Let’s look at some ways to quickly get the length of an object in JavaScript.
1. The Object.keys() Method
To get the length of an object, we can pass the object to the static Object
keys
() method, and access the length
property of the resulting array. For example:
const obj = {
color: 'red',
topSpeed: 120,
age: 2,
};
const objectLength = Object.keys(obj).length;
console.log(objectLength); // 3
Note: Object.keys()
only returns the enumerable properties found directly on the object. If you want to include non-enumerable properties in the count, use Object.getOwnPropertyNames()
instead:
const obj = {
color: 'red',
topSpeed: 120,
age: 2,
};
Object.defineProperty(obj, 'acceleration', {
enumerable: false,
value: 5,
});
console.log(Object.keys(obj).length); // 3
console.log(Object.getOwnPropertyNames(obj).length); // 4
Note: Object.keys()
and Object.getOwnPropertyNames()
don’t work for symbolic properties. To count symbolic properties, use Object.getOwnPropertySymbols()
:
const obj = {
color: 'red',
speed: 120,
age: 2,
[Symbol('acceleration')]: 5,
[Symbol('weight')]: 1000,
};
console.log(Object.keys(obj).length); // 3
console.log(Object.getOwnPropertyNames(obj).length); // 3
console.log(Object.getOwnPropertySymbols(obj).length); // 2
2. for..in Loop and hasOwnProperty()
Another method to get the length of an object is to use the JavaScript for...in
loop to iterate over the properties of the object and increment a variable in each iteration. The variable will contain the object length after the loop.
const obj = {
color: 'red',
topSpeed: 120,
age: 2,
};
let objectLength = 0;
for (let key in obj) {
if (obj.hasOwnProperty(key)) objectLength++;
}
console.log(objectLength); // 3
Because the for...in
loop also iterates over the inherited properties of the object, we use the hasOwnProperty()
method to ensure that the property exists directly on the object before incrementing the variable.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
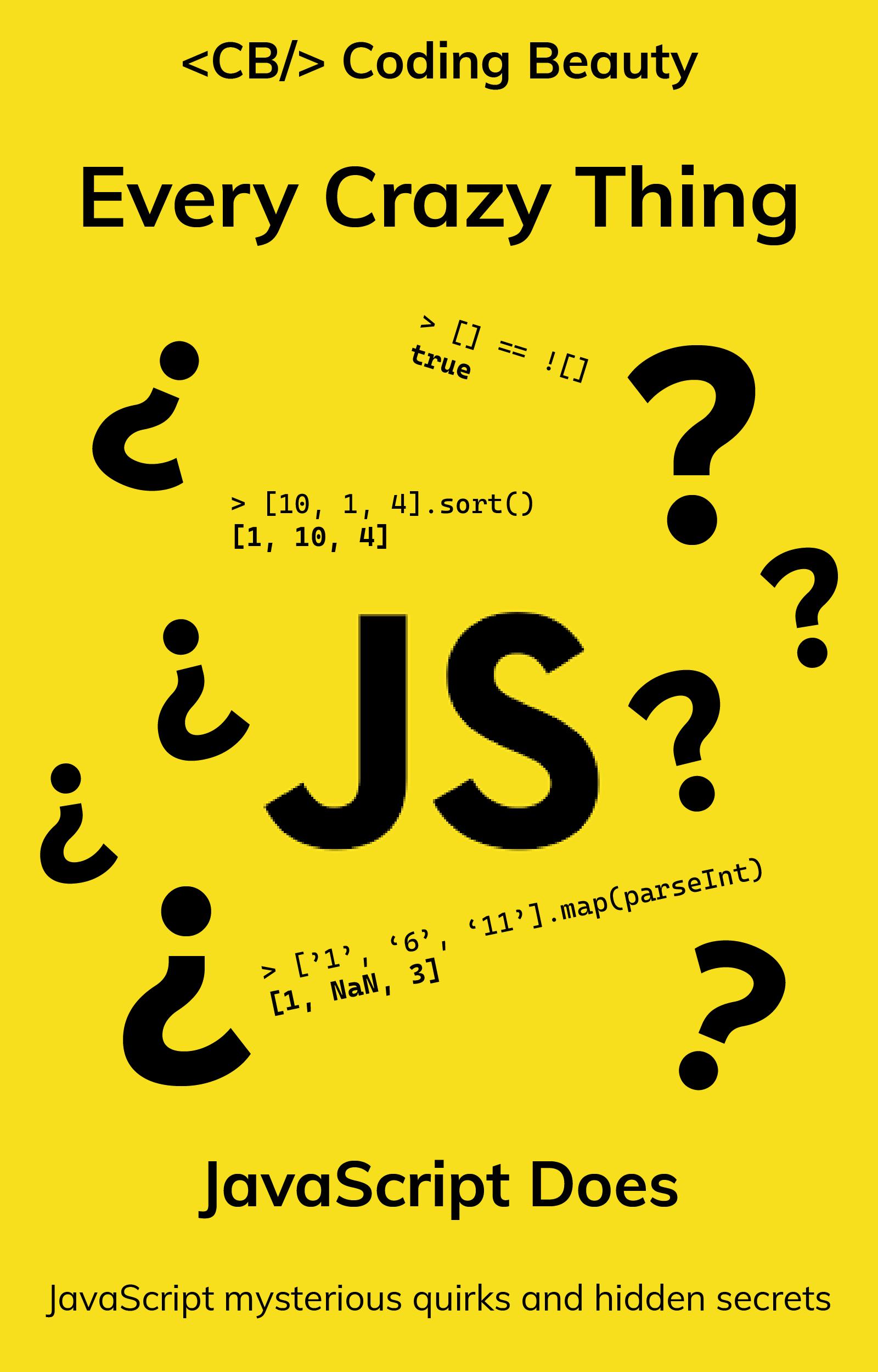