We can use the csv-to-xml
library available on NPM to quickly convert any CSV string to XML in JavaScript.
import csvToXml from 'csv-to-xml';
const csv = `color,maxSpeed,age
red,120,2
blue,100,3
green,130,2`;
const xml = csvToXml(csv);
console.log(xml);
This code will produce the following output:
<row>
<color>red</color>
<maxSpeed>120</maxSpeed>
<age>2</age>
</row>
<row>
<color>blue</color>
<maxSpeed>100</maxSpeed>
<age>3</age>
</row>
<row>
<color>green</color>
<maxSpeed>130</maxSpeed>
<age>2</age>
</row>
The default export function, csvToXml()
, takes CSV data in a string and returns its equivalent XML representation.
csv-to-xml
is an ES module that can work both in Node.js and browser environments.
Install csv-to-xml
Before using csv-to-xml
, you’ll need to install your project. You can do this with the NPM or Yarn CLI.
npm i csv-to-xml
# Yarn
yarn add csv-to-xml
After installation, you’ll be able to import it into a JavaScript module, like this:
import csvToXml from 'csv-to-xml';
csvToXml()
function
The primary function of this package is the csvToXml()
function. It takes a string containing CSV data, converts it to XML, and returns the result as a string.
csvToXml()
has two parameters:
csvString
: The string containing the CSV data.options
: An object with option properties that control how the CSV is converted to the XML.
const xml = csvToXml(csv, { header: false, quote: 'single' });
Customize conversion of CSV to XML
The option
parameter of the csvToXml()
function lets you specify options to customize the CSV-to-XML conversion process.
rowName
option
One such option is rowName
, which lets you set the tag name used for the XML elements that each represent a row.
import csvToXml from 'csv-to-xml';
const csv = `color,maxSpeed,age
red,120,2
blue,100,3
green,130,2`;
const xml = csvToXml(csv, { rowName });
console.log(xml);
<car>
<color>red</color>
<maxSpeed>120</maxSpeed>
<age>2</age>
</car>
<car>
<color>blue</color>
<maxSpeed>100</maxSpeed>
<age>3</age>
</car>
<car>
<color>green</color>
<maxSpeed>130</maxSpeed>
<age>2</age>
</car>
Now each XML row element is named car
instead of the default row
.
headerList
option
There’s also headerList
, an option used to set the CSV headers used for the conversion.
import csvToXml from 'csv-to-xml';
const csv = `First Name, Last Name, Location
Alexander,Hill,Los Angeles
Brandon,Evans,Paris
Mike,Baker,London`;
const xml = csvToXml(csv, {
rowName: 'person',
headerList: ['firstName', 'lastName', 'location'],
});
console.log(xml);
<person>
<firstName>Alexander</firstName>
<lastName>Hill</lastName>
<location>Los Angeles</location>
</person>
<person>
<firstName>Brandon</firstName>
<lastName>Evans</lastName>
<location>Paris</location>
</person>
<person>
<firstName>Mike</firstName>
<lastName>Baker</lastName>
<location>London</location>
</person>
header
option
headerList
is especially useful when the CSV data has no header row, and you see the header
option to false
to indicate this to the converter.
import csvToXml from 'csv-to-xml';
const csv = `Alexander,Hill,Los Angeles
Brandon,Evans,Paris
Mike,Baker,London`;
const xml = csvToXml(csv, {
rowName: 'user',
headerList: ['fName', 'lName', 'city'],
header: false,
});
console.log(xml);
<user>
<fName>Alexander</fName>
<lName>Hill</lName>
<city>Los Angeles</city>
</user>
<user>
<fName>Brandon</fName>
<lName>Evans</lName>
<city>Paris</city>
</user>
<user>
<fName>Mike</fName>
<lName>Baker</lName>
<city>London</city>
</user>
If header
is false
, and header names are not set with headerList
, a default column name will be used. The name takes the format col{n}
where {n}
is the position of the column within its row.
import csvToXml from 'csv-to-xml';
const csv = `Alexander,Hill,Los Angeles
Brandon,Evans,Paris
Mike,Baker,London`;
const xml = csvToXml(csv, {
header: false,
});
console.log(xml);
<row>
<col1>Alexander</col1>
<col2>Hill</col2>
<col3>Los Angeles</col3>
</row>
<row>
<col1>Brandon</col1>
<col2>Evans</col2>
<col3>Paris</col3>
</row>
<row>
<col1>Mike</col1>
<col2>Baker</col2>
<col3>London</col3>
</row>
quote
option
Another important option is quote
, which lets you group data containing a comma (or some other separator) as a single column.
