Related: How to Remove a Class From All Elements in JavaScript
To remove all classes from an element in JavaScript, set the className
property of the element to an empty string (''
), i.e., element.className = ''
. Setting the className
property to an empty string will eliminate every class in the element.
const box = document.getElementById('box');
// 👇 Remove all classes from element.
box.className = '';
For this HTML:
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Coding Beauty Tutorial</title>
<style>
.class-1 {
height: 30px;
width: 300px;
font-size: 1.1em;
box-shadow: 0 2px 3px #c0c0c0;
}
.class-2 {
background-color: blue;
color: white;
border: 1px solid black;
border-radius: 3px;
}
</style>
</head>
<body>
<input id="input" type="text" class="class-1 class-2" />
<br /><br />
<button id="btn">Remove classes</button>
<script src="index.js"></script>
</body>
</html>
This JavaScript code will remove all classes from the input field when the button is clicked:
index.js
const btn = document.getElementById('btn');
const input = document.getElementById('input');
btn.addEventListener('click', () => {
input.className = '';
});
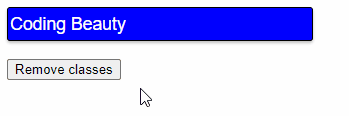
The className
property is used to get and set the value of the class
attribute of a specified element.
Setting className
to an empty string effectively removes all the classes from the element.
Remove all classes from element with removeAttribute()
method
To remove all classes from an element with this approach, call the removeAttribute()
method on the specified for the class
attribute, i.e., element.removeAttribute('class')
. This method will remove the class
attribute from the element, effectively removing all the element’s classes.
const box = document.getElementById('box');
// 👇 Remove all classes from element
box.removeAttribute('class');
removeAttribute()
takes a name and removes the attribute from an element with that name.
For this HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Coding Beauty Tutorial</title>
<style>
.class-1 {
height: 30px;
font-size: 1.1em;
box-shadow: 0 2px 3px #c0c0c0;
}
.class-2 {
background-image: linear-gradient(to bottom, rgb(136, 136, 255), blue);
color: white;
border: none;
}
</style>
</head>
<body>
<button id="styled-btn" type="text" class="class-1 class-2">
Download
</button>
<br /><br />
<button id="btn">Remove classes</button>
<script src="index.js"></script>
</body>
</html>
This JavaScript code will remove all classes from the styled button when the other button is clicked.
const btn = document.getElementById('btn');
const styledButton = document.getElementById('styled-btn');
btn.addEventListener('click', () => {
styledButton.removeAttribute('class');
});
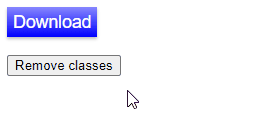
In the first method, the class
attribute remains on the element after setting the className
property. But using the removeAttribute()
method completely removes the class
attribute from the element.
If the element doesn’t have a class
attribute, removeAttribute()
will return without causing an error.
Either of these two methods is fine, it’s up to you which one to pick. I think using the className
property is better because it more clearly shows what you’re trying to do.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
