The “Cannot read property ‘toString’ of undefined” error occurs in JavaScript when you call the toString()
on a variable that unexpectedly has a value of undefined
.

To fix it, try one of the following:
- Add an
undefined
check on the variable. - Ensure the variable is initialized.
- Ensure you’re not trying to access the variable from a non-existent index in the array.
1. Add undefined
check on variable
a. Use if
statement
We can use an if
statement to check if the variable is truthy before calling the toString()
method.
const obj = undefined;
let result = undefined;
if (obj) {
result = obj.toString();
}
console.log(result); // undefined
b. Use optional chaining
We also use the optional chaining operator ?.
to return undefine
d and prevent the method access if the variable is null
or undefined
.
const obj = undefined;
const result = obj?.toString();
console.log(result);
We can use the ?.
operator for as many levels as possible.
This:
if (a?.b?.c?.d?.e) {
console.log('property "e" is not undefined');
}
is equivalent to the following:
if (a && a.b && a.b.c && a.b.c.d && a.b.c.d.e) {
console.log('property "e" is not undefined');
}
c. Call toString()
on fallback value
We can use the nullish coalescing operator (??
) to provide a fallback value to call toString()
on:
const num = undefined;
const numStr = (num ?? 10).toString();
console.log(numStr); // 10
const date = undefined;
const dateStr = (date ?? new Date()).toString();
console.log(dateStr); // Fri Jan 13 2023 20:07:10
The null coalescing operator (??
) returns the value to its left if it is not null
or undefined
. If it is, then ??
returns the value to its right.
console.log(5 ?? 10); // 5
console.log(undefined ?? 10); // 10
The logical OR operator (||
) can also do the same:
console.log(5 || 10); // 5
console.log(undefined || 10); // 10
d. Use a fallback result instead of calling replace()
We can combine the optional chaining operator (?.
) and the nullish coalescing operator (??
) to provide a fallback value to use as the result, instead of performing the replacement.
const num = undefined;
const numStr = num?.toString() ?? 'no number';
console.log(numStr); // 10
const date = undefined;
const dateStr = date?.toString() ?? 'no date';
console.log(dateStr); // Fri Jan 13 2023 20:07:10
2. Ensure variable is initialized
The solutions above are handy when we don’t know beforehand if the variable will be undefined
or not. But there are situations where the “Cannot read property ‘toString’ of undefined” error is caused by a coding error that led to the variable being undefined
.
It could be that you forgot to initialize the variable:
let date; // possibly missing initialized to new Date();
// code...
// ❌ Cannot read property 'toString' of undefined
console.log(date.toString());
Because an uninitialized variable has a default value of undefined
in JavaScript, accessing a property/method causes the error to be thrown.
The obvious fix for the error, in this case, is to assign the variable to a defined value.
let date = new Date(); // possibly missing initialized to new Date();
// ✅ toString() called, no error thrown
// Fri Jan 13 2023 20:44:22
console.log(date.toString());
3. Ensure variable is accessed from existing array index
Another common mistake that causes this error is trying to access an element at an index that doesn’t exist in the array.
For example:
const dates = [new Date('2020-05-17'), new Date('2021-08-11')];
const dateStrs = [];
// ❌ Cannot read properties of undefined (reading 'toString')
for (let i = 0; i <= dates.length; i++) {
dateStrs.push(dates[i].toString());
}
console.log(dateStrs);
We get the error in the example, because the for
loop’s condition makes it perform an iteration where i
is equal to dates.length
. As the last index if dates.length - 1
, accessing index dates.length
will result in undefined
.
