To convert JSON to a Map
in JavaScript:
- Parse the JSON string to an object with the
JSON.parse()
method. - Call
Object.entries()
with this object as an argument. - Pass the result of
Object.entries()
to theMap()
constructor.
For example:
const json =
'{"user1":"John","user2":"Kate","user3":"Peter"}';
const map = new Map(Object.entries(JSON.parse(json)));
// Map(3) { 'user1' => 'John', 'user2' => 'Kate', 'user3' => 'Peter' }
console.log(map);
We first convert the string to an object and then to an array, because we can’t parse a JSON string to a Map
directly. The Object.entries()
method takes an object and returns a list of key-value pairs that correspond to the key and value of each property of the object:
const obj = {
user1: 'John',
user2: 'Kate',
user3: 'Peter',
};
const arr = Object.entries(obj);
// [ [ 'user1', 'John' ], [ 'user2', 'Kate' ], [ 'user3', 'Peter' ] ]
console.log(arr);
The Map()
constructor can take an iterable of key-value pairs to create the Map
elements, so we pass the result of the Object.entries()
directly to it.
Convert Map to JSON
To convert the Map
back to a JSON string, call the Object.fromEntries()
method with the Map as an argument, and pass the result to the JSON.stringify()
method:
const json =
'{"user1":"John","user2":"Kate","user3":"Peter"}';
const map = new Map(Object.entries(JSON.parse(json)));
const jsonFromMap = JSON.stringify(Object.fromEntries(map));
// {"user1":"John","user2":"Kate","user3":"Peter"}
console.log(jsonFromMap);
We first transform the Map
with Object.fromEntries()
, because we can’t serialize a Map
to a JSON string directly. The Object.fromEntries()
method transforms any list of key-value pairs into an object:
const map = new Map([
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
]);
const obj = Object.fromEntries(map);
// { user1: 'John', user2: 'Kate', user3: 'Peter' }
console.log(obj);
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
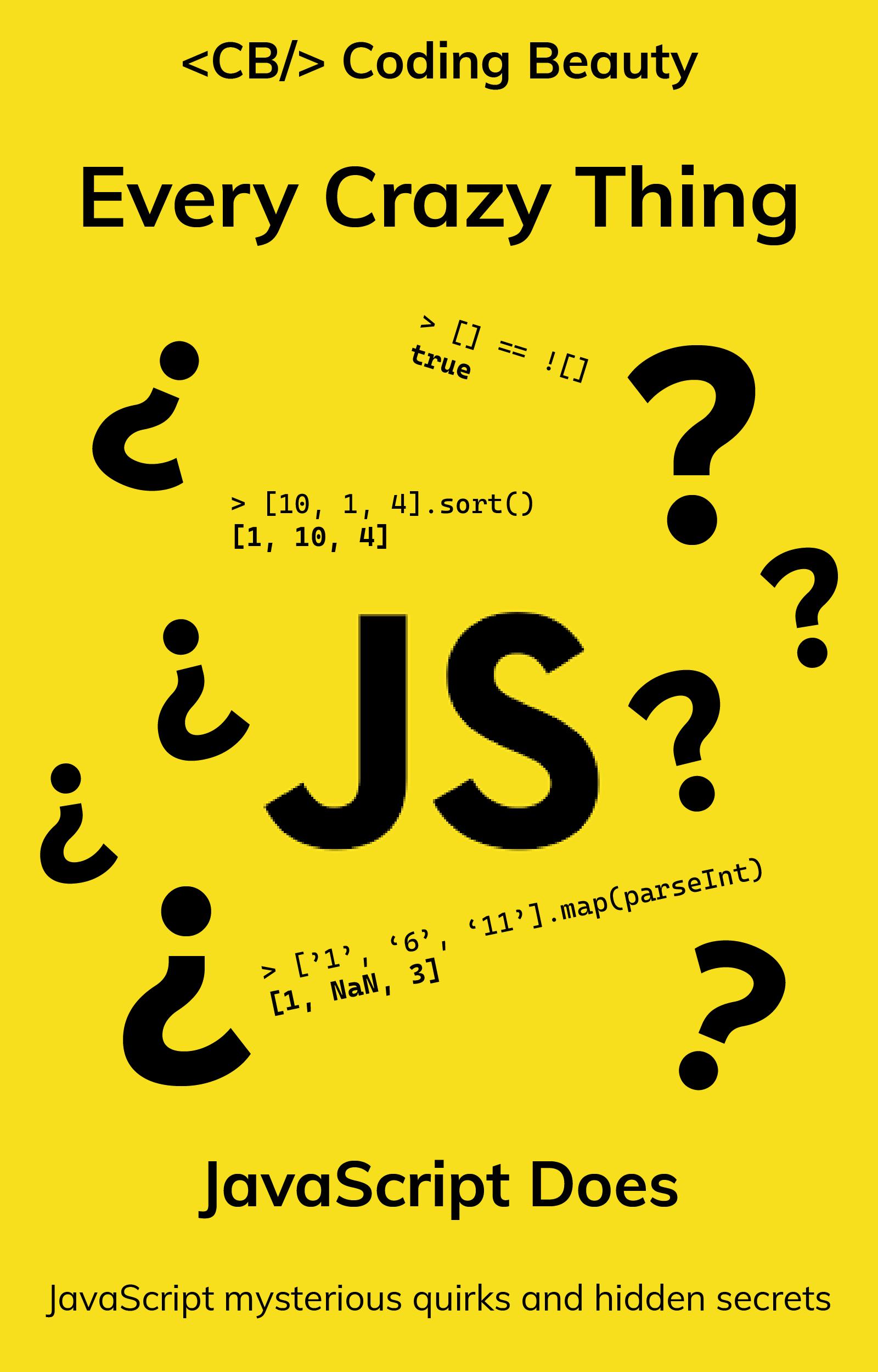