JavaScript Mutation Observer.
An underrated API for watching for changes in the DOM: “child added”, “attribute changed”, and more.
// Node/element to be observed for mutations
const targetNode = document.getElementById('my-el');
// Options for the observer (which mutations to observe)
const config = { attributes: true, childList: true, subtree: true };
// Callback function to execute when mutations are observed
const callback = (mutationList, observer) => {
for (const mutation of mutationList) {
if (mutation.type === 'childList') {
console.log('A child node has been added or removed.');
} else if (mutation.type === 'attributes') {
console.log(
`The ${mutation.attributeName} attribute was modified.`
);
}
}
};
// Create an observer instance linked to the callback function
const observer = new MutationObserver(callback);
// Start observing the target node for configured mutations
observer.observe(targetNode, config);
// Later, you can stop observing
observer.disconnect();
I see a bunch of people online curious to know the point of this API in the real-world.
In this post I’ll show you how I used it to easily shut-up this annoying script carrying out unwanted activities on the page.
And you’ll see how sleek and polish the UI elements became after this.
The 3rd party script
It came from Mailchimp – a service for managing email lists.
Mailchimp provides embedded forms to easily collect sign-ups to your list.
These forms are HTML code embedded in your webpage without any additional configuration.
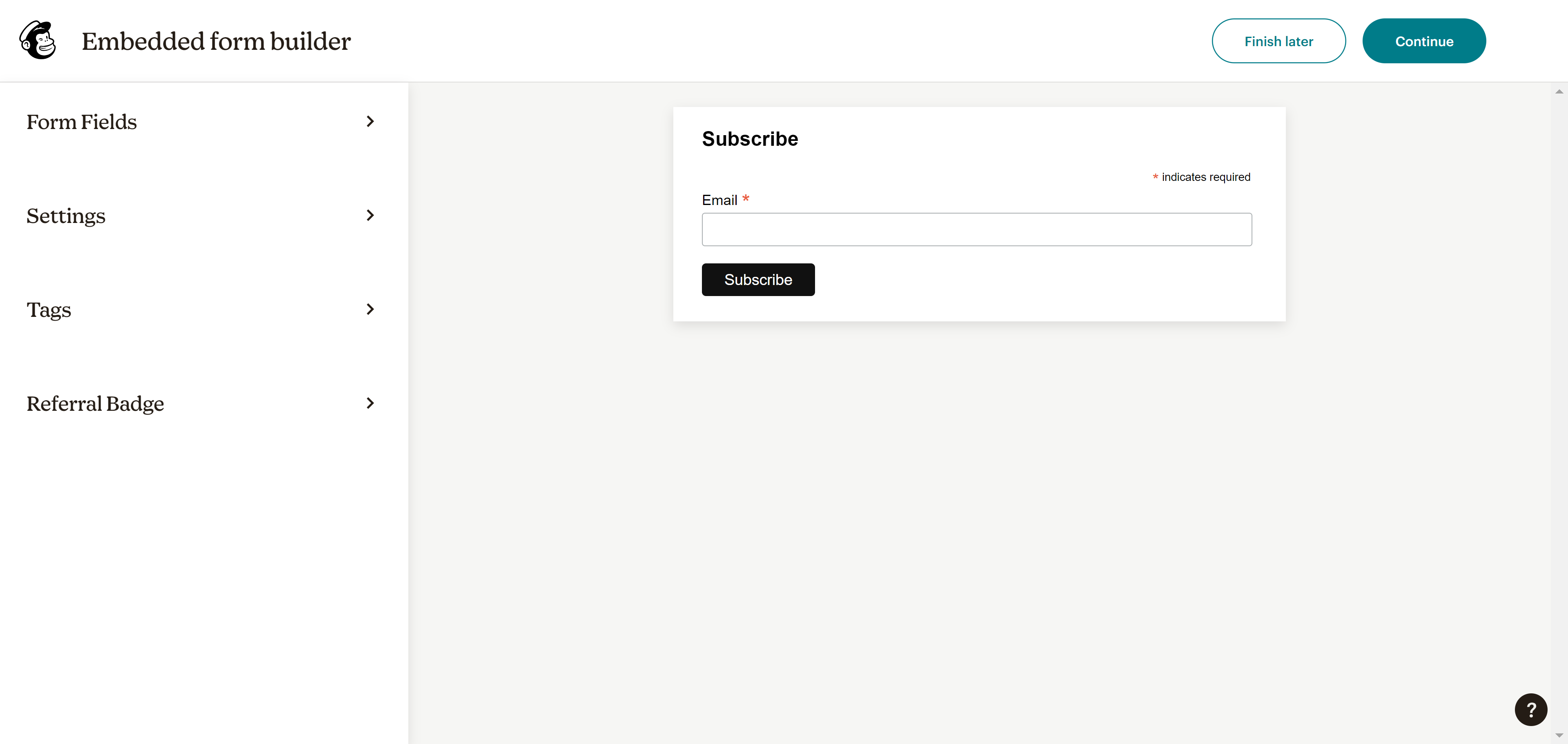
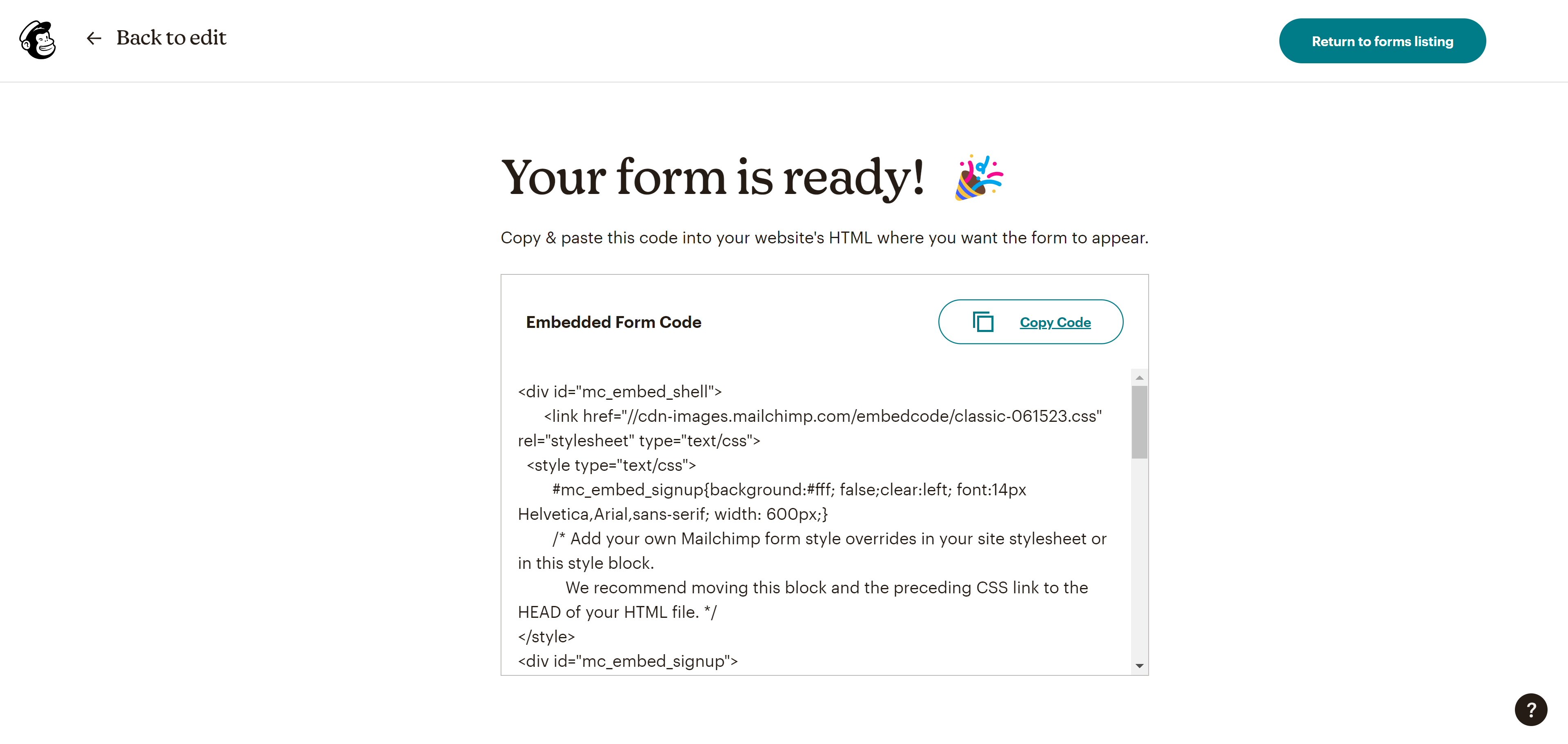
But being this ready-to-use nature comes at a cost; deeply embedded CSS stubbornly resisting any attempt to customize the form’s look and feel.