For example:
import csvToXml from 'csv-to-xml';
const csv = `"Hill, A.",Los Angeles
"Evans, B.",Paris
"Baker, M.",London`;
const xml = csvToXml(csv, {
header: false,
headerList: ['name', 'location'],
quote: 'double', // Ignore comma in double quotes
});
console.log(xml);
<row>
<name>Hill, A.</name>
<location>Los Angeles</location>
</row>
<row>
<name>Evans, B.</name>
<location>Paris</location>
</row>
<row>
<name>Baker, M.</name>
<location>London</location>
</row>
indentation
option
With the indentation
option, we can control how many spaces are used to indent child XML elements in the output. By default it’s 4
, but we can set it to another numeric value, or a string like '\t'
.
import csvToXml from 'csv-to-xml';
const csv = `Alexander,Hill,Los Angeles
Brandon,Evans,Paris
Mike,Baker,London`;
const xml = csvToXml(csv, {
header: false,
indentation: 2,
});
console.log(xml);
<row>
<col1>Alexander</col1>
<col2>Hill</col2>
<col3>Los Angeles</col3>
</row>
<row>
<col1>Brandon</col1>
<col2>Evans</col2>
<col3>Paris</col3>
</row>
<row>
<col1>Mike</col1>
<col2>Baker</col2>
<col3>London</col3>
</row>
separator
option
Sometimes a character other than a comma is used to separate the columns. The separator
option helps indicate this to the converter:
import csvToXml from 'csv-to-xml';
const csv = `color;maxSpeed;age
red;120;2
blue;100;3
green;130;2`;
const xml = csvToXml(csv, { separator: ';' });
console.log(xml);
<row>
<color>red</color>
<maxSpeed>120</maxSpeed>
<age>2</age>
</row>
<row>
<color>blue</color>
<maxSpeed>100</maxSpeed>
<age>3</age>
</row>
<row>
<color>green</color>
<maxSpeed>130</maxSpeed>
<age>2</age>
</row>
Find a full list of options in the csv-to-xml
homepage.
Native conversion of CSV to XML
It’s also possible to convert CSV data to XML without using any third-party libraries. Here’s how we can do it:
const csv = `color,maxSpeed,age
red,120,2
blue,100,3
green,130,2`;
const xml = csvToXml(csv);
console.log(xml);
function csvToXml(csvString) {
const csvRows = csvString.split('\n').map((row) => row.trim());
// e.g., 'maxSpeed,color,age' -> ['maxSpeed', 'color', 'age']
const headers = csvRows[0].split(',');
let xml = '';
// loop through each CSV row, csvRows[i] contains the row string
for (let i = 1; i < csvRows.length; i++) {
// e.g., 'blue,100,3' -> ['maxSpeed', 'color', 'age']
const details = csvRows[i].split(',');
xml += `<row>\n`;
for (let j = 0; j < headers.length; j++) {
// e.g., <color>blue</color>
xml += `<${headers[j]}>${details[j]}</${headers[j]}>\n`;
}
xml += `</row>\n`;
}
return xml;
}
<row>
<color>red</color>
<maxSpeed>120</maxSpeed>
<age>2</age>
</row>
<row>
<color>blue</color>
<maxSpeed>100</maxSpeed>
<age>3</age>
</row>
<row>
<color>green</color>
<maxSpeed>130</maxSpeed>
<age>2</age>
</row>
This csvToXml()
function is certainly not as versatile as the one from the csv-to-xml
library, but as shown in the example above, it works for simple, straightforward CSV data.
