Get asymmetric difference between two arrays
To get the difference between two arrays in JavaScript, use the filter()
and include()
array methods, like this:
function getDifference(arrA, arrB) {
return arrA.filter((element) => !arrB.includes(element));
}
const arr1 = [1, 2, 3, 4];
const arr2 = [2, 4];
console.log(getDifference(arr1, arr2)); // [1, 3]
Array filter()
runs a callback on every element of an array and returns an array of elements that the callback returns true
for:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = numbers.filter(
(number) => number % 2 === 0
);
console.log(evenNumbers); // [2, 4, 6, 8, 10]
The Array includes()
method returns true
if the Array
contains a particular element and returns false
if it doesn’t:
const arr = ['a', 'b', 'c'];
console.log(arr.includes('b')); // true
If we wanted to find the difference between two Sets, we’d have used Set has()
in place of Array includes()
:
function getDifference(setA, setB) {
return new Set(
[...setA].filter(element => !setB.has(element))
);
}
const set1 = new Set([1, 2, 3, 4]);
const set2 = new Set([2, 4]);
console.log(getDifference(set1, set2)); // {1, 3}
Get symmetric difference between two arrays
The method above only gives the methods in the second array that aren’t in the first:
function getDifference(arrA, arrB) {
return arrA.filter((element) => !arrB.includes(element));
}
const arr1 = [1, 3];
const arr2 = [1, 3, 5, 7];
console.log(getDifference(arr1, arr2)); // []
You want this sometimes, especially if arr2
is meant to be arr1
‘s subset.
But other times you may want to find the symmetric difference between the arrays; regardless of which one comes first.
To do that, we simply merge the results of two getDifference()
calls, each with the order of the arrays reversed:
function getDifference(arrA, arrB) {
return arrA.filter((element) => !arrB.includes(element));
}
function getSymmetricDifference(arrA, arrB) {
return [
...getDifference(arrA, arrB),
...getDifference(arrB, arrA),
];
}
const arr1 = [1, 3];
const arr2 = [1, 3, 5, 7];
console.log(getSymmetricDifference(arr1, arr2)); // [5, 7]
console.log(getSymmetricDifference(arr2, arr1)); // [5, 7]
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
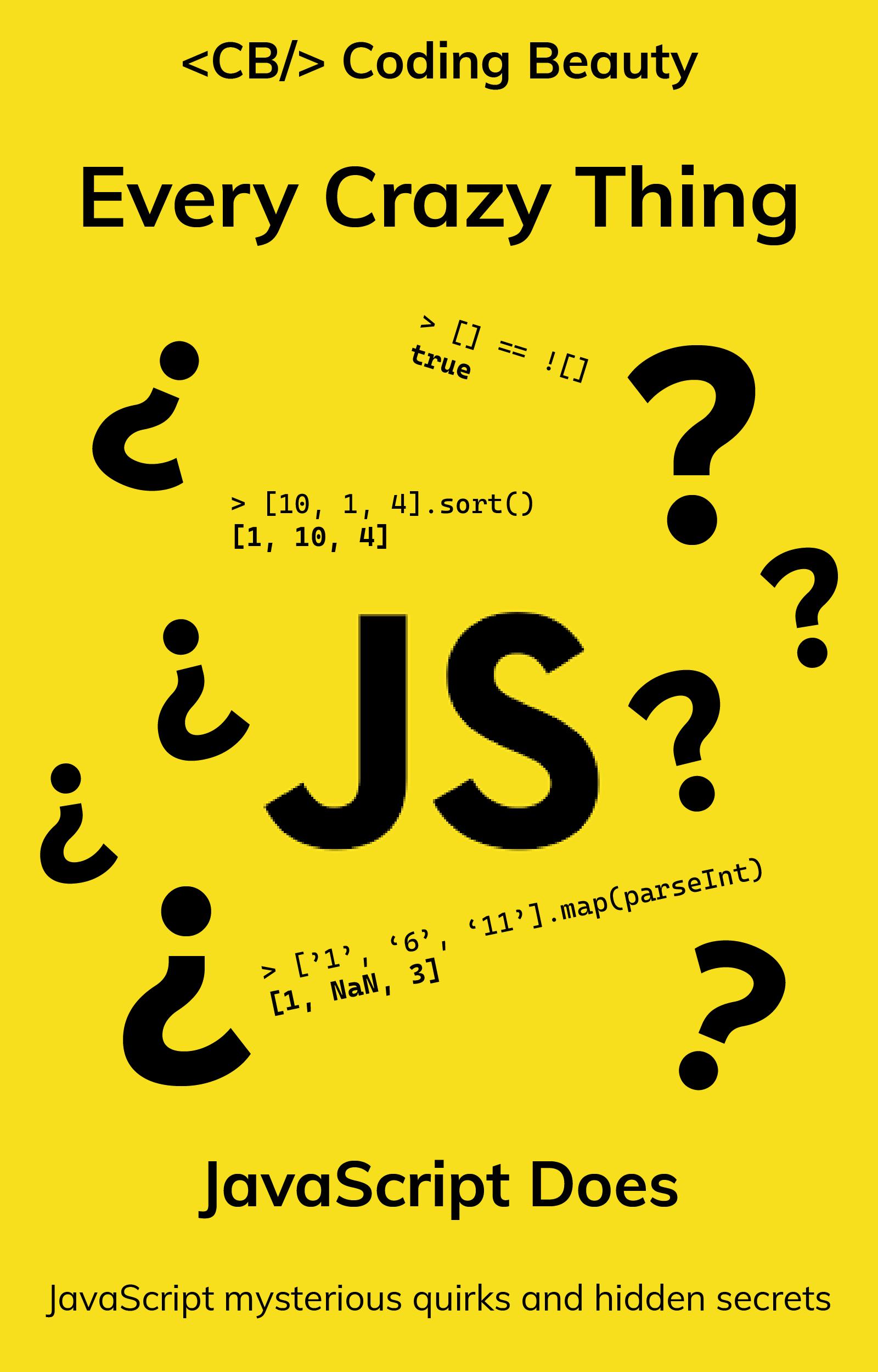