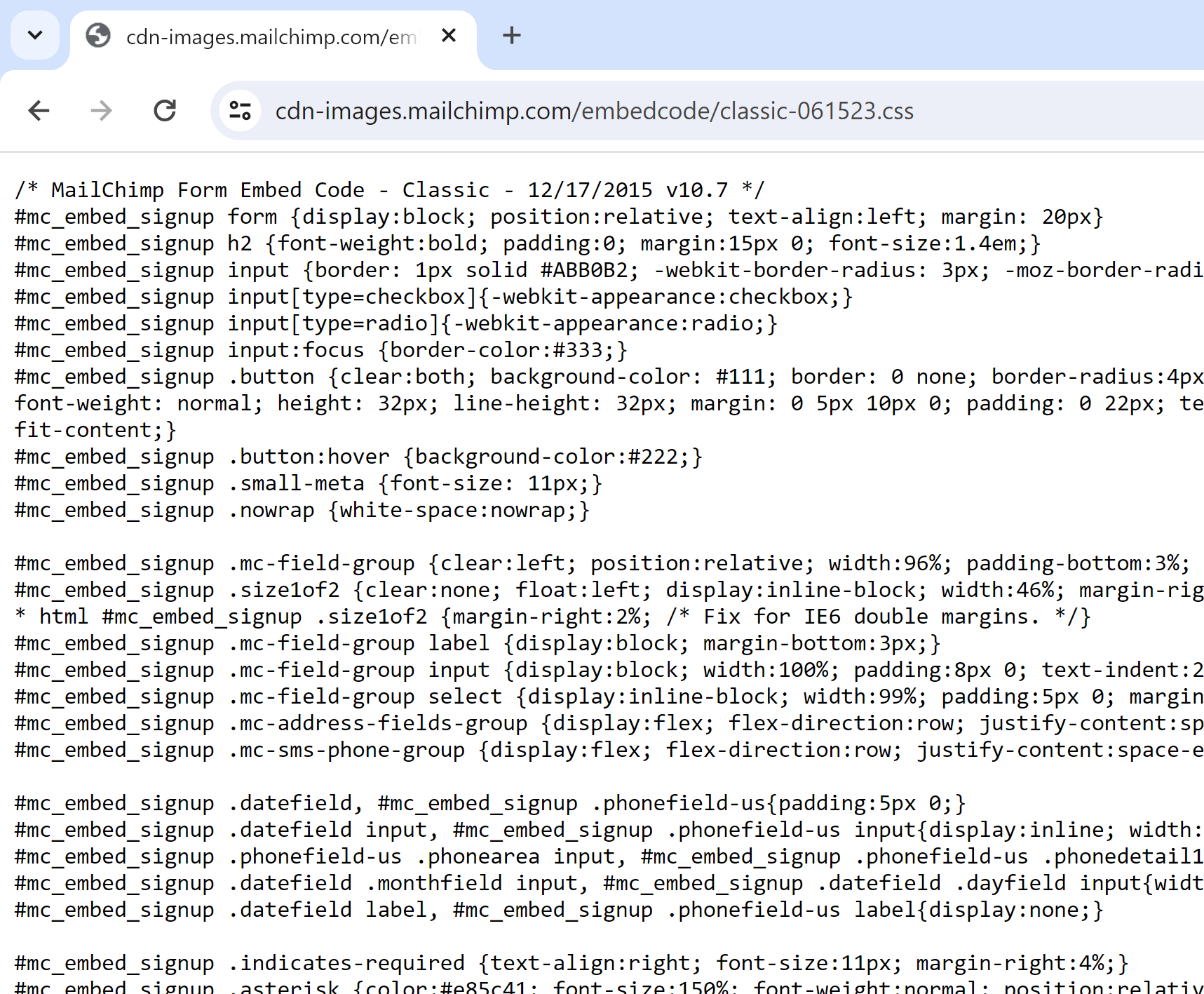
I mean I know I definitely spent WAY more than I should have doing this, having to throw CSS !important
all over the place and all…
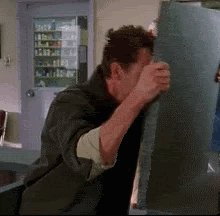
Stubbornly rigid JS
On the JS side I had the remote Mailchimp script showing these pre-defined error/success messages after the request to the Mailchimp API.
