },
{ text: 'Iron (%)', value: 'iron' },
],
}),
};
</script>
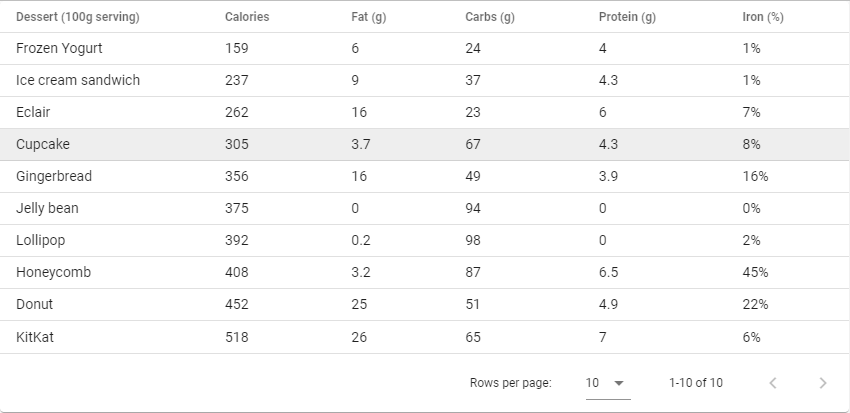
Vuetify Data Table Search Features
We can filter data on the data table with the search
prop. Here, we’re binding whatever value entered in the text field with the input used for searching the table:
<template>
<v-app>
<v-card>
<v-card-title>
Nutrition
<v-spacer></v-spacer>
<v-text-field
v-model="search"
append-icon="mdi-magnify"
label="Search"
single-line
hide-details
></v-text-field>
</v-card-title>
<v-data-table
:headers="headers"
:items="desserts"
:search="search"
:items-per-page="5"
></v-data-table>
</v-card>
</v-app>
</template>
<script>
export default {
data: () => ({
search: '',
desserts: [
{
name: 'Frozen Yogurt',
calories: 159,
fat: 6.0,
carbs: 24,
protein: 4.0,
iron: '1%',
},
{
name: 'Ice cream sandwich',
calories: 237,
fat: 9.0,
carbs: 37,
protein: 4.3,
iron: '1%',
},
{
name: 'Eclair',
calories: 262,
fat: 16.0,
carbs: 23,
protein: 6.0,
iron: '7%',
},
{
name: 'Cupcake',
calories: 305,
fat: 3.7,
carbs: 67,
protein: 4.3,
iron: '8%',
},
{
name: 'Gingerbread',
calories: 356,
fat: 16.0,
carbs: 49,
protein: 3.9,
iron: '16%',
},
{
name: 'Jelly bean',
calories: 375,
fat: 0.0,
carbs: 94,
protein: 0.0,
iron: '0%',
},
{
name: 'Lollipop',
calories: 392,
fat: 0.2,
carbs: 98,
protein: 0,
iron: '2%',
},
{
name: 'Honeycomb',
calories: 408,
fat: 3.2,
carbs: 87,
protein: 6.5,
iron: '45%',
},
{
name: 'Donut',
calories: 452,
fat: 25.0,
carbs: 51,
protein: 4.9,
iron: '22%',
},
{
name: 'KitKat',
calories: 518,
fat: 26.0,
carbs: 65,
protein: 7,
iron: '6%',
},
],
headers: [
{
text: 'Dessert (100g serving)',
align: 'start',
sortable: false,
value: 'name',
},
{ text: 'Calories', value: 'calories' },
{ text: 'Fat (g)', value: 'fat' },
{ text: 'Carbs (g)', value: 'carbs' },
{ text: 'Protein (g)', value: 'protein' },
{ text: 'Iron (%)', value: 'iron' },
],
}),
};
</script>
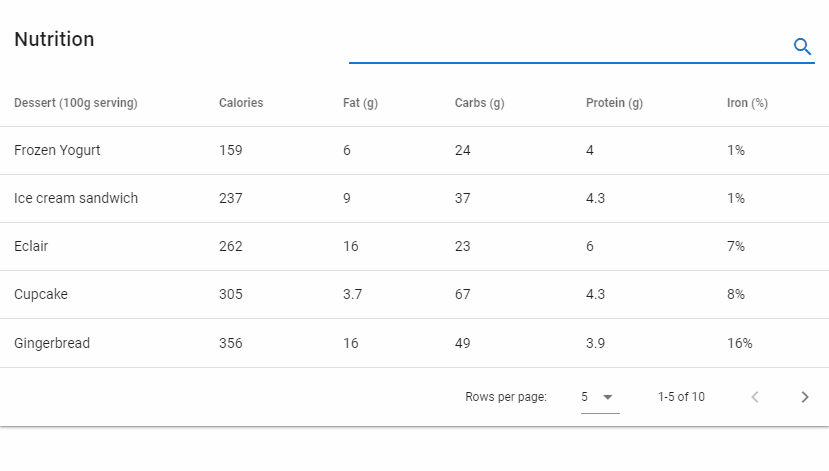
Vuetify Data Table Custom Filters
We can specify our custom filter function by passing a method to the custom-filter
prop that has the signature (value: any, search: string | null, item: any) => boolean
.
