Add Element to Array With push() Method
To add an element to an array in Vue, call the push()
method in the array with the element as an argument. The push()
method will add the element to the end of the array.
For example:
<template>
<div id="app">
<button @click="addFruit">Add fruit</button>
<ul>
<h2
v-for="(fruit, i) in fruits"
:key="i"
>
{{ fruit }}
</h2>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
fruits: ['Orange', 'Apple'],
};
},
methods: {
addFruit() {
this.fruits.push('Banana');
},
},
};
</script>
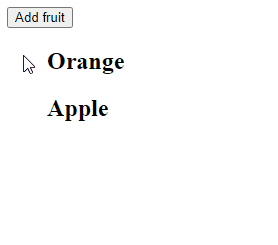
The Array push() method adds one or more elements to the end of an array and returns the length of the array.
We use the v-for
Vue directive to display the elements in the array. These rendered elements are automatically updated in the view when the array is modified with push()
.
Add Object Element to Array in Vue
We can use the same approach to add an object to an array and display more complex data. We just have to make sure that we render the properties of each object in the array, not the object itself.
<template>
<div id="app">
<button @click="addFruit">Add fruit</button>
<ul>
<h2
v-for="(fruit, i) in fruits"
:key="i"
>
<!-- Render "name" and "amount" properties -->
{{ fruit.name }} ({{ fruit.amount }})
</h2>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
fruits: [
{ name: 'Orange', amount: 3 },
{ name: 'Apple', amount: 5 },
],
};
},
methods: {
addFruit() {
// Add object element to array
this.fruits.push({ name: 'Banana', amount: 4 });
},
},
};
</script>
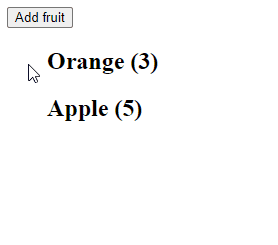
Like before, the list automatically updates in the view when a new object element is added to the array.