To use a button as a link in React, wrap the button in an anchor (<a>
) element. Clicking a link button will make the browser navigate to the specified URL.
export default function MyComponent() {
return (
<div>
<a href="/posts">
<button>Posts</button>
</a>
</div>
);
}
Use button as React Router link
If you’re using React Router, then wrap the button in the Link
component instead, to make the browser navigate to the specified route without refreshing the page when the button is clicked.
import { Link } from 'react-router-dom';
export default function MyComponent() {
return (
<div>
<Link to="/posts">
<button>Posts</button>
</Link>
</div>
);
}
The react-router-dom
Link
component renders an anchor element, so it works similarly to the first example.
We can also use custom button components as links by wrapping them with an anchor element or Link
component. For example:
import { Link } from 'react-router-dom';
function MyCustomButton({ children }) {
return <button>{children}</button>;
}
export default function MyComponent() {
return (
<div>
<Link to="/posts">
<MyCustomButton>Posts</MyCustomButton>
</Link>
</div>
);
}
Use Material UI button as link
When using Material UI, we can specify a link for the Button
component using the href
prop.
import { Button } from '@mui/material';
export default function MyComponent() {
return (
<div>
<Button href="/posts">Posts</Button>
</div>
);
}
Use Material UI button as React Router link
To use as a React Router Link
, we can use the component
prop of the Button
.
import { Button } from '@mui/material';
import { Link } from 'react-router-dom';
export default function MyComponent() {
return (
<div>
<Button component={Link} to="/posts">
Posts
</Button>
</div>
);
}
Other Material UI components like IconButton
also have a component
prop. Setting this prop is useful when we want the button component to inherit its color from its parent.
In this scenario, if we wrap the component with a Link
, it will inherit its color from the Link
instead of the intended parent. For example:
import { IconButton, Box, Typography } from '@mui/material';
import { Link } from 'react-router-dom';
import { Photo } from '@mui/icons-material';
export default function MyComponent() {
return (
// We want the IconButton to inherit its parent color (white)
<Box sx={{ backgroundColor: 'black', color: 'white', padding: 2 }}>
<Typography>Lorem Ipsum</Typography>
{/* But this wrapping makes it inherit from this Link */}
<Link to="/photos">
<IconButton color="inherit">
<Photo />
</IconButton>
</Link>
</Box>
);
}
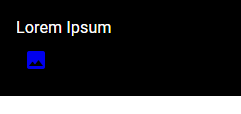
IconButton
inherits its color from the Link
(blue) instead of the Box
(white).We can fix this issue by setting the component
prop to a Link
instead of wrapping:
import { IconButton, Box, Typography } from '@mui/material';
import { Link } from 'react-router-dom';
import { Photo } from '@mui/icons-material';
export default function MyComponent() {
return (
<Box sx={{ backgroundColor: 'black', color: 'white', padding: 2 }}>
<Typography>Lorem Ipsum</Typography>
{/* Setting the "component" prop to a "Link" component */}
<IconButton component={Link} to="/photos" color="inherit">
<Photo />
</IconButton>
</Box>
);
}
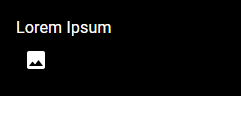
IconButton
inherits its color from the Box
.11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
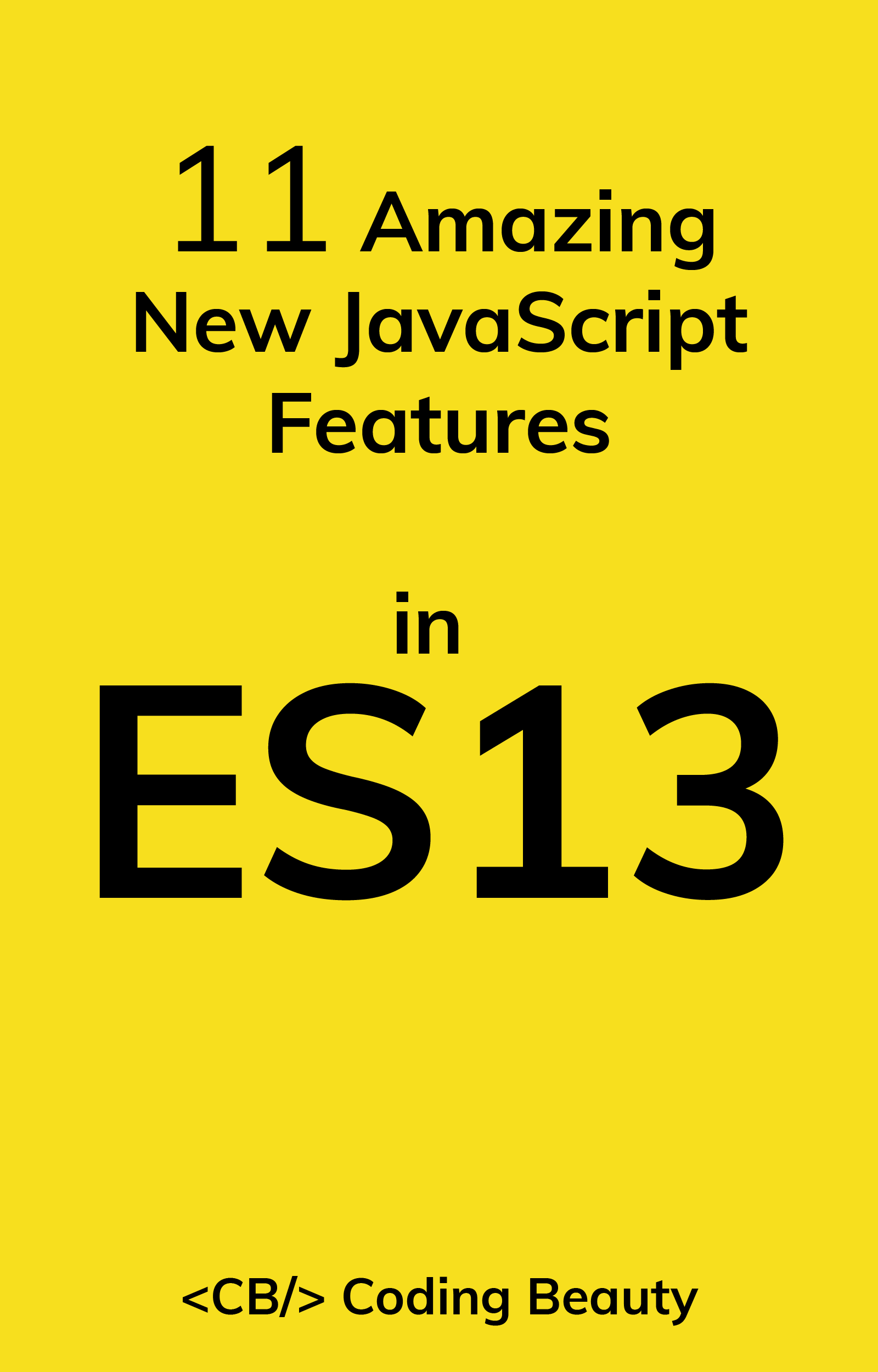