<template>
<v-app>
<div>
<v-data-table
:headers="headers"
:items="desserts"
item-key="name"
class="elevation-1"
:search="search"
:custom-filter="filterOnlyCapsText"
:items-per-page="5"
>
<template v-slot:top>
<v-text-field
v-model="search"
label="Search (UPPER CASE ONLY)"
class="mx-4"
></v-text-field>
</template>
</v-data-table>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data() {
return {
search: '',
calories: '',
desserts: [
{
name: 'Frozen Yogurt',
calories: 159,
fat: 6.0,
carbs: 24,
protein: 4.0,
iron: '1%',
},
{
name: 'Ice cream sandwich',
calories: 237,
fat: 9.0,
carbs: 37,
protein: 4.3,
iron: '1%',
},
{
name: 'Eclair',
calories: 262,
fat: 16.0,
carbs: 23,
protein: 6.0,
iron: '7%',
},
{
name: 'Cupcake',
calories: 305,
fat: 3.7,
carbs: 67,
protein: 4.3,
iron: '8%',
},
{
name: 'Gingerbread',
calories: 356,
fat: 16.0,
carbs: 49,
protein: 3.9,
iron: '16%',
},
{
name: 'Jelly bean',
calories: 375,
fat: 0.0,
carbs: 94,
protein: 0.0,
iron: '0%',
},
{
name: 'Lollipop',
calories: 392,
fat: 0.2,
carbs: 98,
protein: 0,
iron: '2%',
},
{
name: 'Honeycomb',
calories: 408,
fat: 3.2,
carbs: 87,
protein: 6.5,
iron: '45%',
},
{
name: 'Donut',
calories: 452,
fat: 25.0,
carbs: 51,
protein: 4.9,
iron: '22%',
},
{
name: 'KitKat',
calories: 518,
fat: 26.0,
carbs: 65,
protein: 7,
iron: '6%',
},
],
};
},
computed: {
headers() {
return [
{
text: 'Dessert (100g serving)',
align: 'start',
sortable: false,
value: 'name',
},
{
text: 'Calories',
value: 'calories',
},
{ text: 'Fat (g)', value: 'fat' },
{ text: 'Carbs (g)', value: 'carbs' },
{ text: 'Protein (g)', value: 'protein' },
