To add a class to multiple elements in JavaScript:
- Get a list of all the elements with a method like
document.querySelectorAll()
. - Iterate over the list with
forEach()
. - For each element, call
classList.add(class)
to add the class to each element.
i.e.:
const elements = document.querySelectorAll('.class');
elements.forEach((element) => {
element.classList.add('class');
});
For example:
<p class="bold text">Coding</p>
<p class="bold text">Beauty</p>
<p class="bold text">Dev</p>
<button id="add">Add class</button>
.bold {
font-weight: bold;
}
.big {
font-size: 1.2em;
}
const elements = document.querySelectorAll('.text');
elements.forEach((element) => {
element.classList.add('big');
});
This will be the HTML after the button is clicked.
<p class="big bold text">Coding</p>
<p class="big bold text">Beauty</p>
<p class="big bold text">Dev</p>
<button id="add">Add class</button>
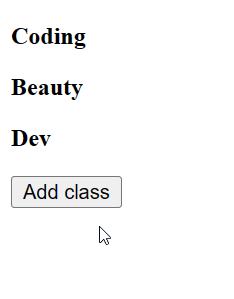
big
class to the p
elements when the button is clicked.We use the document.querySelectorAll()
method to select all DOM elements to which we want to add the class.
We loop through the elements in the list object with its forEach()
method. This forEach()
method works much like Array
forEach()
.
document.getElementsByClassName()
method
We can use the document.getElementsByClassName()
method in place of document.querySelectorAll()
when the selector is a class selector. For getElementsByClassName()
, we pass the class name without the .
(dot), and we use Array.from()
to convert the result to an array before the iteration with forEach()
.
const elements = Array.from(document.getElementsByClassName('text'));
elements.forEach((element) => {
element.classList.add('big');
});
Note: Unlike the querySelectorAll()
method, getElementByClassName()
returns a live list of HTML Elements; changes in the DOM will reflect in the array as the changes occur. If an element in the array no longer qualifies for the selector specified in getElementsByClassName()
, it will be removed automatically. This means that one iteration of the list could be different from another, even without any direct modifications to the list.
classList.add()
method
We use the classList.add()
method to add a class to each of the elements.
You can add multiple classes by passing more arguments to add()
.
const elements = document.querySelectorAll('.text');
elements.forEach((element) => {
element.classList.add('big', 'blue');
});
If any of the classes passed to add()
already exists on the element, add()
will ignore this, instead of throwing an error.
Add class to elements with different selectors
Sometimes there is no common selector between the elements that you want to add the class to. For such a case, you can pass multiple comma-separated selectors to the querySelectorAll()
method.
const elements = document.querySelectorAll('.class-1, #id-1, tag-1');
elements.forEach((element) => {
element.classList.add('class');
});
For example:
<p class="bold text">Coding</p>
<p class="bold text">Beauty</p>
<p class="bold text">Dev</p>
<div id="box-1">English</div>
<div>Spanish</div>
<div id="box-2">French</div>
<br />
<button id="add">Add class</button>
.bold {
font-weight: bold;
}
.italic {
font-style: italic;
}
.underline {
text-decoration: underline;
}
const elements = document.querySelectorAll('.text, #box-1, #box-2');
const addButton = document.getElementById('add');
addButton.addEventListener('click', () => {
elements.forEach((element) => {
element.classList.add('italic', 'underline');
});
});
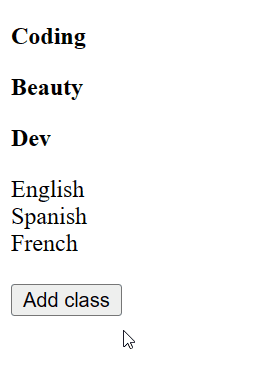
Remove class from multiple elements
Just as the classList.add()
adds one or more classes to an element, the classList.remove()
removes one or more classes from an element.
So we can use it in a forEach()
loop to a class to multiple DOM elements.
const elements = document.querySelectorAll('.text');
elements.forEach((element) => {
element.classList.remove('big', 'bold');
});
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.

This was very helpful. I couldn’t get my code to work for querySelectorAll but your example led me on the right path. Thanks!
You’re welcome 👍