To remove the ID attribute from an element, call the removeAttribute()
method on the element, passing the string 'id'
as an argument.
Example
index.html
<!DOCTYPE html>
<html>
<head>
<title>
Removing the ID Attribute from an Element with
JavaScript
</title>
</head>
<body>
<div class="box" id="box-1">This is a box</div>
<script src="index.js"></script>
</body>
</html>
Here’s how we can remove the ID from the div
element we created:
index.js
const box = document.getElementById('box-1');
console.log(box.getAttribute('id')); // box-1
box.removeAttribute('id');
console.log(box.getAttribute('id')); // null
The Element
removeAttribute() method removes the attribute of an element with a specified name.
Note: If the specified attribute doesn’t exist, removeAttribute()
returns instead of throwing an error.
Element
setAttribute()
method
If you would like to replace the ID of the element instead of removing it, you can use the setAttribute()
method.
For example, we can change the ID of the div
element we created earlier to a new value.
For example:
index.js
const box = document.getElementById('box-1');
console.log(box.getAttribute('id')); // box-1
box.setAttribute('id', 'new-id');
console.log(box.getAttribute('id')); // new-id
The Element
setAttribute() method takes two arguments:
name
: A string specifying the name of the attribute whose value is to be set.value
: A string containing the value to assign to the attribute.
We pass 'id'
and the new id as the first and second arguments respectively, to set the ID attribute.
Note: If the attribute already exists on the element, the value is updated. Otherwise, a new attribute is added with the specified name and value.
The Element
getAttribute()
method
We’ve used the getAttribute() method a number of times in this article. Calling this method on an Element
returns the value of the attribute with the specified name. So we call it with 'id'
to get the ID of the element.
Note: If the attribute does not exist on the element, getAttribute()
returns null
or an empty string (''
), depending on the browser.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
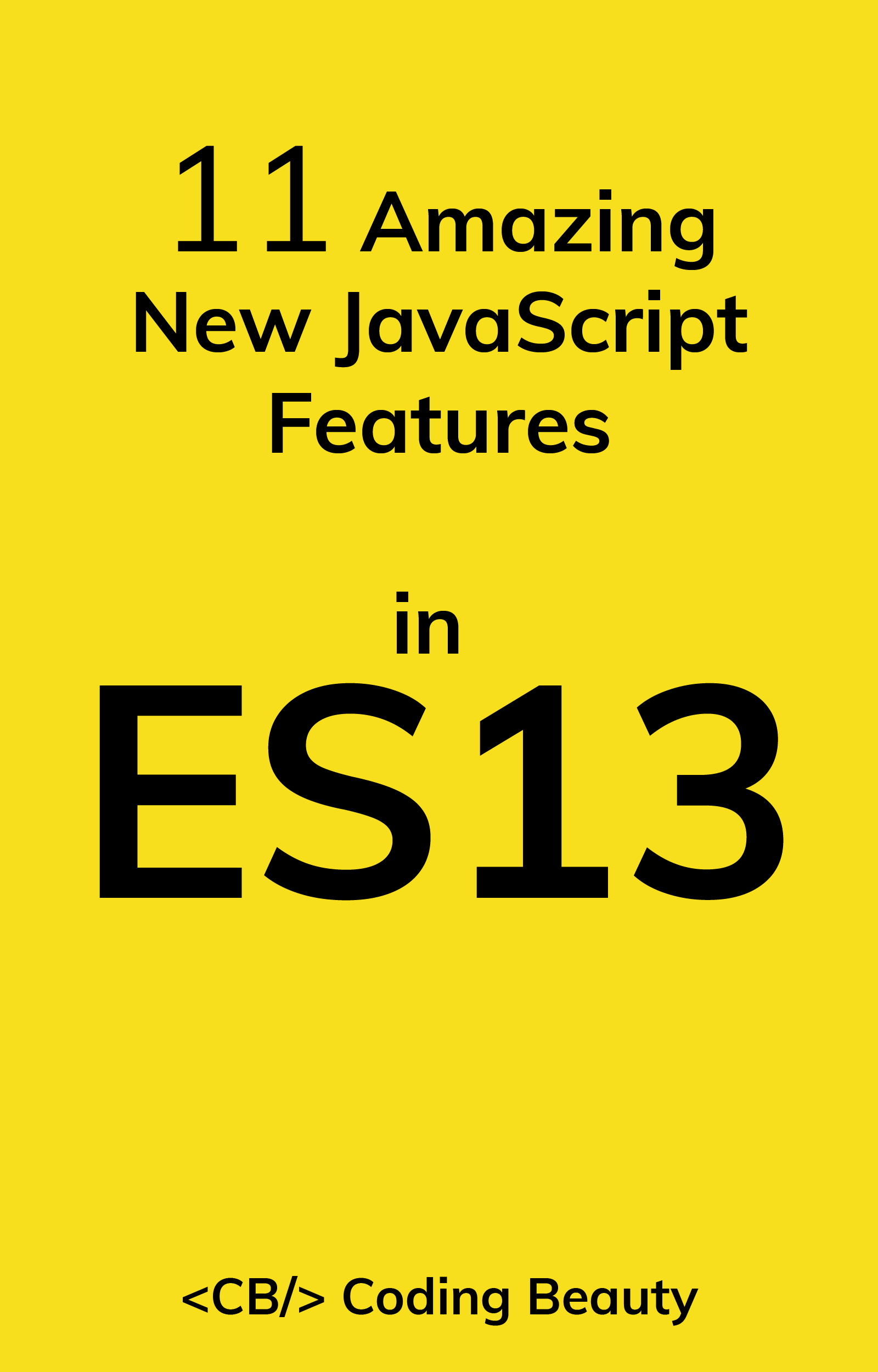