{ text: 'Iron (%)', value: 'iron' },
];
},
},
methods: {
filterOnlyCapsText(value, search, item) {
return (
value != null &&
search != null &&
typeof value === 'string' &&
value.toString().toLocaleUpperCase().indexOf(search) !== -1
);
},
},
};
</script>
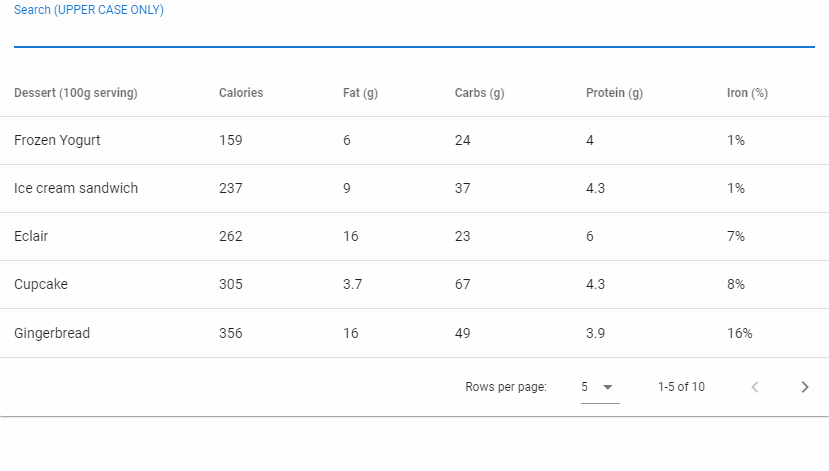
We can also customize the filtering of a specific column by supplying a function to the filter
property on an item in the array passed to the headers
prop. In the code example below, we add a text field below the rows of the data table (with the body.append
slot) which can be used to display all the desserts with calories less than a certain value.
<template>
<v-app>
<div>
<v-data-table
:headers="headers"
:items="desserts"
item-key="name"
class="elevation-1"
:search="search"
:custom-filter="filterOnlyCapsText"
:items-per-page="5"
>
<template v-slot:top>
<v-text-field
v-model="search"
label="Search (UPPER CASE ONLY)"
class="mx-4"
></v-text-field>
</template>
<template v-slot:body.append>
<tr>
<td></td>
<td>
<v-text-field
v-model="calories"
type="number"
label="Less than"
></v-text-field>
</td>
<td colspan="4"></td>
</tr>
</template>
</v-data-table>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data() {
return {
search: '',
calories: '',
desserts: [
{
name: 'Frozen Yogurt',
calories: 159,
fat: 6.0,
carbs: 24,
protein: 4.0,
iron: '1%',
},
{
name: 'Ice cream sandwich',
calories: 237,
fat: 9.0,
carbs: 37,
protein: 4.3,
iron: '1%',
},
{
name: 'Eclair',
calories: 262,
fat: 16.0,
carbs: 23,
protein: 6.0,
iron: '7%',
},
{
name: 'Cupcake',
calories: 305,
fat: 3.7,
carbs: 67,
protein: 4.3,
iron: '8%',