The fix in this particular case is to change the <=
to <
, to make the loop stop after the iteration where i
is the last index.
const dates = [new Date('2020-05-17'), new Date('2021-08-11')];
const dateStrs = [];
// ✅ loop executes successfully - no error
for (let i = 0; i < dates.length; i++) {
dateStrs.push(dates[i].toString());
}
console.log(dateStrs);
// ['Sun May 17 2020 00:00:00...', 'Wed Aug 11 2021 00:00:00...'];
If you’re not sure that the index exists in the array, you can use the ?.
operator like we did previously.
const dates = [new Date('2020-05-17'), new Date('2021-08-11')];
// No error
console.log(dates[50]?.toString()); // undefined
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
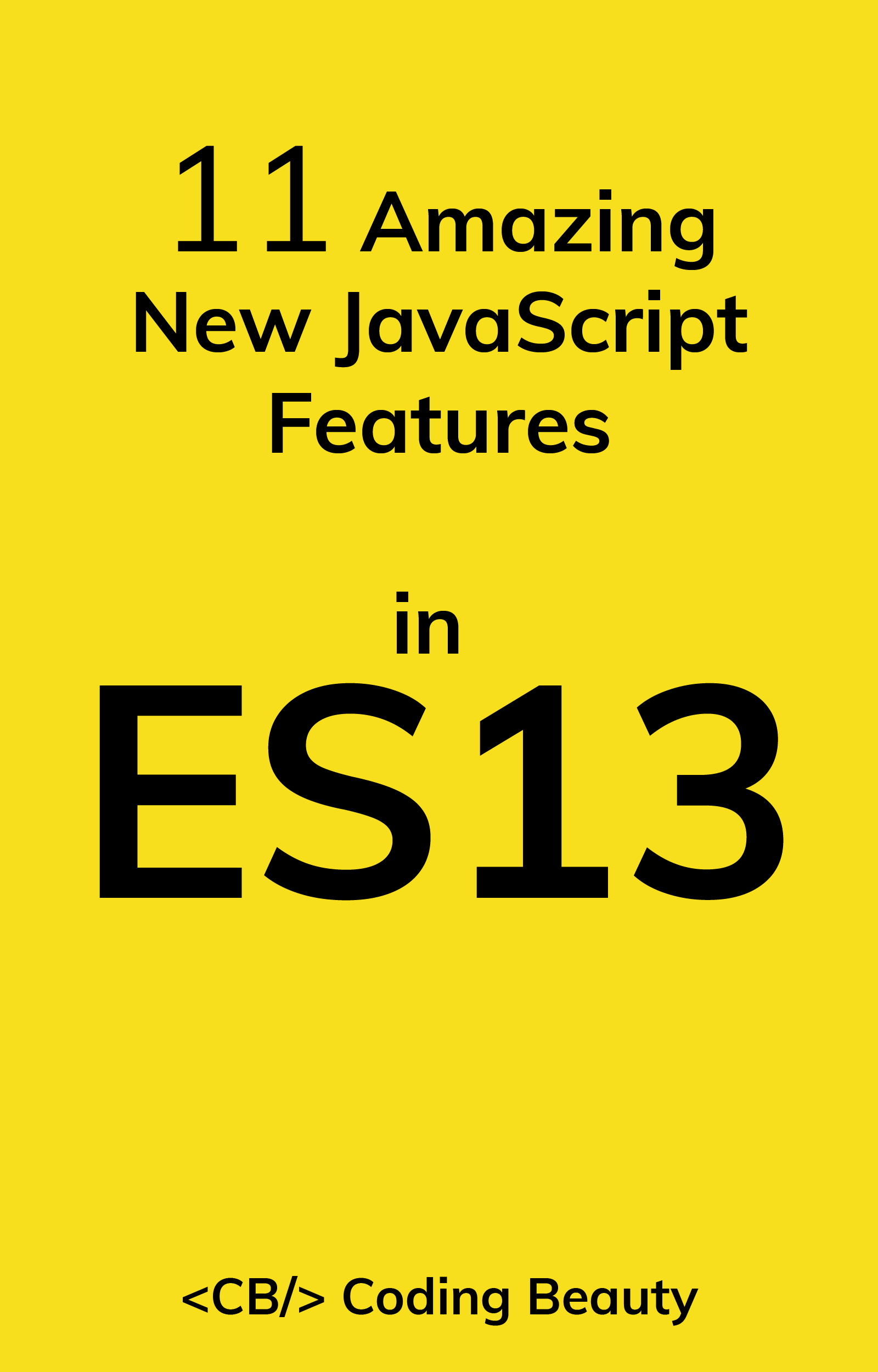