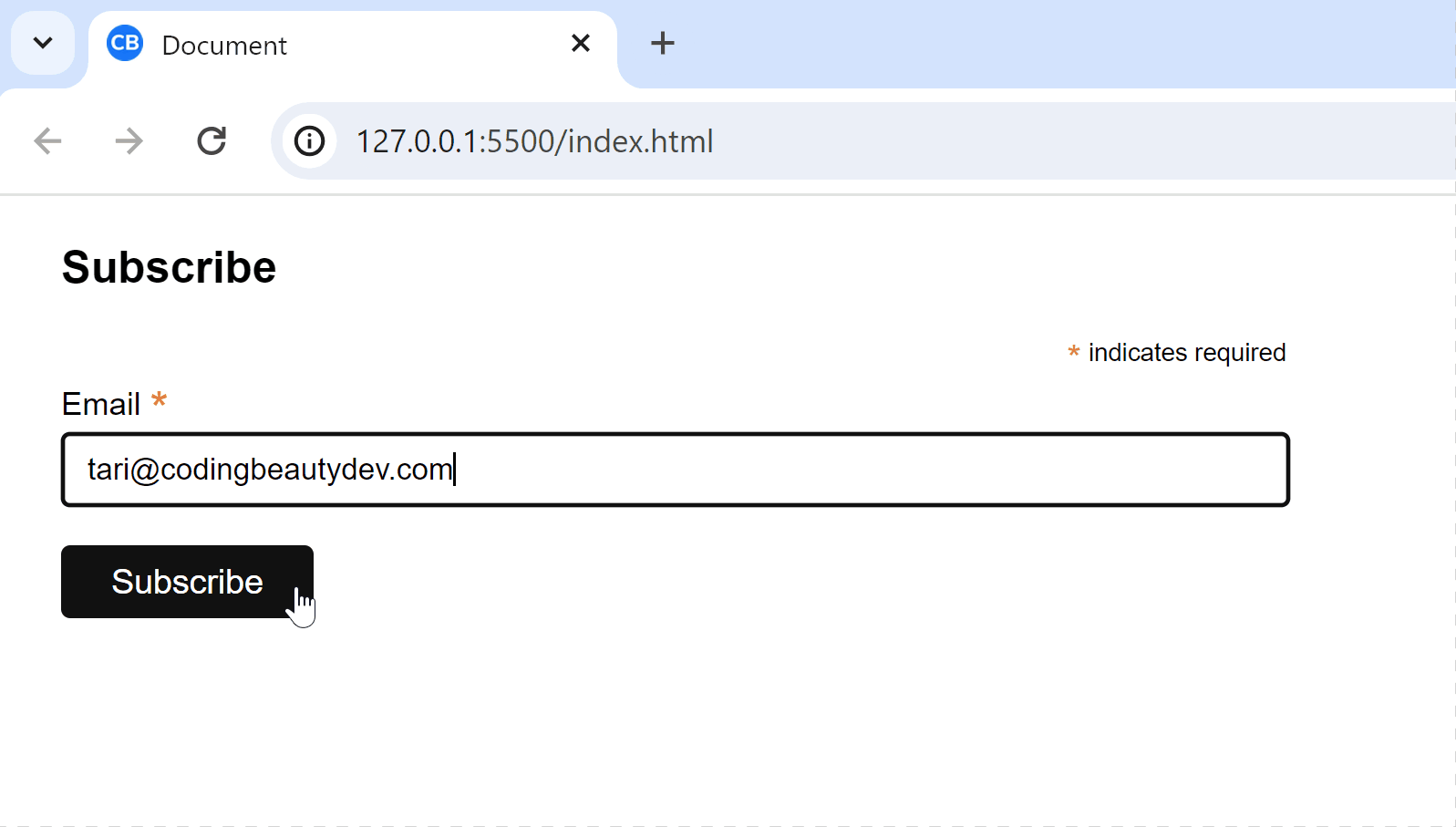
Sure this was an decent success message but there was simply no built-in option to customize it. And we couldn’t indicate success in other ways like a color or icon change.