},
{
name: 'Gingerbread',
calories: 356,
fat: 16.0,
carbs: 49,
protein: 3.9,
iron: '16%',
},
{
name: 'Jelly bean',
calories: 375,
fat: 0.0,
carbs: 94,
protein: 0.0,
iron: '0%',
},
{
name: 'Lollipop',
calories: 392,
fat: 0.2,
carbs: 98,
protein: 0,
iron: '2%',
},
{
name: 'Honeycomb',
calories: 408,
fat: 3.2,
carbs: 87,
protein: 6.5,
iron: '45%',
},
{
name: 'Donut',
calories: 452,
fat: 25.0,
carbs: 51,
protein: 4.9,
iron: '22%',
},
{
name: 'KitKat',
calories: 518,
fat: 26.0,
carbs: 65,
protein: 7,
iron: '6%',
},
],
};
},
computed: {
headers() {
return [
{
text: 'Dessert (100g serving)',
align: 'start',
sortable: false,
value: 'name',
},
{
text: 'Calories',
value: 'calories',
filter: (value) => {
return this.calories ? value < parseInt(this.calories) : true;
},
},
{ text: 'Fat (g)', value: 'fat' },
{ text: 'Carbs (g)', value: 'carbs' },
{ text: 'Protein (g)', value: 'protein' },
{ text: 'Iron (%)', value: 'iron' },
];
},
},
methods: {
filterOnlyCapsText(value, search, item) {
return (
value != null &&
search != null &&
typeof value === 'string' &&
value.toString().toLocaleUpperCase().indexOf(search) !== -1
);
},
},
};
</script>
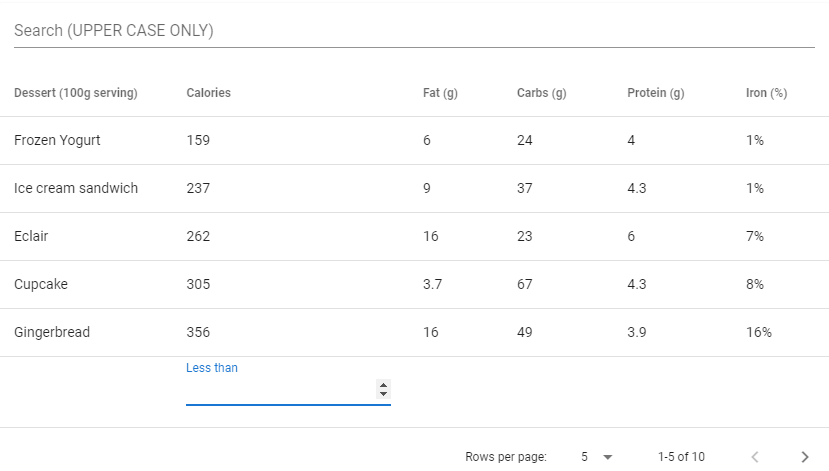
Vuetify Data Table Filterable
We can prevent specific columns from being included when searching through the table rows by setting the filterable
property to false
on the element of the array passed to headers
that is associated with the column. In the code example below, we’ve turned off searching for the dessert name column:
<template>
<v-app>
<v-card class="ma-4">
<v-card-title>
<v-text-field
v-model="search"