You can modify it to allow for more complex and varying CSV data.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
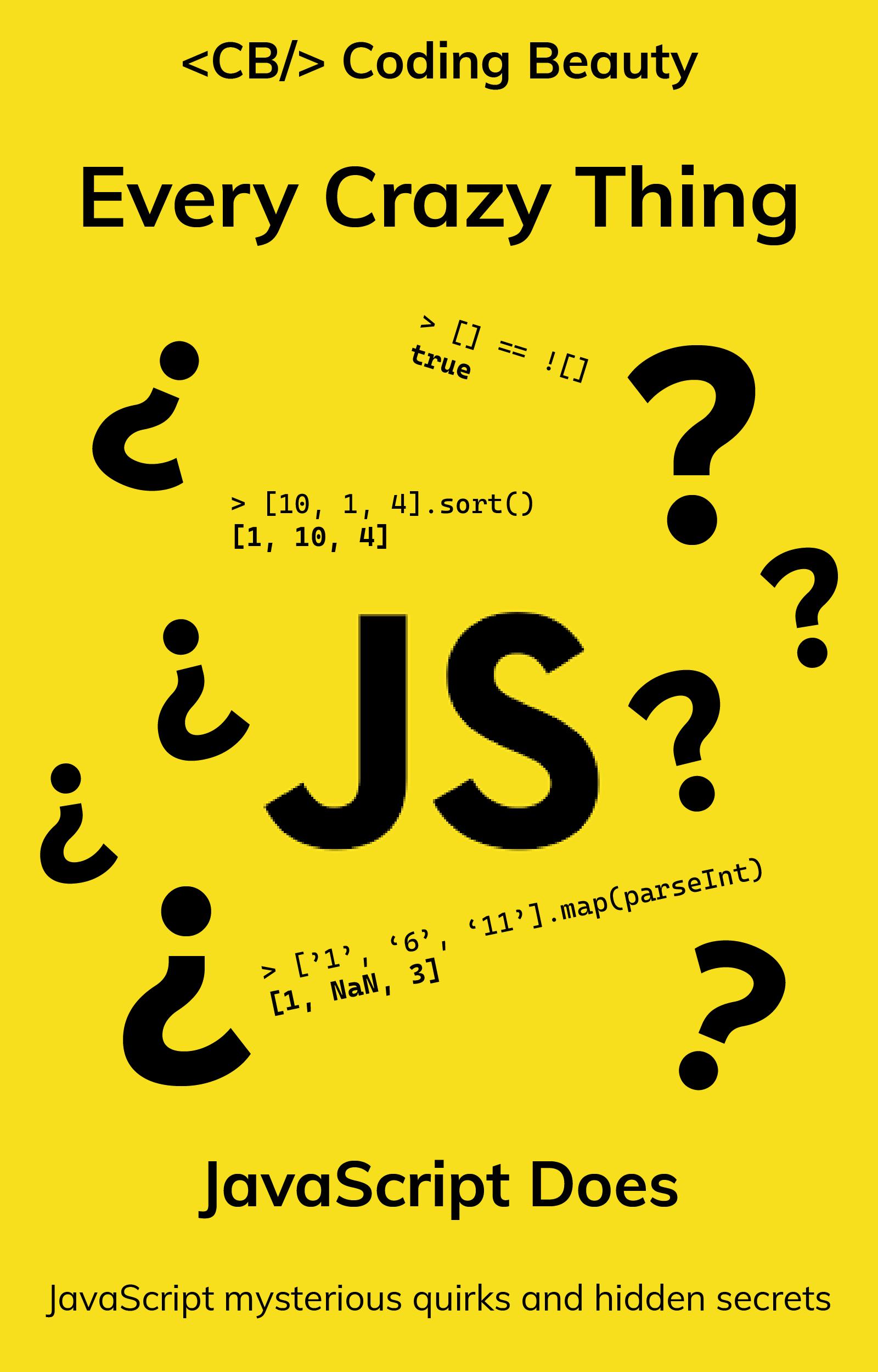