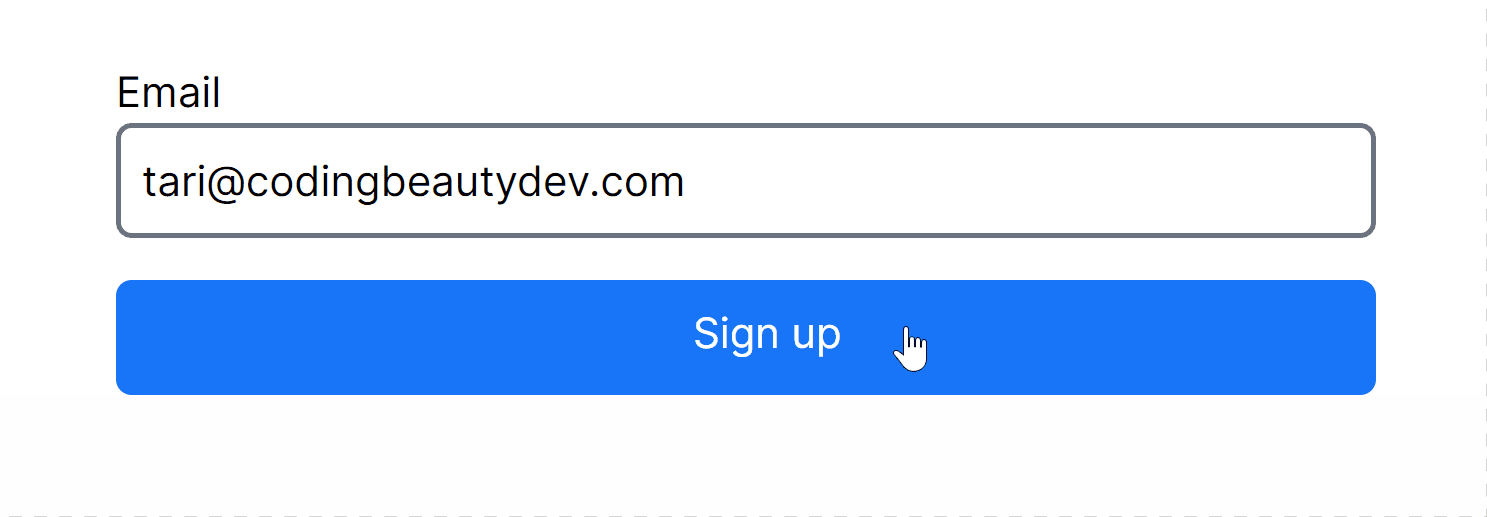
Mutation Observer to the rescue…
Waiting for the precise moment the success text came in and instantly swapping out the copy for whatever I wanted and doing anything else.
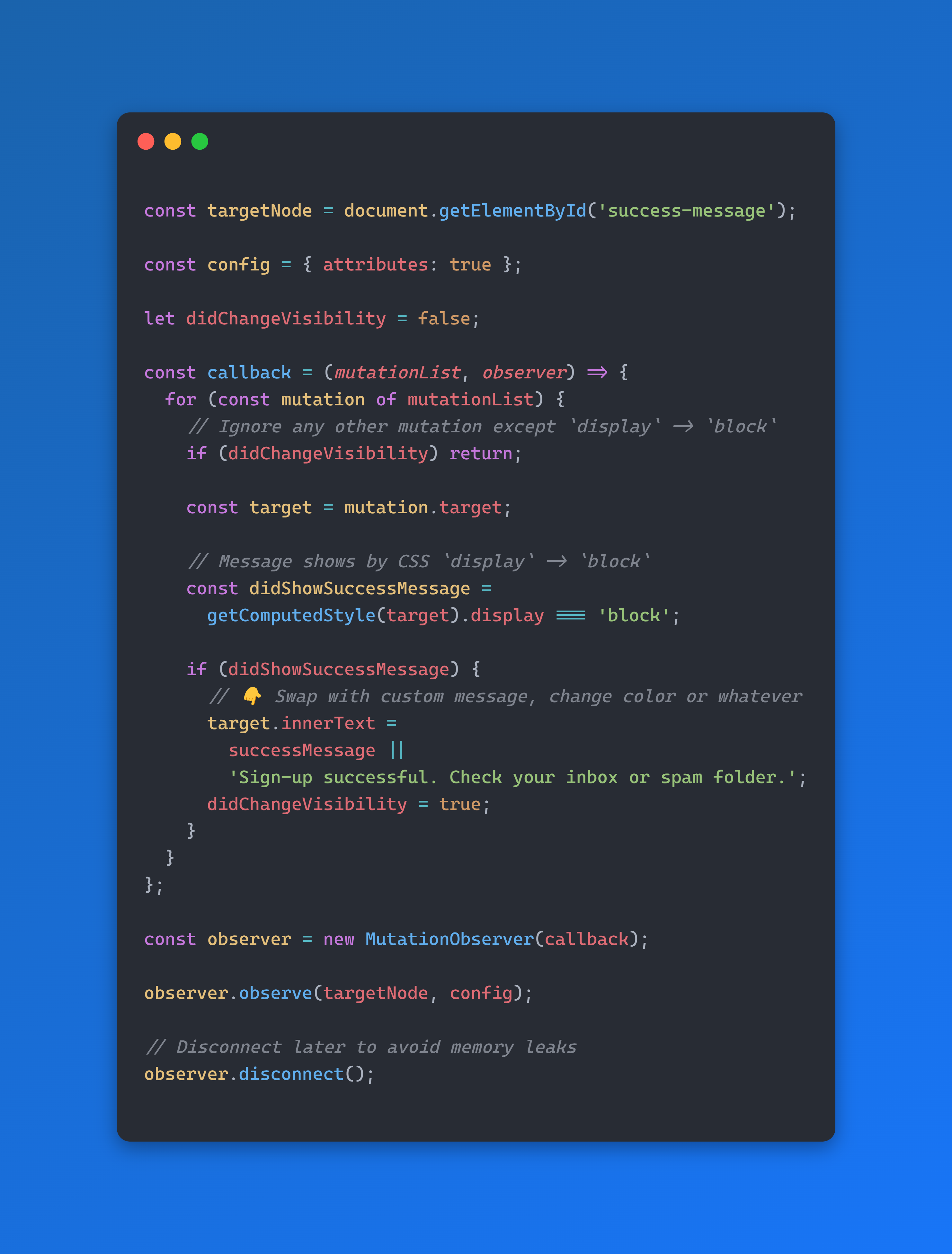
And just like that the script was blocked from directly affecting the UI.