append-icon="mdi-magnify"
label="Search"
single-line
hide-details
></v-text-field>
</v-card-title>
<v-data-table
:headers="headers"
:items="desserts"
:search="search"
:items-per-page="5"
></v-data-table>
</v-card>
</v-app>
</template>
<script>
export default {
name: 'App',
data() {
return {
search: '',
headers: [
{
text: 'Dessert (100g serving)',
align: 'start',
filterable: false,
value: 'name',
},
{ text: 'Calories', value: 'calories' },
{ text: 'Fat (g)', value: 'fat' },
{ text: 'Carbs (g)', value: 'carbs' },
{ text: 'Protein (g)', value: 'protein' },
{ text: 'Iron (%)', value: 'iron' },
],
desserts: [
{
name: 'Frozen Yogurt',
calories: 159,
fat: 6.0,
carbs: 24,
protein: 4.0,
iron: '1%',
},
{
name: 'Ice cream sandwich',
calories: 237,
fat: 9.0,
carbs: 37,
protein: 4.3,
iron: '1%',
},
{
name: 'Eclair',
calories: 262,
fat: 16.0,
carbs: 23,
protein: 6.0,
iron: '7%',
},
{
name: 'Cupcake',
calories: 305,
fat: 3.7,
carbs: 67,
protein: 4.3,
iron: '8%',
},
{
name: 'Gingerbread',
calories: 356,
fat: 16.0,
carbs: 49,
protein: 3.9,
iron: '16%',
},
{
name: 'Jelly bean',
calories: 375,
fat: 0.0,
carbs: 94,
protein: 0.0,
iron: '0%',
},
{
name: 'Lollipop',
calories: 392,
fat: 0.2,
carbs: 98,
protein: 0,
iron: '2%',
},
{
name: 'Honeycomb',
calories: 408,
fat: 3.2,
carbs: 87,
protein: 6.5,
iron: '45%',
},
{
name: 'Donut',
calories: 452,
fat: 25.0,
carbs: 51,
protein: 4.9,
iron: '22%',
},
{
name: 'KitKat',
calories: 518,
fat: 26.0,
carbs: 65,
protein: 7,
iron: '6%',
},
],
};
},
};
</script>
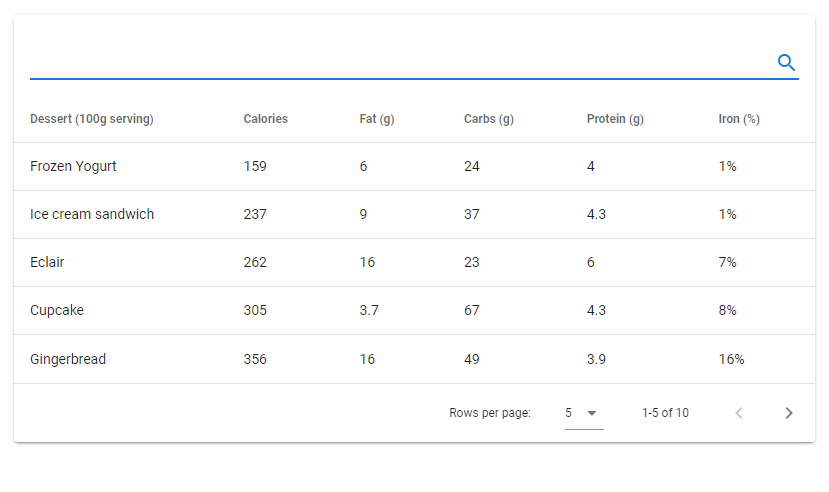
Footer Props
