To remove an element from the DOM onclick in JavaScript:
- Select the DOM element with a method like
getElementById()
. - Add a
click
event listener to the element. - Call the
remove()
method on the element in the event handler.
const element = document.getElementById('el');
element.remove();
Consider this sample HTML where we create a blue box.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Coding Beauty Tutorial</title>
<style>
#box {
height: 100px;
width: 100px;
background-color: blue;
}
</style>
</head>
<body>
Click the box to remove it.
<div id="box"></div>
<script src="index.js"></script>
</body>
</html>
Here’s how we can cause the element to be removed when it is clicked.
index.js
const box = document.getElementById('box');
box.addEventListener('click', () => {
box.remove();
});
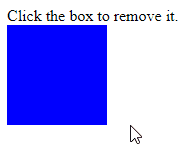
We used the addEventListener()
method to add a handler for the click
event to the #box
element. This event handler will be called whenever the user clicks the box.
In the handler function, we called the remove()
method on the element to remove it from the DOM.
We could also have used the target
property on the event
object passed to the handler to remove the element.
const box = document.getElementById('box');
box.addEventListener('click', (event) => {
event.target.remove();
});
We can use the event
object to access useful information and perform certain actions related to the event. For the click
event, the target
property lets us access the DOM element that was clicked.
Removing the element with the target
property is useful when we want to dynamically remove many elements onclick. For example:
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Coding Beauty Tutorial</title>
<link rel="stylesheet" href="index.css" />
</head>
<body>
Click on a box to remove it.
<div class="container">
<div class="box" id="box-1"></div>
<div class="box" id="box-2"></div>
<div class="box" id="box-3"></div>
</div>
<script src="index.js"></script>
</body>
</html>
index.css
.container {
display: flex;
}
.box {
height: 100px;
width: 100px;
margin: 5px;
}
#box-1 {
background-color: blue;
}
#box-2 {
background-color: red;
}
#box-3 {
background-color: green;
}
index.js
const boxes = document.getElementsByClassName('box');
for (const box of boxes) {
box.addEventListener('click', (event) => {
event.target.remove();
});
}
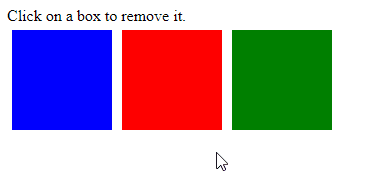
We can also remove any one of the elements onclick by adding a single event listener to the parent of all the elements.
index.js
const container = document.querySelector('.container');
container.addEventListener('click', (event) => {
event.target.remove();
});
This is because the target
property returns the innermost element in the DOM that was clicked. This is in contrast to the event.currentTarget
property, which returns the element that the event listener was added to.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