We basically turned it into an Event Emitter service; an observable.
This let us easily abstract everything into a React component and add various event listeners (like onSuccess
and onError
):
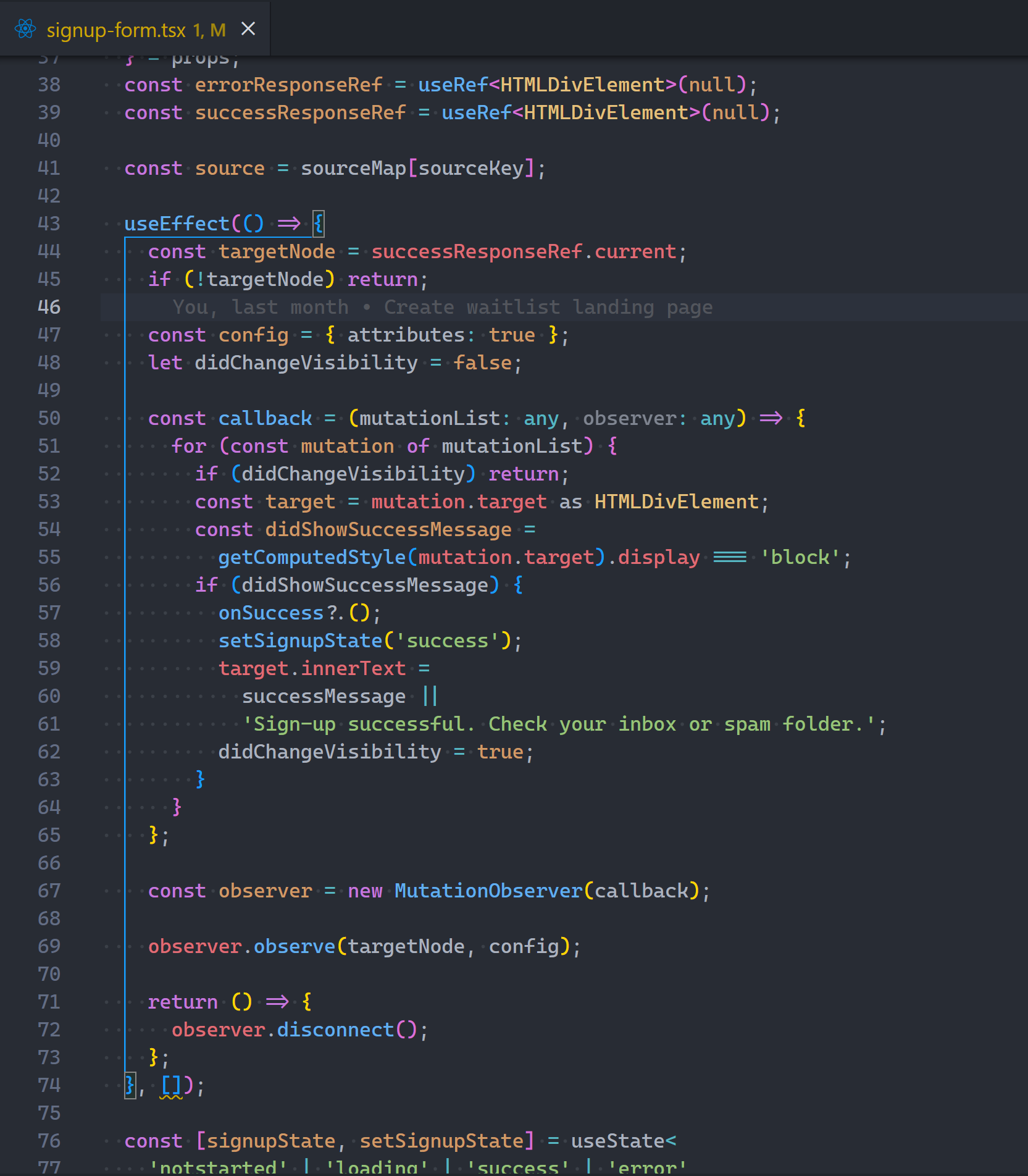
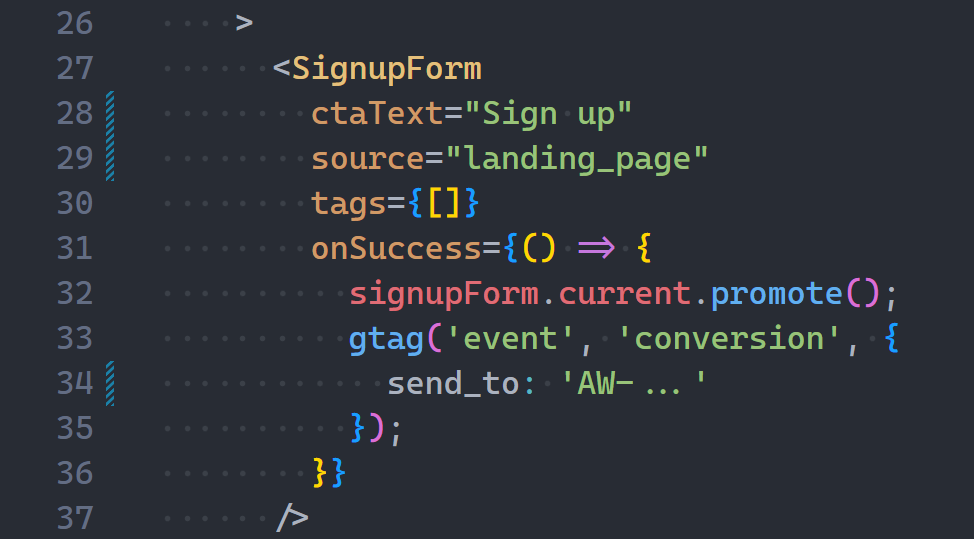
To create the polished form we saw earlier:
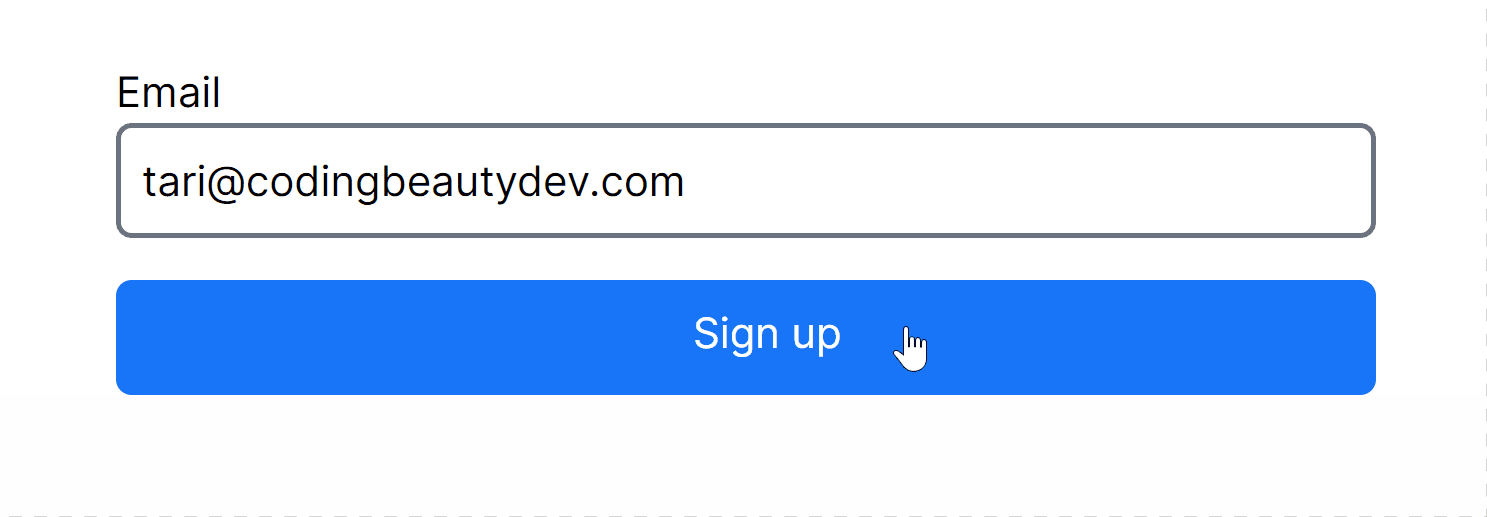