By default, the data table component displays a default footer using the v-data-footer
component. We can pass props to this component with the footer-props
prop of v-data-table
:
<template>
<v-app>
<v-data-table
:headers="headers"
:items="desserts"
:items-per-page="5"
item-key="name"
class="elevation-1 ma-4"
:footer-props="{
showFirstLastPage: true,
firstIcon: 'mdi-arrow-collapse-left',
lastIcon: 'mdi-arrow-collapse-right',
prevIcon: 'mdi-minus',
nextIcon: 'mdi-plus',
}"
></v-data-table>
</v-app>
</template>
<script>
export default {
name: 'App',
data() {
return {
headers: [
{
text: 'Dessert (100g serving)',
align: 'start',
value: 'name',
},
{ text: 'Category', value: 'category' },
],
desserts: [
{
name: 'Frozen Yogurt',
category: 'Ice cream',
},
{
name: 'Ice cream sandwich',
category: 'Ice cream',
},
{
name: 'Eclair',
category: 'Cookie',
},
{
name: 'Cupcake',
category: 'Pastry',
},
{
name: 'Gingerbread',
category: 'Cookie',
},
{
name: 'Jelly bean',
category: 'Candy',
},
{
name: 'Lollipop',
category: 'Candy',
},
{
name: 'Honeycomb',
category: 'Toffee',
},
{
name: 'Donut',
category: 'Pastry',
},
{
name: 'KitKat',
category: 'Candy',
},
],
};
},
};
</script>
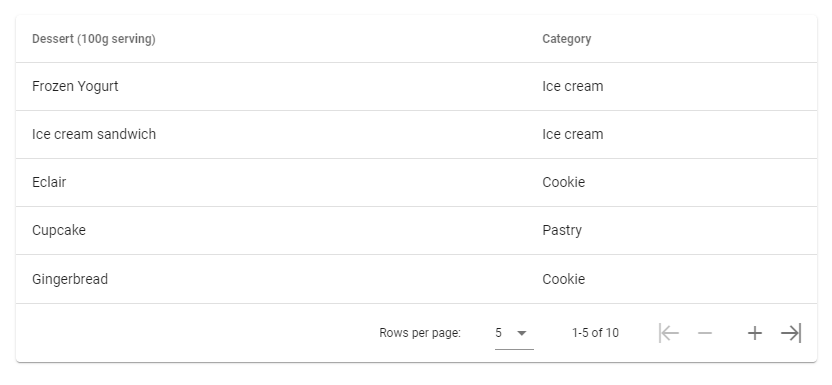
Conclusion
The Vuetify data table component (v-data-table
) comes in handy for laying out data in a digestible format. We can not only display data but perform searching and other operations. It also allows UI customizations like making the table more compact and functional customizations such as specifying a custom filter function.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
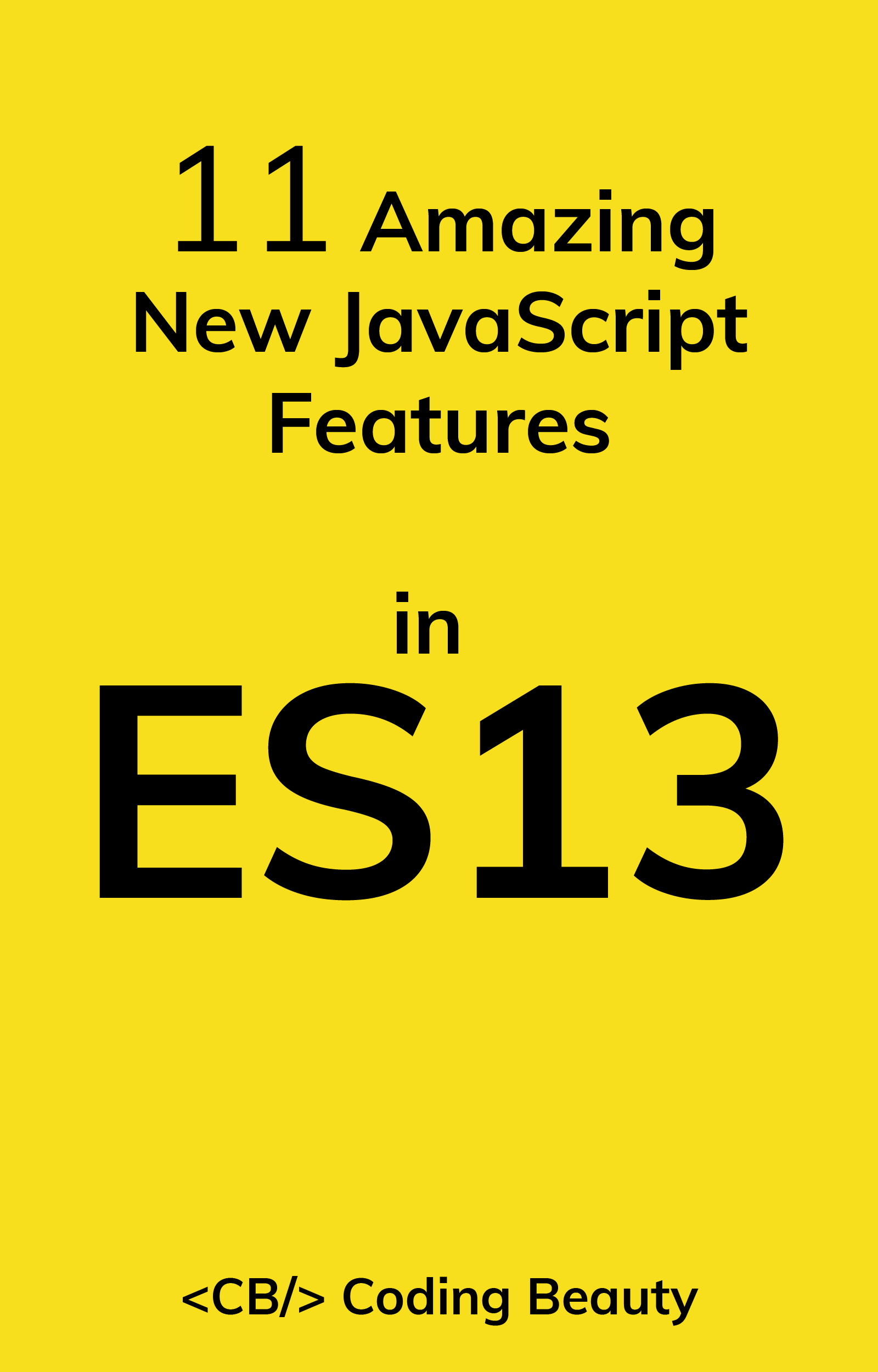