To remove a class from multiple elements in JavaScript:
- Get a list of all the elements with a method like
document.querySelectorAll(selector)
. - Iterate over the list with
forEach()
. - For each element, call
classList.remove(class)
to remove the class from each element.
i.e.:
const elements = document.querySelectorAll('.class');
elements.forEach((element) => {
element.classList.remove('class');
});
For example:
HTML
<p class="big bold text">Coding</p>
<p class="big bold text">Beauty</p>
<p class="big bold text">Dev</p>
<button id="remove">Remove class</button>
JavaScript
const removeBtn = document.getElementById('remove');
removeBtn.addEventListener('click', () => {
const elements = document.querySelectorAll('.text');
elements.forEach((element) => {
element.classList.remove('big');
});
});
CSS
.bold {
font-weight: bold;
}
.big {
font-size: 1.2em;
}
This will be the HTML after the button is clicked:
<p class="bold text">Coding</p>
<p class="bold text">Beauty</p>
<p class="bold text">Dev</p>
<button id="remove">Remove class</button>
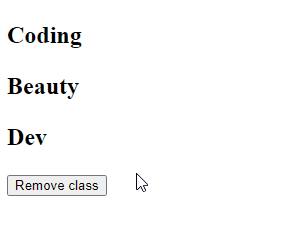
big
class is removed from the texts when the button is clicked.We use the document.querySelectorAll()
method to select all DOM elements from which we want to remove the class.
We iterate over the elements in the list object with the forEach()
method. This forEach()
method works similarly to Array
forEach()
.
document.getElementsByClassName()
method
We can use the document.getElementsByClassName()
method in place of the document.querySelectorAll()
method when the selector is a class selector. For getElementsByClassName()
, we pass the class name without the .
(dot), and we use Array.from()
to convert the result to an array before the iteration with forEach()
.
const elements = Array.from(document.getElementsByClassName('text'));
elements.forEach((element) => {
element.classList.remove('big');
});
classList.remove()
method
We use the classList.remove()
method to remove a class from the elements. You can remove multiple classes by passing more arguments to remove()
.
const elements = document.querySelectorAll('.text');
elements.forEach((element) => {
element.classList.remove('big', 'bold');
});
If any of the classes passed to remove()
doesn’t exist on the element, remove()
will ignore it, instead of throwing an error.
Remove class from multiple elements with different selectors
Sometimes there is no common selector between the elements that you want to remove the class from. For such a case, you can pass multiple comma-separated selectors to the querySelectorAll()
method.
const elements = document.querySelectorAll('.text, #box-1, #box-2');
elements.forEach((element) => {
element.classList.remove('bold');
});
Add class to multiple elements
Just like the classList.remove()
method removes one or more classes from an element, the classList.add()
method adds one or more classes to an element. This means that we can use it in the forEach()
method to remove a class from multiple DOM elements:
const elements = document.querySelectorAll('.text');
elements.forEach((element) => {
element.classList.add('italic', 'underline');
});
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
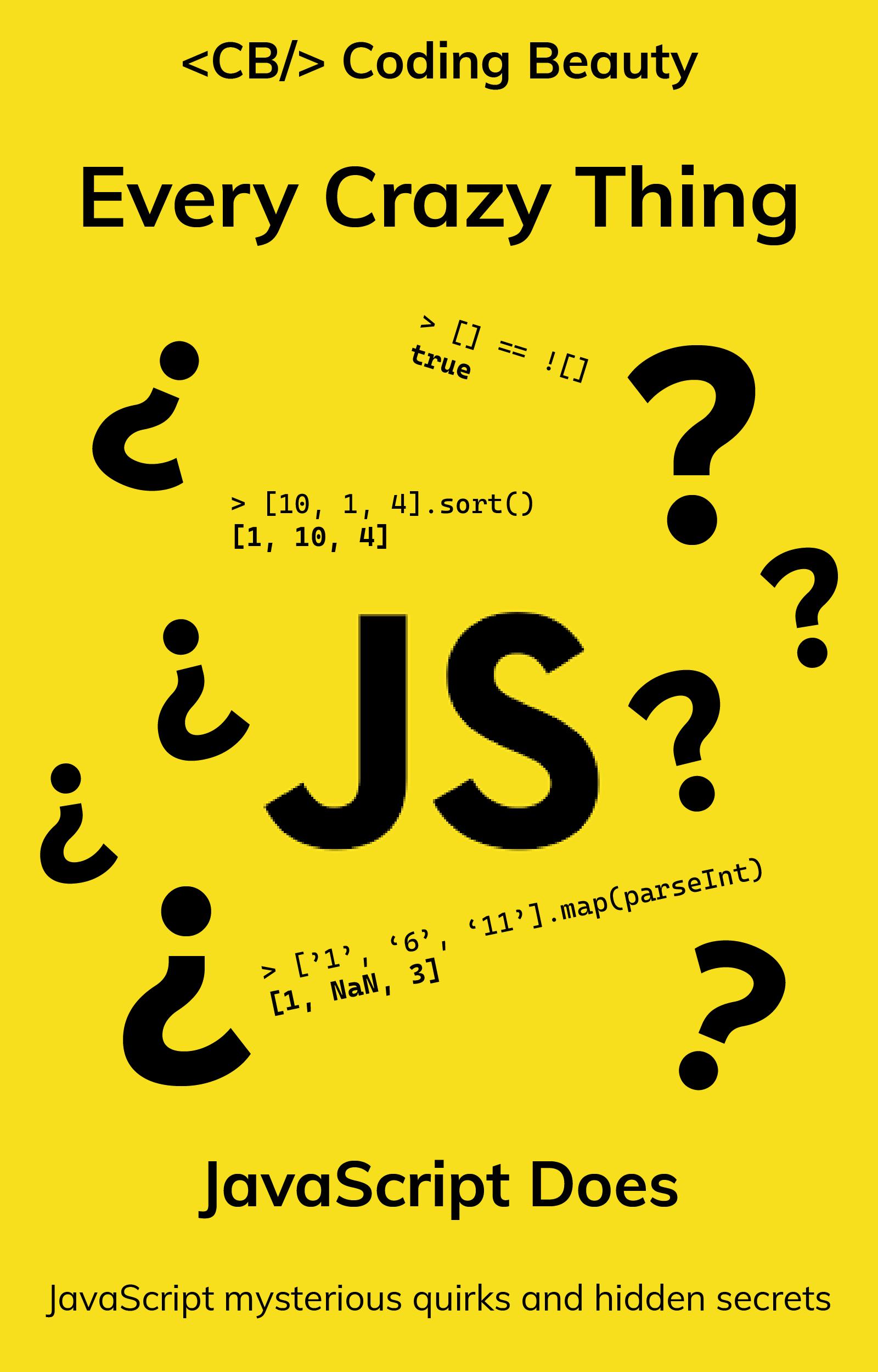