This is the power of the JavaScript Mutation Observer API.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
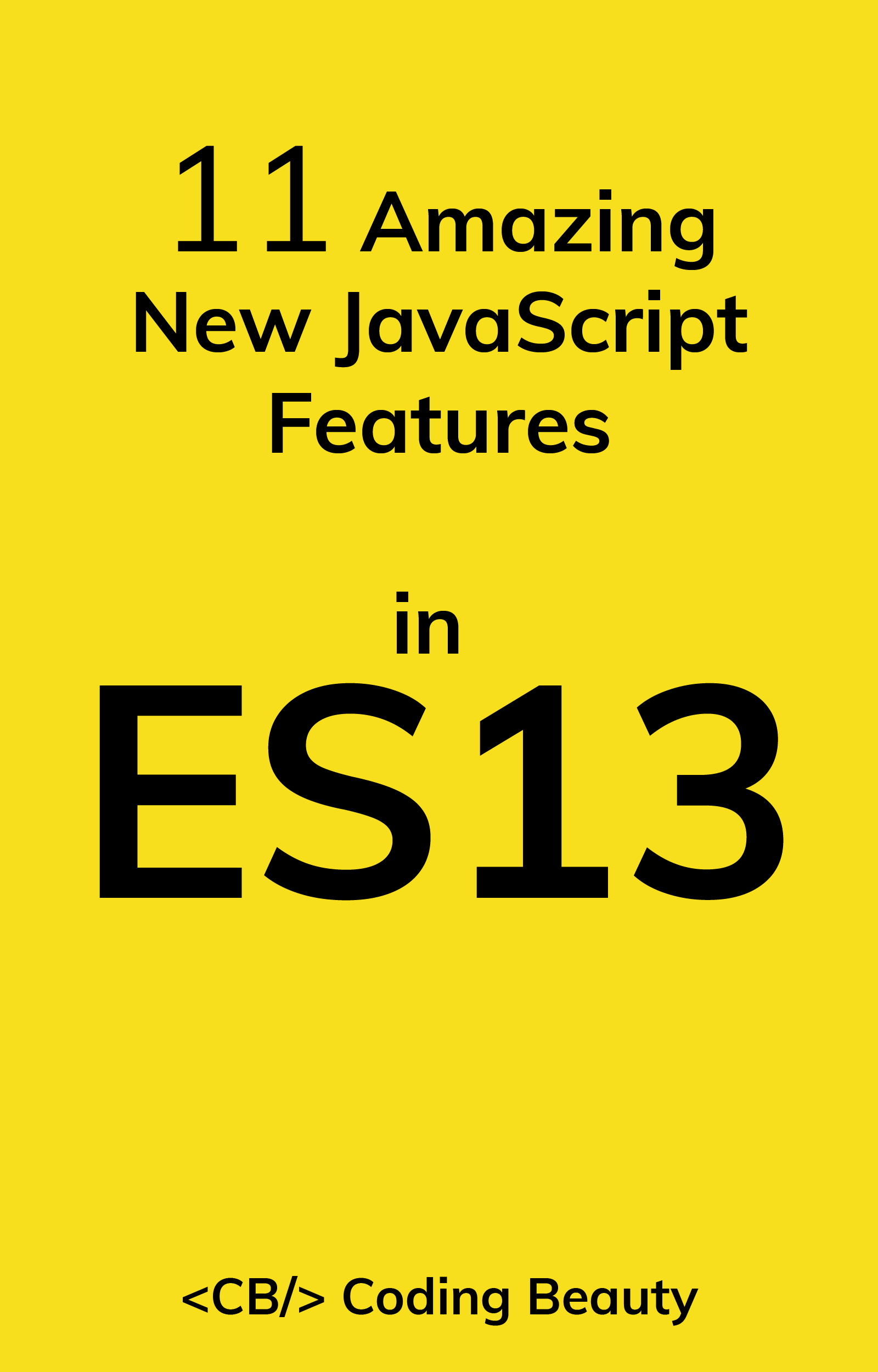