In this article, we’re going to learn how to easily add number of hours to a Date
object in JavaScript.
1. Date
setHours()
and getHours()
methods
To add hours to a Date
in JavaScript:
- Call the
getHours()
on theDate
to get the hours. - Add the hours.
- Pass the sum to the
setHours()
method.
For example:
function addHours(date, hours) {
date.setHours(date.getHours() + hours);
return date;
}
const date = new Date('2022-05-15T12:00:00.000Z');
const newDate = addHours(date, 5);
console.log(newDate); // 2022-05-15T17:00:00.000Z
The Date getHours()
method returns a number between 0
and 23
that represents the hours of a particular date.
The Date setHours()
method sets the hours of a date to a specified number.
If the hours added would increase the day, month, or year of the Date
, setHours()
will automatically update the date information to reflect this.
// 11 PM on June 17, 2022
const date = new Date('2022-06-17T23:00:00.000Z');
date.setHours(date.getHours() + 4);
// 3 AM on June 18, 2022 (next day)
console.log(date); // 2022-06-18T03:00:00.000Z
In this example, increasing the hours of the date by 4 moves the day forward to June 18, 2022.
Avoid side effects
The setHours()
method mutates the Date
object it is called on. This introduces a side effect into our addHours()
function. To make a pure function, create a copy of the Date
and call setHours()
on this copy, instead of the original. For example:
function addHours(date, hours) {
// π Make copy with "Date" constructor.
const dateCopy = new Date(date);
dateCopy.setHours(dateCopy.getHours() + hours);
return dateCopy;
}
const date = new Date('2022-05-15T12:00:00.000Z');
const newDate = addHours(date, 2);
console.log(newDate); // 2022-05-15T14:00:00.000Z
// π Original not modified
console.log(date); // 2022-05-15T12:00:00.000Z
Tip: Functions that donβt modify external state (i.e., pure functions) tend to be more predictable and easier to reason about, as they always give the same output for a particular input. This makes it a good practice to limit the number of side effects in your code.
2. date-fns addHours()
function
Alternatively, you can use the addHours()
function from the date-fns NPM package to quickly add hours to a Date
. It works like our pure addHours()
function.
import { addHours } from 'date-fns';
const date = new Date('2022-05-15T12:00:00.000Z');
const newDate = addHours(date, 4);
console.log(newDate); // 2022-05-15T16:00:00.000Z
// Original not modified
console.log(date); // 2022-05-15T12:00:00.000Z
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
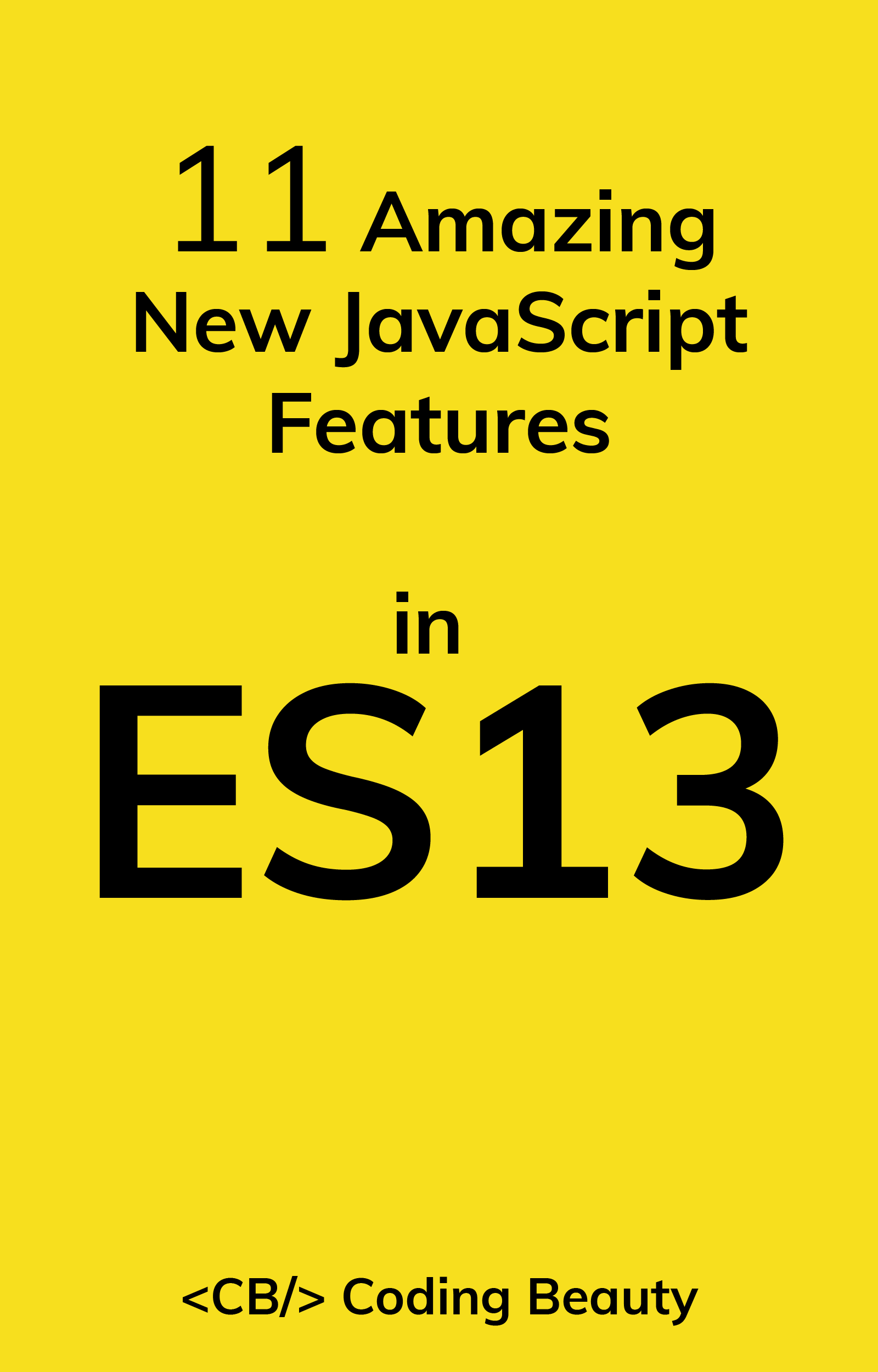